
Be patient..... we are fetching your source code.
Objectives
The main objective of this blog post is to explain how to implement Ads in Unity. This blog post is very useful to developers who don’t know anything about Ad or Ad implementation in Unity. Here we go through the idea of how to implement Ad in unity by using ChartBoost SDK.
Step 1 ChartBoost
Here we are going to focus on how to implement it using ChartBoost. This SDK basically provides interstitial ads, more apps, rewarded video and in-play ad. Advertisers can implement any of them according to their requirement.
Following are the steps from which u can get brief idea about how to integrate ChartBoost SDK in Android as well as in iOS.
Step 2 Find or Create Your App ID
You can find/ create your app IDs from following given link:
Step 3 Configuation
To access the ChartBoost SDK and register your application, you have to get your app id and app signature.
- First of all, download latest available version of the SDK.
- Now, you have to import this SDK as a custom package.
ChartBoost Unity SDK can be integrate using c# or JavaScript code. Here you have to keep one thing in mind that, you have to test it only in devices, as Ads will not show in Unity editor/ xCode simulator.
After importing the SDK, Go to Chartboost >> Edit Settings.
Now paste your app id and app signature for all the platforms.
Step 4 Integrate in iOS
Chartboost SDK for iOS supports only in XCode version 4.4.1 or later. You have to include following two libraries as it is required.
- AdSupport
- StoreKit
You can get more idea by following image:
Step 5 Integrate in Android
Here , You have to add following required permission into your AndroidManifest.xml File by using following line of code.
<!-- Required --><uses-permissionandroid:name="android.permission.INTERNET"/>
Now, add the required Chartboost activity inside the application tag.
<application>
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<uses-permission android:name="com.android.vending.BILLING" />
<activity android:name="com.chartboost.sdk.sample.SampleActivity"
android:label="@string/app_name"
android:configChanges="keyboardHidden|orientation|screenSize" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>>
</activity>
</application>
Step 6 Integration
Initialize:
First of all, just drag and drop the Chartboost prefab in hierarchy. You can found Chartboost prefab from Assets/Chartboost/Chartboost.
You can Create Ad (Cache Ads in memory) using following line of code.
Create Interstitial (Cache in Memory):
Chartboost.cacheInterstitial(CBLocation.Default);
Create More Apps:
Chartboost.cacheMoreApps(CBLocation.Default);
Create Rewarded Video:
Chartboost. cacheRewardedVideo (CBLocation.Default);
Create In-play:
Chartboost. cacheInPlay (CBLocation.Default);
You Can Show Ad (Display on Screen) using following line of code.
Display Interstitial:
Chartboost.showInterstitial (CBLocation.Default);
Display More Apps:
Chartboost.showMoreApps(CBLocation.Default);
Display Rewarded Video:
Chartboost. showRewardedVideo (CBLocation.Default);
Display In-play:
To display in-play get reference of two game objects. One of them is for game icon and another is for text.
public GameObject inPlayIcon; // for in-play icon
public GameObject inPlayText; // for in-play text
private CBInPlay inPlayAd;
inPlayAd = Chartboost.getInPlay(CBLocation.Default);
if(inPlayAd != null) {
inPlayIcon.guiTexture.texture = inPlayAd.appIcon;
inPlayText.guiText.text = inPlayAd.appName;
inPlayAd.show();
}
Step 7 Listeners
Listeners (which is also called Delegates/Callbacks) help you to follow Ad workflow. Whenever state of the Ad will change Chartboost SDK will fore events respectively. It calls specific methods as per the callbacks. For example, if Ad is loaded successfully or not, you can identify whenever user click on Ad etc. Following line of code gives brief idea about it.
You have to register and remove all the callbacks with the use of OnEnable() and OnDisable() methods.
