
Be patient..... we are fetching your source code.
Objective
Box SDK for android is the powerful API, the main objective is to provide proper guidance of Box SDK and it's implementation in your android projects.
Step 1 What is Box SDK?
Box is the Android SDK which acts as powerful content provider for your application. To get started, you can upload files directly from your device. The Content API will give full access of Box. Whether you are constructing a business app, content management software or need to present content exquisitely on web and mobile, the Box Platform can help facilitate the content and data layer of your application.
What functionality API Provides?
- Once your files are on Box you can share files easily with a link.
- You can quickly search for your files and folders.
- With the help of this API you can edit files and save back to Box.
- You can save files to your device for offline access.
- You can create online workspace by inviting teammates to your folder.
- You can exchange feedbacks with colleagues.
- Also you can see recent activities by team members on the updates tab
Following are the key feature of Box Content:
1.1 Content
Box content covers all basics, and many other functionality which includes: Folders, Files, Users, Comments, Tasks with persistent ID's for every object.
1.2 Metadata
Customizable name-value pairs you can associate with any file. This will helps you to make your Context more meaningful.
1.3 Security
This API involved great attention to permissions, which uses 256-bit AES encryption and more.
1.4 Administration
This API provides functionality like Manage users, Manage groups and other enterprise settings. Provides all the detailed reports of who is accessing, what is accessing and the log time of access. Box(V2) requires Android SDK. This project of Box(V2) is not functional without JAVA SDK To Implement this functionality in your android app you need Box Java SDK, so first you must download this SDK. There are few important steps listed below which will guide you to implement the Box(V2) API in your project. Here Android SDK depends on Box Java SDK, So you must first import Java SDK and after that import Android SDK, make Java SDK as a build dependency
Step 2 Download Box Java SDK
Download Box Java SDK which contains BoxAndroidLibraryV2 and BoxJavaLibraryV2 libraries.
Step 3 Import Projects
Now in Eclipse simply import those two projects into your workspace, then select this two projects
Step 4 Add box android SDK as an android library project
Add box android SDK as an android library project: Open properties of your project, select Android, on bottom Library section, click Add, add BoxAndroidLibraryV2.
Step 5 Authentication
Authentication Make sure you've set up your client id, client secret and (optional) redirect url correctly.
For setting up your necessary parameters:
- Go to https://developers.box.com/
- Create your account in this site or login if already created.
- After login, within your account click on Create a Box Application
1. Give unique name to your Box Application and click on Box Content.
2. Configure your Application: [Type here localhost as a default redirect_uri ]
[Type here localhost as a default redirect_uri ]
[Select check box for Android Phone and Tablet]
3. Save your Application:
[Save generated cliend_id and client_secret for further use in your application]
Step 6 How to use client_id and client_secret keys
How to Use client_id and client_secret keys in your project.
public class BoxSDKSampleApplication extends Application {
// TODO: use your own client settings
public static final String CLIENT_ID = "//add your client id";
public static final String CLIENT_SECRET = "//add your client secret";
private ArrayList<BoxAndroidClient> list = new ArrayList<BoxAndroidClient>();
public void setClient(BoxAndroidClient client) {
list.add(client);
}
/**
* Gets the BoxAndroidClient for this app.
*
* @return a singleton instance of BoxAndroidClient.
*/
public ArrayList<BoxAndroidClient> getClient() {
return list;
}
}
[Implementation of keys in BoxSDKSampleApplication class]
Step 7 OAuthActivity Activity.
Setting parameters and other settings in project.
