
Be patient..... we are fetching your source code.
Objective
The main objective of this code sample is to explain how to crop 2D sprite in unity.
In version 4.3 and later, Unity introduces new 2D tools for 2D game play.
Step 1 Sprite
Sprite is one of the new functionality of 2D tools that is used in 2D games. Setting the texture importer of an image in project explorer can create sprite.
To create sprite, just drag and drop an image in assets folder and set Sprite Importer settings in inspector.
Texture Type | Set texture type to sprite for sprite 2D object. |
Sprite Mode | Sprite mode gives options whether single or multiple. Multiple sprite mode is used to slice the texture and creates multiple sprites. |
Packing Tag | The name of an optional sprite atlas into which this sprite texture should be packed. |
Pixels To Units | Used to set number of pixels per unit in world space. |
Pivot | Sets pivot of sprite. |
After creating sprite, it can be used as graphic in scene with the help of Sprite Renderer component.
Step 2 Sprite Renderer
Understand About Sprite Renderer:
- Sprite Renderer component is used to access or display the sprite in scene.
Some useful properties of 2D sprite renderer:
Sprite | Defines or hold the 2D sprite object. |
Color | Sets color of render mesh. |
Material | Defines the material that is used to render the 2D sprite. |
Sorting Layer | Defines the sprite’s rendering priority. |
Order In Layer | Defines the sprite’s rendering order (priority) within its layer. |
Step 3 Example
The following simple c# code will draw simple line rectangle on mouse move and crop the 2D sprite when mouse up.
3.1 Basic Setup
Add empty game object and put following script on it.
Create sprite as described above and add BoxCollider or BoxCollider2D component on it.
Rotation of sprite must be (0,0,0);.
Video:
3.2 CropSprite Script
using UnityEngine;
using System.Collections;
public class CropSprite : MonoBehaviour
{
// Reference for sprite which will be cropped and it has BoxCollider or BoxCollider2D
public GameObject spriteToCrop;
private Vector3 startPoint, endPoint;
private bool isMousePressed;
// For sides of rectangle. Rectangle that will display cropping area
private LineRenderer leftLine, rightLine, topLine, bottomLine;
void Start ()
{
isMousePressed = false;
// Instantiate rectangle sides
leftLine = createAndGetLine("LeftLine");
rightLine = createAndGetLine("RightLine");
topLine = createAndGetLine("TopLine");
bottomLine = createAndGetLine("BottomLine");
}
// Creates line through LineRenderer component
private LineRenderer createAndGetLine (string lineName)
{
GameObject lineObject = new GameObject(lineName);
LineRenderer line = lineObject.AddComponent();
line.SetWidth(0.03f,0.03f);
line.SetVertexCount(2);
return line;
}
void Update ()
{
if(Input.GetMouseButtonDown(0) && isSpriteTouched(spriteToCrop))
{
isMousePressed = true;
startPoint = Camera.main.ScreenToWorldPoint(Input.mousePosition);
}
else if(Input.GetMouseButtonUp(0))
{
if(isMousePressed)
cropSprite();
isMousePressed = false;
}
if(isMousePressed && isSpriteTouched(spriteToCrop))
{
endPoint = Camera.main.ScreenToWorldPoint(Input.mousePosition);
drawRectangle();
}
}
// Following method draws rectangle that displays cropping area
private void drawRectangle()
{
leftLine.SetPosition(0, new Vector3(startPoint.x, endPoint.y, 0));
leftLine.SetPosition(1, new Vector3(startPoint.x, startPoint.y, 0));
rightLine.SetPosition(0, new Vector3(endPoint.x, endPoint.y, 0));
rightLine.SetPosition(1, new Vector3(endPoint.x, startPoint.y, 0));
