
Be patient..... we are fetching your source code.
Objective
The Main Objective of this blog post gives you brief idea about how to implement local notifications in android.
Step 1 Introduction
Local notifications is used for notify user about your application’s updates or news. I will teach you how to implement local notification in your android application. Before moving forward, I would suggest you to refer Android Notifications.
In this blog I will cover different types of notifications:
- Simple Notification
- Expanded Layout Notification
- Notifications with Action Buttons
- Notifications With Priority
- Combine Multiple Notifications
Follow these simple steps to create a local notification in your Android App.
Step 2 Create New Android Project
If you are new to Android studio and don't know how to create a new project then see how to Create New Project in Android Studio
Step 3 Create Application Layout File
Now, in this blog I am taking notification title and notification description from user.
So for that I am updating my activity_main.xml file as follow
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.tag.localnotificationdemo.MainActivity">
<ScrollView
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<EditText
android:id="@+id/etMainNotificationTitle"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="@string/notification_title" />
<EditText
android:id="@+id/etMainNotificationText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="@string/write_your_text_here" />
<Button
android:id="@+id/btnMainSendSimpleNotification"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="50dp"
android:text="@string/send_simple_notification" />
<Button
android:id="@+id/btnMainSendExpandLayoutNotification"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="@string/send_expand_layout_notification" />
<Button
android:id="@+id/btnMainSendNotificationActionBtn"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="@string/send_notification_with_action_buttons" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<Button
android:id="@+id/btnMainSendMaxPriorityNotification"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_weight="1"
android:text="@string/send_max_priority_notification" />
<Button
android:id="@+id/btnMainSendMinPriorityNotification"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_weight="1"
android:text="@string/send_min_priority_notification" />
</LinearLayout>
<Button
android:id="@+id/btnMainSendCombinedNotification"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="@string/send_combined_notification" />
<Button
android:id="@+id/btnMainClearAllNotification"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="@string/clear_all_notification" />
</LinearLayout>
</ScrollView>
</LinearLayout>
In this layout file, I have taken:
Two EditTexts |
|
Seven Buttons |
|
I am done with my layout and I have taken all string reference in strings.xml.
Step 4 Update strings.xml file
Put strings.xml file on following path app >> res >> values >> strings.xml. So my strings.xml file will look like this.
strings.xml
<resources>
<string name="app_name">Local Notification Demo</string>
<string name="write_your_text_here">Write Your Text Here(optional)</string>
<string name="dismiss">Dismiss</string>
<string name="snooze">Snooze</string>
<string name="send_simple_notification">Send Simple Notification</string>
<string name="send_expand_layout_notification">Send Expand Layout Notification</string>
<string name="send_notification_with_action_buttons">Send Notification With Action Buttons</string>
<string name="send_max_priority_notification">Send Max Priority Notification</string>
<string name="send_min_priority_notification">Send Min Priority Notification</string>
<string name="send_combined_notification">Send Combined Notification</string>
<string name="clear_all_notification">Clear All Notifications</string>
<string name="notification_title">Notification Title(optional)</string>
</resources>
In strings.xml file I have declared button texts and edit text hint. I am done with my layout file, so now let’s move towards the coding part of the application.