Authentication: For authentication on click use this function, The coolest way to authenticate is to use the OAuthActivity, which is included in the SDK. Add it to your manifest to use it.
private void startAuth() {
boxModelModelList=new ArrayList<BoxModel>();
Intent intent = OAuthActivity.createOAuthActivityIntent(this,
BoxSDKSampleApplication.CLIENT_ID,
BoxSDKSampleApplication.CLIENT_SECRET, false);
startActivityForResult(intent, AUTHENTICATE_REQUEST);
}
// If you don't have a server redirect url, use this instead:
// Intent intent = createOAuthActivityIntent(context, clientId, clientSecret, false, "http://localhost");
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
switch (requestCode) {
case AUTHENTICATE_REQUEST:
if (resultCode == Activity.RESULT_CANCELED) {
if (data != null) {
String failMessage = data
.getStringExtra(OAuthActivity.ERROR_MESSAGE);
Toast.makeText(this, "Auth fail:" + failMessage,
Toast.LENGTH_LONG).show();
finish();
}
} else {
BoxAndroidOAuthData oauth = data
.getParcelableExtra(OAuthActivity.BOX_CLIENT_OAUTH);
BoxAndroidClient client = new BoxAndroidClient(
BoxSDKSampleApplication.CLIENT_ID,
BoxSDKSampleApplication.CLIENT_SECRET, null, null, null);
client.authenticate(oauth);
BoxSDKSampleApplication app = (BoxSDKSampleApplication) getApplication();
client.addOAuthRefreshListener(new OAuthRefreshListener() {
//This SDK auto refreshes OAuth access token when it expires. You will want to listen to the refresh events and update your stored token after refreshing.
@Override
public void onRefresh(IAuthData newAuthData) {
Log.d("OAuth",
"oauth refreshed, new oauth access token is:"
+ newAuthData.getAccessToken());
}
});
app.setClient(client);
onClientAuthenticated();
}
break;
}
}
Set background parameters by implementing below code:
@Override
protected ArrayList<BoxCollection> doInBackground(Null... params) {
ArrayList<BoxCollection> boxCollectionItemsList = new ArrayList<BoxCollection>();
try {
BoxPagingRequestObject requestObj = new BoxPagingRequestObject();
requestObj.getRequestExtras().addField(BoxFile.FIELD_NAME);
requestObj.getRequestExtras().addField(
BoxFile.FIELD_MODIFIED_AT);
requestObj.getRequestExtras().addField(
BoxFile.FIELD_SHARED_LINK);
requestObj.getRequestExtras().addField(BoxFile.FIELD_SIZE);
requestObj.getRequestExtras().addField(
BoxFile.FIELD_OWNED_BY);
for (int i = 0; i < getClient().size(); i++) {
boxCollectionItemsList.add(getClient().get(i)
.getFoldersManager()
.getFolderItems("0", requestObj));
}
return boxCollectionItemsList;
} catch (BoxSDKException e) {
return boxCollectionItemsList;
}
}
For more about doInBackground() method:
Step 8 AndroidManifest file
Set Parameters in the project’s Manifest file. Box FileManager to Download Files.
<activity android:name="com.box.boxandroidlibv2.activities.OAuthActivity" />
<activity android:name="BoxFileManager" >
</activity>
Set your application name same as class created in step 5
<application
android:name="yourPackageName.BoxSDKSampleApplication">
</application>
If you have got any query related to BOX SDK for Android comment them below.
Got an Idea of Android App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Android App Development Company in India.
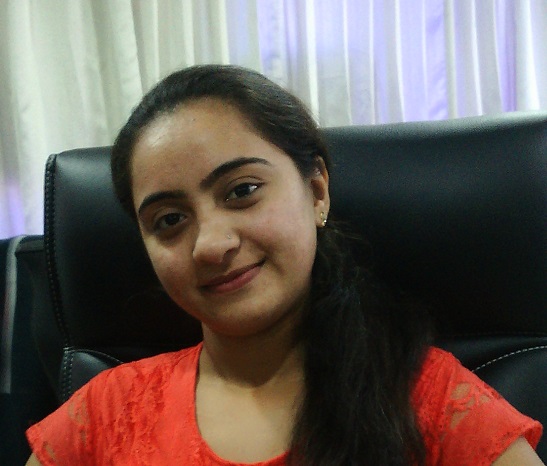
I am android developer, and do development not because to get paid or get adulation by the public, but because it is fun to program. I have been working in this field since one and half year.
How to start FTP in Android
iOS - Dropbox Integration