topLine.SetPosition(0, new Vector3(startPoint.x, startPoint.y, 0));
topLine.SetPosition(1, new Vector3(endPoint.x, startPoint.y, 0));
bottomLine.SetPosition(0, new Vector3(startPoint.x, endPoint.y, 0));
bottomLine.SetPosition(1, new Vector3(endPoint.x, endPoint.y, 0));
}
// Following method crops as per displayed cropping area
private void cropSprite()
{
// Calculate topLeftPoint and bottomRightPoint of drawn rectangle
Vector3 topLeftPoint = startPoint, bottomRightPoint=endPoint;
if((startPoint.x > endPoint.x))
{
topLeftPoint = endPoint;
bottomRightPoint = startPoint;
}
if(bottomRightPoint.y > topLeftPoint.y)
{
float y = topLeftPoint.y;
topLeftPoint.y = bottomRightPoint.y;
bottomRightPoint.y = y;
}
SpriteRenderer spriteRenderer = spriteToCrop.GetComponent();
Sprite spriteToCropSprite = spriteRenderer.sprite;
Texture2D spriteTexture = spriteToCropSprite.texture;
Rect spriteRect = spriteToCrop.GetComponent().sprite.textureRect;
int pixelsToUnits = 100; // It's PixelsToUnits of sprite which would be cropped
// Crop sprite
GameObject croppedSpriteObj = new GameObject("CroppedSprite");
Rect croppedSpriteRect = spriteRect;
croppedSpriteRect.width = (Mathf.Abs(bottomRightPoint.x - topLeftPoint.x)*pixelsToUnits)* (1/spriteToCrop.transform.localScale.x);
croppedSpriteRect.x = (Mathf.Abs(topLeftPoint.x - (spriteRenderer.bounds.center.x-spriteRenderer.bounds.size.x/2)) *pixelsToUnits)* (1/spriteToCrop.transform.localScale.x);
croppedSpriteRect.height = (Mathf.Abs(bottomRightPoint.y - topLeftPoint.y)*pixelsToUnits)* (1/spriteToCrop.transform.localScale.y);
croppedSpriteRect.y = ((topLeftPoint.y - (spriteRenderer.bounds.center.y - spriteRenderer.bounds.size.y/2))*(1/spriteToCrop.transform.localScale.y))* pixelsToUnits - croppedSpriteRect.height;//*(spriteToCrop.transform.localScale.y);
Sprite croppedSprite = Sprite.Create(spriteTexture, croppedSpriteRect, new Vector2(0,1), pixelsToUnits);
SpriteRenderer cropSpriteRenderer = croppedSpriteObj.AddComponent();
cropSpriteRenderer.sprite = croppedSprite;
topLeftPoint.z = -1;
croppedSpriteObj.transform.position = topLeftPoint;
croppedSpriteObj.transform.parent = spriteToCrop.transform.parent;
croppedSpriteObj.transform.localScale = spriteToCrop.transform.localScale;
Destroy(spriteToCrop);
}
// Following method checks whether sprite is touched or not. There are two methods for simple collider and 2DColliders. you can use as per requirement and comment another one.
// For simple 3DCollider
// private bool isSpriteTouched(GameObject sprite)
// {
// RaycastHit hit;
// Ray ray = Camera.main.ScreenPointToRay (Input.mousePosition);
// if (Physics.Raycast (ray, out hit))
// {
// if (hit.collider != null && hit.collider.name.Equals (sprite.name))
// return true;
// }
// return false;
// }
// For 2DCollider
private bool isSpriteTouched(GameObject sprite)
{
Vector3 posFor2D = Camera.main.ScreenToWorldPoint(Input.mousePosition);
RaycastHit2D hit2D = Physics2D.Raycast(posFor2D, Vector2.zero);
if (hit2D != null && hit2D.collider != null)
{
if(hit2D.collider.name.Equals(sprite.name))
return true;
}
return false;
}
}
If you got any query related to How To crop 2Dsprite in unity then comment them below. We will try to solve them out.
Got an Idea of Game Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Game Development Company in India.
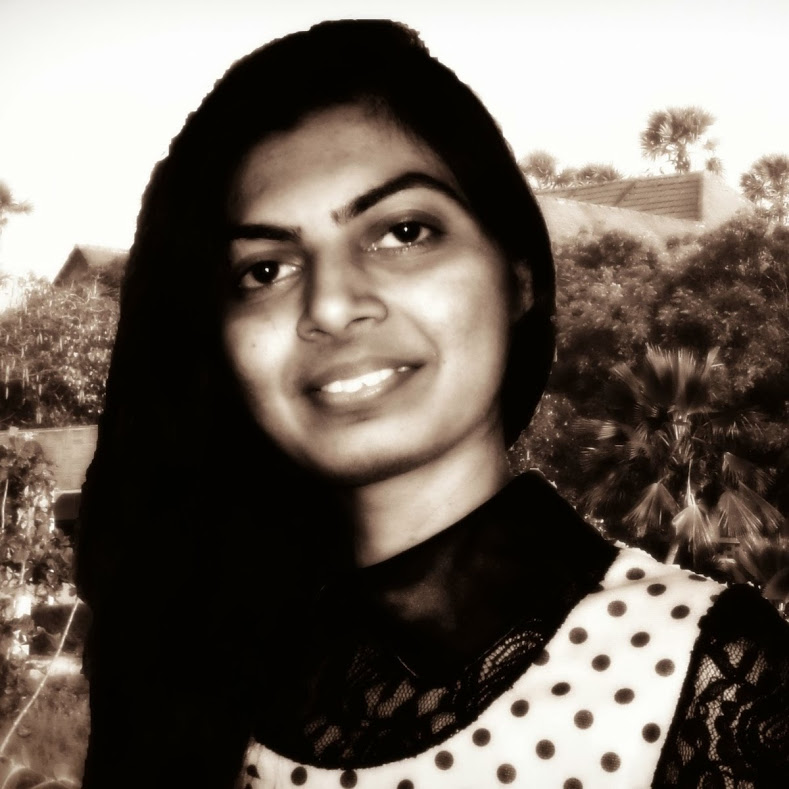
I am professional Game Developer, developing games in cocos2d(for iOS) and unity(for all platforms). Games are my passion and I aim to create addictive, high quality games. I have been working in this field since 1 year.
How Sliding View Controller works in iOS