
Be patient..... we are fetching your source code.
Objective
Main objective of this Android action bar example is to explain the working of ActionBar in Android.
Basics of Action Bar in Android:
Action Bar is located at top of the activity. It is nothing but a view that displays Activity Title, Icon, and Actions together and supports for navigation and view switching. It is dedicated space for our application and identify and indicate user’s location in the Android application.
Step 1 Add ActionBar in Android
Following are the steps to add Action Bar in your Android app:
- A simple Action Bar displays activity name and activity icon on the left of the Action Bar.
- This simple form of action bar in our activity is used to inform users where they are in our application.
- To add this simple action bar in application, we need to set Activity Theme that enables action bar to our activity.
- Setting up theme to add action bar depends on which minimum version of android we are going to use in our application.
- If Support Version of android is 2.1 or above in your app, integrate Supports library in application.
- After integrating support library, edit all activities to extend ActionBarActivity.
- And set android theme of activity to @style/Theme.AppCompat.Light in Application manifest file
- If Support Version of android is 3.0 or above in your app.
- Then set android theme of activity to @style/Theme.Holo or one of its descendants in Application Manifest file.
- I have use support version 3.0 in following example.
Adding Action Buttons on the Action Bar:
- The Action Bar allows you to add action buttons to perform action relating to your current app context.
- Buttons which are appeared directly in the action bar are called Action buttons.
- And actions that cannot fit to the action bar are hidden in the action overflow.
- To add items, define xml resource in menu.
Following are the steps to add Action Buttons:
1.1 Create XML file actionmenu.xml
Create XML file actionmenu.xml with following content in res >> menu for this Actionbar in Android example in your project.
<menu xmlns:android="http://schemas.android.com/apk/res/android" >
<item
android:id="@+id/action_search"
android:icon="@drawable/ic_action_search"
android:showAsAction="always"
android:title="@string/action_search"/>
<!-- Settings, should always be in the overflow -->
<item
android:id="@+id/action_location_found"
android:icon="@drawable/ic_action_location_found"
android:showAsAction="always"
android:title="@string/action_location_found"/>
<item
android:id="@+id/menu_overflow"
android:icon="@drawable/ic_action_menu"
android:orderInCategory="11111"
android:showAsAction="always">
<menu>
<!-- Refresh -->
<item
android:id="@+id/action_refresh"
android:icon="@drawable/ic_action_refresh"
android:showAsAction="ifRoom"
android:title="@string/action_refresh"/>
<!-- Help -->
<item
android:id="@+id/action_help"
android:icon="@drawable/ic_action_help"
android:showAsAction="never"
android:title="@string/action_help"/>
<!-- Check updates -->
<item
android:id="@+id/action_check_updates"
android:icon="@drawable/ic_action_refresh"
android:showAsAction="never"
android:title="@string/action_check_updates"/>
</menu>
</item>
</menu>
1.2 Add Items In Menu
To add action items you specified in menu in activity, override onCreateOptionMenu() in your activity class in following way.
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.actionmenu, menu);
return true;
}
1.3 Add Item Events
Now to add Action Item Events Override onOptionsItemSelected() method as following:
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Take appropriate action for each action item click
switch (item.getItemId()) {
case R.id.action_search:
// search action
return true;
case R.id.action_location_found:
// location found
return true;
case R.id.action_refresh:
// refresh
return true;
case R.id.action_help:
// help action
return true;
case R.id.action_check_updates:
// check for updates action
return true;
default:
return super.onOptionsItemSelected(item);
}
}
getItemId() method of MenuItem is used to determine which action items is clicked.
Step 2 Manage Back Navigation.
Now in our Android Action Bar tutorial, we will add Back/ Up Navigation.
Following are the steps to enable back navigation to activity when our application involves hierarchical relationship between screens.