Step 5 Update MainActivity.java file
MainActivity.java
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
private int currentNotificationID = 0;
private EditText etMainNotificationText, etMainNotificationTitle;
private Button btnMainSendSimpleNotification, btnMainSendExpandLayoutNotification, btnMainSendNotificationActionBtn, btnMainSendMaxPriorityNotification, btnMainSendMinPriorityNotification, btnMainSendCombinedNotification, btnMainClearAllNotification;
private NotificationManager notificationManager;
private NotificationCompat.Builder notificationBuilder;
private String notificationTitle;
private String notificationText;
private Bitmap icon;
private int combinedNotificationCounter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
getAllWidgetReference();
bindWidgetWithAnEvent();
}
private void getAllWidgetReference() {
etMainNotificationText = (EditText) findViewById(R.id.etMainNotificationText);
etMainNotificationTitle = (EditText) findViewById(R.id.etMainNotificationTitle);
btnMainSendSimpleNotification = (Button) findViewById(R.id.btnMainSendSimpleNotification);
btnMainSendExpandLayoutNotification = (Button) findViewById(R.id.btnMainSendExpandLayoutNotification);
btnMainSendNotificationActionBtn = (Button) findViewById(R.id.btnMainSendNotificationActionBtn);
btnMainSendMaxPriorityNotification = (Button) findViewById(R.id.btnMainSendMaxPriorityNotification);
btnMainSendMinPriorityNotification = (Button) findViewById(R.id.btnMainSendMinPriorityNotification);
btnMainSendCombinedNotification = (Button) findViewById(R.id.btnMainSendCombinedNotification);
btnMainClearAllNotification = (Button) findViewById(R.id.btnMainClearAllNotification);
notificationManager = (NotificationManager) this.getSystemService(Context.NOTIFICATION_SERVICE);
icon = BitmapFactory.decodeResource(this.getResources(),
R.mipmap.ic_launcher);
}
private void bindWidgetWithAnEvent() {
btnMainSendSimpleNotification.setOnClickListener(this);
btnMainSendExpandLayoutNotification.setOnClickListener(this);
btnMainSendNotificationActionBtn.setOnClickListener(this);
btnMainSendMaxPriorityNotification.setOnClickListener(this);
btnMainSendMinPriorityNotification.setOnClickListener(this);
btnMainSendCombinedNotification.setOnClickListener(this);
btnMainClearAllNotification.setOnClickListener(this);
}
@Override
public void onClick(View v) {
setNotificationData();
switch (v.getId()) {
case R.id.btnMainSendSimpleNotification:
setDataForSimpleNotification();
break;
case R.id.btnMainSendExpandLayoutNotification:
setDataForExpandLayoutNotification();
break;
case R.id.btnMainSendNotificationActionBtn:
setDataForNotificationWithActionButton();
break;
case R.id.btnMainSendMaxPriorityNotification:
setDataForMaxPriorityNotification();
break;
case R.id.btnMainSendMinPriorityNotification:
setDataForMinPriorityNotification();
break;
case R.id.btnMainSendCombinedNotification:
setDataForCombinedNotification();
break;
case R.id.btnMainClearAllNotification:
clearAllNotifications();
break;
}
}
private void sendNotification() {
Intent notificationIntent = new Intent(this, MainActivity.class);
PendingIntent contentIntent = PendingIntent.getActivity(this, 0, notificationIntent, PendingIntent.FLAG_UPDATE_CURRENT);
notificationBuilder.setContentIntent(contentIntent);
Notification notification = notificationBuilder.build();
notification.flags |= Notification.FLAG_AUTO_CANCEL;
notification.defaults |= Notification.DEFAULT_SOUND;
currentNotificationID++;
int notificationId = currentNotificationID;
if (notificationId == Integer.MAX_VALUE - 1)
notificationId = 0;
notificationManager.notify(notificationId, notification);
}
private void clearAllNotifications() {
if (notificationManager != null) {
currentNotificationID = 0;
notificationManager.cancelAll();
}
}
private void setNotificationData() {
notificationTitle = this.getString(R.string.app_name);
notificationText = "Hello..This is a Notification Test";
if (!etMainNotificationText.getText().toString().equals("")) {
notificationText = etMainNotificationText.getText().toString();
}
if (!etMainNotificationTitle.getText().toString().equals("")) {
notificationTitle = etMainNotificationTitle.getText().toString();
}
}
private void setDataForSimpleNotification() {
notificationBuilder = new NotificationCompat.Builder(this)
.setSmallIcon(R.mipmap.ic_launcher)
.setLargeIcon(icon)
.setContentTitle(notificationTitle)
