
Be patient..... we are fetching your source code.
Objective
Center of gravity is a part of physics applied to game objects in a game. So let's take an overview of physics first.
You will get final output like this:
Step 1 Physics in Unity
To have a realistic physical behavior, your gameObject must accelerate correctly and should be affected by collisions, gravity and various other forces. This feature for physical simulation is provided by physics engine in unity.
After understanding physics, let’s understand what gravity is.
1.1 Gravity
Gravity can be described as force that pulls all matter together. Matter includes anything which is tangible. Gravity is linearly proportional to matter. So, thing which has a lot of matter,
For example planets, moon, stars will have more gravity to pull the things more strongly.
Mass is how we measure the amount of matter in something. The more massive something is, the more of gravitational pull it exerts. As we walk on the surface of Earth, it pulls us and we pull back. But since the Earth is much more massive than we are, the pull from us is not strong enough to move the Earth, while the pull from the Earth can make us fall flat on our faces.
In addition to depending on the amount of mass, gravity also depends on how far you are from something. This is why we are stuck to the surface of the Earth instead of being pulled off into the Sun, whose gravity is much more times than that of the Earth.
Now, for implementing gravity’s effect on planet in your game, let’s make a short demo on it.
Step 2 Demo Project
2.1 Project Setup
Open Unity and go to File >> New Project. Give the name Center Of Gravity Project and select 2D from the drop down menu and press create button.
Create a scene with Main Camera, Planet’s sprite, Bird’s Sprite and Canvas that includes Text inside it as shown below:
You can download images of planet and bird from the internet and convert it into 2D sprite as shown below:
After converting an image into 2D sprite, you can use that sprite as your gameObject inside your scene view. For giving any information inside game view, go to GameObject >> UI >> Text and you can write your information inside that text. Here, I have used the text Press Space for writing inside the game view.
Now, for rotating your bird around the planet, write a C# script as below and apply it to your bird.
2.2 Bird.cs Script
using UnityEngine;
using System.Collections;
public class Bird : MonoBehaviour
{
public Transform planet;
private float forceAmountForRotation = 10;
private Vector3 directionOfPlanetFromBird;
private bool allowForce;
void Start()
{
directionOfPlanetFromBird = Vector3.zero;
}
void Update ()
{
allowForce = false;
if (Input.GetKey(KeyCode.Space))
allowForce = true;
directionOfPlanetFromBird = transform.position - planet.position;
transform.right = Vector3.Cross(directionOfPlanetFromBird, Vector3.forward);
}
void FixedUpdate ()
{
if (allowForce)
rigidbody2D.AddForce (transform.right * forceAmountForRotation);
}
}
For giving the gravitational force that can pull the bird towards the center of the planet, write a C# script as below and apply it to your Planet.
2.3 Planet.cs Script
using UnityEngine;
using System.Collections;
public class Planet : MonoBehaviour
{
public Transform bird;
private float gravitationalForce = 5;
private Vector3 directionOfBirdFromPlanet;
void Start ()
{
directionOfBirdFromPlanet = Vector3.zero;
}
void FixedUpdate ()
{
directionOfBirdFromPlanet = (transform.position-bird.position).normalized;
bird.rigidbody2D.AddForce (directionOfBirdFromPlanet*gravitationalForce);
}
}
I hope you find this blog is very helpful while reating effect like center of gravity of planet in unity. Let me know in comment if you have any questions regarding Unity. I will reply you ASAP.
Got an Idea of Game Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Game Development Company in India.
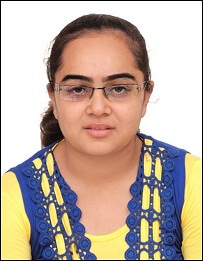
I am an enthusiastic game developer who likes to explore different gaming concepts to enhance the knowledge and polish the development skills.
Unity Tips for Beginners