2.1 onOptionsItemSelected() Method
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.action_search:
// search action
return true;
case R.id.action_location_found:
// location found
Intent i = new Intent(MainActivity.this, LocationFound.class);
startActivity(i);
return true;
case R.id.action_refresh:
// refresh
return true;
case R.id.action_help:
// help action
return true;
case R.id.action_check_updates:
// check for updates action
return true;
default:
return super.onOptionsItemSelected(item);
}
}
More about onOptionsItemSelected() Method please refer the following link:
2.2 Create locationfound.xml file
Add locationfound.xml using following code in layout folder of app:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:background="#E1B4E3"
>
<TextView
android:layout_width="wrap_content"
android:textColor="@android:color/black"
android:layout_height="wrap_content"
android:text="Location Found" />
</RelativeLayout>
2.3 Enable Navigation
setDisplayHomeAsUpEnabled(true) Method of action bar is used enable Back/ Up navigation to activity. This function displays back arrow on Action Bar.
Add following code to enable Up Navigation:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.locationfound);
ActionBar actionBar = getActionBar();
// Enabling Up / Back navigation
actionBar.setDisplayHomeAsUpEnabled(true);
actionBar.setBackgroundDrawable(new ColorDrawable(Color.GRAY));
// actionBar.setIcon(R.drawable.ico_actionbar);
}
setBackgroundDrawable() Method is used to change background of ActionBar. And setIcon() Method is used to change icon of ActionBar.
More about ActionBar please refer the following link:
2.4 Add LocationFound activity in AndroidManifest.xml file
android:parentActivityName attribute is used to specify Up/ Back activity from current activity as following:
Step 3 Add ActionView with Search Functionality
An action view is a weight that appears as a substitute for an action button on ActionBar. We can perform our action without changing activity or fragment using this action view.
For Example:
- If you want to perform action for search, you can add action view to embed a searchview widget in ActionBar.
Following are the steps to add search action view on ActionBar:
3.1 actionmenu.xml
To add search view edit menu item in actionmenu.xml file as following: android:actionViewClass attribute is used to add search view on ActionBar.
<item
android:id="@+id/action_search"
android:actionViewClass="android.widget.SearchView"
android:icon="@drawable/ic_action_search"
android:showAsAction="always"
android:title="@string/action_search"/>
3.2 search.xml
Create search.xml file in xml folder as following:
<?xml version="1.0" encoding="utf-8"?>
<searchable xmlns:android="http://schemas.android.com/apk/res/android"
android:hint="Search your friend"
android:label="@string/app_name" />
3.3 onCreateOptionMenu() Method
Also edit onCreateOptionMenu() Method as following:
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.actionmenu, menu);
SearchManager searchManager = (SearchManager) getSystemService(Context.SEARCH_SERVICE);
SearchView searchView = (SearchView)menu.findItem(R.id.action_search).getActionView();
searchView.setSearchableInfo(searchManager.getSearchableInfo(getComponentName());
return true;
}
More About onCreateOptionsMenu() Method please refer the following link:
3.4 searchresult.xml
Now we need Activity that handles search result to display. For that add following searchresult.xml file in res and SearchResult Activity.
SearchResult.xml:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:background="#E1B4E3"
>
<TextView
android:layout_width="wrap_content"
android:textColor="@android:color/black"
android:layout_height="wrap_content"
android:id="@+id/txtQuery"
android:text="Location Found" />
</RelativeLayout>
3.5 SearchResult.java
public class SearchResult extends Activity {
private TextView txtQuery;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.searchresult);
ActionBar actionBar = getActionBar();
actionBar.setDisplayHomeAsUpEnabled(true);
txtQuery = (TextView) findViewById(R.id.txtQuery);
handleIntent(getIntent());
}
@Override
protected void onNewIntent(Intent intent) {
setIntent(intent);
handleIntent(intent);
}
private void handleIntent(Intent intent) {
if (Intent.ACTION_SEARCH.equals(intent.getAction())) {
String query = intent.getStringExtra(SearchManager.QUERY);
txtQuery.setText("Search Query: " + query);
}
}
}
Step 4 Adding spinner navigation
Add SearchResult activity in AndroidManifest.xml file as following:
Adding spinner drop-down navigation to switch between views within activity, action bar provides different navigation types. We can use tab or spinner drop-down navigation to navigate between views.