void OnEnable() {
// Listen to all impression-related events
Chartboost.didFailToLoadInterstitial += didFailToLoadInterstitial;
Chartboost.didDismissInterstitial += didDismissInterstitial;
Chartboost.didCloseInterstitial += didCloseInterstitial;
Chartboost.didClickInterstitial += didClickInterstitial;
Chartboost.didCacheInterstitial += didCacheInterstitial;
Chartboost.shouldDisplayInterstitial += shouldDisplayInterstitial;
Chartboost.didDisplayInterstitial += didDisplayInterstitial;
Chartboost.didFailToLoadMoreApps += didFailToLoadMoreApps;
Chartboost.didDismissMoreApps += didDismissMoreApps;
Chartboost.didCloseMoreApps += didCloseMoreApps;
Chartboost.didClickMoreApps += didClickMoreApps;
Chartboost.didCacheMoreApps += didCacheMoreApps;
Chartboost.shouldDisplayMoreApps += shouldDisplayMoreApps;
Chartboost.didDisplayMoreApps += didDisplayMoreApps;
Chartboost.didFailToRecordClick += didFailToRecordClick;
Chartboost.didFailToLoadRewardedVideo += didFailToLoadRewardedVideo;
Chartboost.didDismissRewardedVideo += didDismissRewardedVideo;
Chartboost.didCloseRewardedVideo += didCloseRewardedVideo;
Chartboost.didClickRewardedVideo += didClickRewardedVideo;
Chartboost.didCacheRewardedVideo += didCacheRewardedVideo;
Chartboost.shouldDisplayRewardedVideo += shouldDisplayRewardedVideo;
Chartboost.didCompleteRewardedVideo += didCompleteRewardedVideo;
Chartboost.didDisplayRewardedVideo += didDisplayRewardedVideo;
Chartboost.didCacheInPlay += didCacheInPlay;
Chartboost.didFailToLoadInPlay += didFailToLoadInPlay;
Chartboost.didPauseClickForConfirmation += didPauseClickForConfirmation;
Chartboost.willDisplayVideo += willDisplayVideo;
#if UNITY_IPHONE
Chartboost.didCompleteAppStoreSheetFlow += didCompleteAppStoreSheetFlow;
#endif
}
void OnDisable() {
// Remove event handlers
Chartboost.didFailToLoadInterstitial -= didFailToLoadInterstitial;
Chartboost.didDismissInterstitial -= didDismissInterstitial;
Chartboost.didCloseInterstitial -= didCloseInterstitial;
Chartboost.didClickInterstitial -= didClickInterstitial;
Chartboost.didCacheInterstitial -= didCacheInterstitial;
Chartboost.shouldDisplayInterstitial -= shouldDisplayInterstitial;
Chartboost.didDisplayInterstitial -= didDisplayInterstitial;
Chartboost.didFailToLoadMoreApps -= didFailToLoadMoreApps;
Chartboost.didDismissMoreApps -= didDismissMoreApps;
Chartboost.didCloseMoreApps -= didCloseMoreApps;
Chartboost.didClickMoreApps -= didClickMoreApps;
Chartboost.didCacheMoreApps -= didCacheMoreApps;
Chartboost.shouldDisplayMoreApps -= shouldDisplayMoreApps;
Chartboost.didDisplayMoreApps -= didDisplayMoreApps;
Chartboost.didFailToRecordClick -= didFailToRecordClick;
Chartboost.didFailToLoadRewardedVideo -= didFailToLoadRewardedVideo;
Chartboost.didDismissRewardedVideo -= didDismissRewardedVideo;
Chartboost.didCloseRewardedVideo -= didCloseRewardedVideo;
Chartboost.didClickRewardedVideo -= didClickRewardedVideo;
Chartboost.didCacheRewardedVideo -= didCacheRewardedVideo;
Chartboost.shouldDisplayRewardedVideo -= shouldDisplayRewardedVideo;
Chartboost.didCompleteRewardedVideo -= didCompleteRewardedVideo;
Chartboost.didDisplayRewardedVideo -= didDisplayRewardedVideo;
Chartboost.didCacheInPlay -= didCacheInPlay;
Chartboost.didFailToLoadInPlay -= didFailToLoadInPlay;
Chartboost.didPauseClickForConfirmation -= didPauseClickForConfirmation;
Chartboost.willDisplayVideo -= willDisplayVideo;
#if UNITY_IPHONE
Chartboost.didCompleteAppStoreSheetFlow -= didCompleteAppStoreSheetFlow;
#endif
}
Delegates:
void didFailToLoadInterstitial(CBLocation location, CBImpressionError error) {
Debug.Log(string.Format("didFailToLoadInterstitial: {0} at location {1}", error, location));
}
void didDismissInterstitial(CBLocation location) {
Debug.Log("didDismissInterstitial: " + location);
}
void didCloseInterstitial(CBLocation location) {
Debug.Log("didCloseInterstitial: " + location);