Following are the steps to add spinner drop-down to ActionBar:
4.1 onCreate() method from MainActivity.java
Edit MainActivity.java onCreate() Method as following:
private ActionBar actionBar;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
actionBar = getActionBar();
// Hide the action bar title
actionBar.setBackgroundDrawable(new ColorDrawable(Color.GRAY));
actionBar.setDisplayShowTitleEnabled(false);
actionBar.setNavigationMode(ActionBar.NAVIGATION_MODE_LIST);
final String[] dropdownValues = getResources().getStringArray(R.array.dropdown);
// Specify a SpinnerAdapter to populate the dropdown list.
ArrayAdapter adapter = new ArrayAdapter(actionBar.getThemedContext(), android.R.layout.simple_spinner_item, android.R.id.text1, dropdownValues);
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
// Set up the dropdown list navigation in the action bar.
actionBar.setListNavigationCallbacks(adapter, this);
}
Here dropdown is a string as defined in string resource as following:
<string-array name="dropdown">
<item>First Item</item>
<item>Second Item</item>
</string-array>
4.2 override onNavigationItemSelected() method
Implements onNavigationListner and override onNavigationItemSelected() Method as following:
@Override
public boolean onNavigationItemSelected(int itemPosition, long itemId) {
Fragment fragment = new MyFragment();
Bundle args = new Bundle();
args.putInt(MyFragment.ARG_SECTION_NUMBER, itemPosition);
fragment.setArguments(args);
getFragmentManager().beginTransaction().replace(R.id.container, fragment).commit();
return true;
}
For More About onNavigationItemSelected() please refer the following link:
4.3 MyFragment class
Define MyFragment Class as following in MainActivity.java.
public static class MyFragment extends Fragment {
public static final String ARG_SECTION_NUMBER = "placeholder_text";
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
TextView textView = new TextView(getActivity());
textView.setGravity(Gravity.CENTER);
textView.setText(getResources().getStringArray(R.array.dropdown)[getArguments().getInt(ARG_SECTION_NUMBER)]);
textView.setTextColor(Color.BLACK);
return textView;
}
}
4.4 action_main.xml file
Edit layout activity_main.xml as following to add framelayout:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:background="#E1B4E3"
tools:context=".MainActivity" >
<TextView
android:id="@+id/tv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/hello_world"
android:textColor="@android:color/black" />
<FrameLayout
android:id="@+id/container"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/tv" >
</FrameLayout>
</RelativeLayout>
I hope you have learnt How to add Actionbar in Android. If you have any questions or concerns, please put a comment here and I will get back to you ASAP.
Got an Idea of Android App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Android App Development Company.
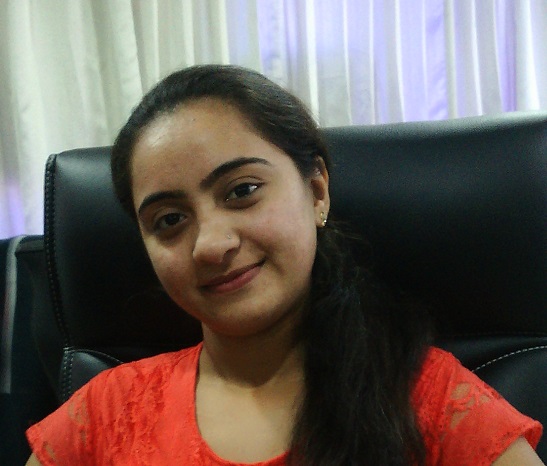
I am android developer, and do development not because to get paid or get adulation by the public, but because it is fun to program. I have been working in this field since one and half year.