}
void didClickInterstitial(CBLocation location) {
Debug.Log("didClickInterstitial: " + location);
}
void didCacheInterstitial(CBLocation location) {
Debug.Log("didCacheInterstitial: " + location);
}
bool shouldDisplayInterstitial(CBLocation location) {
Debug.Log("shouldDisplayInterstitial: " + location);
return true;
}
void didDisplayInterstitial(CBLocation location){
Debug.Log("didDisplayInterstitial: " + location);
}
void didFailToLoadMoreApps(CBLocation location, CBImpressionError error) {
Debug.Log(string.Format("didFailToLoadMoreApps: {0} at location: {1}", error, location));
}
void didDismissMoreApps(CBLocation location) {
Debug.Log(string.Format("didDismissMoreApps at location: {0}", location));
}
void didCloseMoreApps(CBLocation location) {
Debug.Log(string.Format("didCloseMoreApps at location: {0}", location));
}
void didClickMoreApps(CBLocation location) {
Debug.Log(string.Format("didClickMoreApps at location: {0}", location));
}
void didCacheMoreApps(CBLocation location) {
Debug.Log(string.Format("didCacheMoreApps at location: {0}", location));
}
bool shouldDisplayMoreApps(CBLocation location) {
Debug.Log(string.Format("shouldDisplayMoreApps at location: {0}", location));
return true;
}
void didDisplayMoreApps(CBLocation location){
Debug.Log("didDisplayMoreApps: " + location);
}
void didFailToRecordClick(CBLocation location, CBImpressionError error) {
Debug.Log(string.Format("didFailToRecordClick: {0} at location: {1}", error, location));
}
void didFailToLoadRewardedVideo(CBLocation location, CBImpressionError error) {
Debug.Log(string.Format("didFailToLoadRewardedVideo: {0} at location {1}", error, location));
}
void didDismissRewardedVideo(CBLocation location) {
Debug.Log("didDismissRewardedVideo: " + location);
}
void didCloseRewardedVideo(CBLocation location) {
Debug.Log("didCloseRewardedVideo: " + location);
}
void didClickRewardedVideo(CBLocation location) {
Debug.Log("didClickRewardedVideo: " + location);
}
void didCacheRewardedVideo(CBLocation location) {
Debug.Log("didCacheRewardedVideo: " + location);
}
bool shouldDisplayRewardedVideo(CBLocation location) {
Debug.Log("shouldDisplayRewardedVideo: " + location);
return true;
}
void didCompleteRewardedVideo(CBLocation location, int reward) {
Debug.Log(string.Format("didCompleteRewardedVideo: reward {0} at location {1}", reward, location));
}
void didDisplayRewardedVideo(CBLocation location){
Debug.Log("didDisplayRewardedVideo: " + location);
}
void didCacheInPlay(CBLocation location) {
Debug.Log("didCacheInPlay called: "+location);
}
void didFailToLoadInPlay(CBLocation location, CBImpressionError error) {
Debug.Log(string.Format("didFailToLoadInPlay: {0} at location: {1}", error, location));
}
void didPauseClickForConfirmation() {
Debug.Log("didPauseClickForConfirmation called");
}
void willDisplayVideo(CBLocation location) {
Debug.Log("willDisplayVideo: " + location);
}
#if UNITY_IPHONE
void didCompleteAppStoreSheetFlow() {
Debug.Log("didCompleteAppStoreSheetFlow");
}
#endif
Step 8 Testing
You have to test you app in basically two ways.
- When Ad received successfully.
- When Ad cannot deliver by any of the reasons.
By Activating Test mode you can easily get through both of these scenarios. You can get more idea from following image.
Checkout other Ads Implementation in Unity:
I hope you find this blog very helpful. Let me know in comment if you have any question regarding Unity. I will help you ASAP.
Got an Idea of Game Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Game Development Company in India.
Checkout Ads Implementation in Unity Using AppLovin, AdColony, AdMob and RevMob
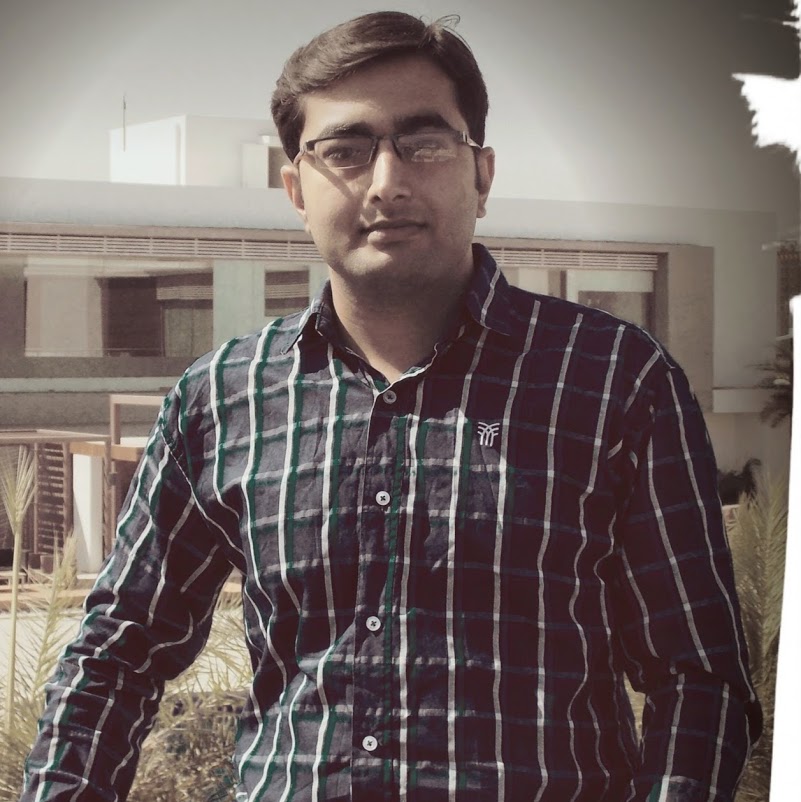
I am 2D Game developer and iOS Developer with an aspiration of learning new technology and creating a bright future in Information Technology.
Responsive Website Using Grid Framework