.setContentText(notificationText);
sendNotification();
}
private void setDataForExpandLayoutNotification() {
notificationBuilder = new NotificationCompat.Builder(this)
.setSmallIcon(R.mipmap.ic_launcher)
.setLargeIcon(icon)
.setContentTitle(notificationTitle)
.setStyle(new NotificationCompat.BigTextStyle().bigText(notificationText))
.setContentText(notificationText);
sendNotification();
}
private void setDataForNotificationWithActionButton() {
notificationBuilder = new NotificationCompat.Builder(this)
.setSmallIcon(R.mipmap.ic_launcher)
.setLargeIcon(icon)
.setContentTitle(notificationTitle)
.setStyle(new NotificationCompat.BigTextStyle().bigText(notificationText))
.setContentText(notificationText);
Intent answerIntent = new Intent(this, AnswerReceiveActivity.class);
answerIntent.setAction("Yes");
PendingIntent pendingIntentYes = PendingIntent.getActivity(this, 1, answerIntent, PendingIntent.FLAG_UPDATE_CURRENT);
notificationBuilder.addAction(R.drawable.thumbs_up, "Yes", pendingIntentYes);
answerIntent.setAction("No");
PendingIntent pendingIntentNo = PendingIntent.getActivity(this, 1, answerIntent, PendingIntent.FLAG_UPDATE_CURRENT);
notificationBuilder.addAction(R.drawable.thumbs_down, "No", pendingIntentNo);
sendNotification();
}
private void setDataForMaxPriorityNotification() {
notificationBuilder = new NotificationCompat.Builder(this)
.setSmallIcon(R.mipmap.ic_launcher)
.setLargeIcon(icon)
.setContentTitle(notificationTitle)
.setStyle(new NotificationCompat.BigTextStyle().bigText(notificationText))
.setPriority(Notification.PRIORITY_MAX)
.setContentText(notificationText);
sendNotification();
}
private void setDataForMinPriorityNotification() {
notificationBuilder = new NotificationCompat.Builder(this)
.setSmallIcon(R.mipmap.ic_launcher)
.setLargeIcon(icon)
.setContentTitle(notificationTitle)
.setStyle(new NotificationCompat.BigTextStyle().bigText(notificationText))
.setPriority(Notification.PRIORITY_MIN)
.setContentText(notificationText);
sendNotification();
}
private void setDataForCombinedNotification() {
combinedNotificationCounter++;
notificationBuilder = new NotificationCompat.Builder(this)
.setSmallIcon(R.mipmap.ic_launcher)
.setLargeIcon(icon)
.setContentTitle(notificationTitle)
.setGroup("group_emails")
.setGroupSummary(true)
.setContentText(combinedNotificationCounter + " new messages");
NotificationCompat.InboxStyle inboxStyle = new NotificationCompat.InboxStyle();
inboxStyle.setBigContentTitle(notificationTitle);
inboxStyle.setSummaryText("[email protected]");
for (int i = 0; i < combinedNotificationCounter; i++) {
inboxStyle.addLine("This is Test" + i);
}
currentNotificationID = 500;
notificationBuilder.setStyle(inboxStyle);
sendNotification();
}
}
Now, let’s understand MainActivity.java step by step.
5.1 Understand getAllWidgetReference() Method
In getAllWidgetReference() method I have taken the reference of edit texts and buttons that I have taken in layout file and I have assigned notificationManager variable for the notification service.
I have created a bitmap image of my application icon for notification Icon and assigned it to the icon (Bitmap variable).
5.2 Understand bindWidgetWithAnEvent() Method
In bindWidgetWithAnEvent() method I have assigned what action will be performed when user clicks the button that I have created in the layout.
5.3 Understand setNotificationData() Method
private void setNotificationData() {
notificationTitle = this.getString(R.string.app_name);
notificationText = "Hello..This is a Notification Test";
if (!etMainNotificationText.getText().toString().equals("")) {
notificationText = etMainNotificationText.getText().toString();
}
if (!etMainNotificationTitle.getText().toString().equals("")) {
notificationTitle = etMainNotificationTitle.getText().toString();
}
}
setNotificationData() method will set a title and description of the notification. I have taken notificationTitle and notificationText variables for Notification Title and Notification Description respectively.
If there is no text in notificationTitle edittext then it will take default title text (here I have taken app name as a default title) and same as notificationText.
5.4 Understand sendNotification() Method
private void sendNotification() {
Intent notificationIntent = new Intent(this, MainActivity.class);
PendingIntent contentIntent = PendingIntent.getActivity(this, 0, notificationIntent, PendingIntent.FLAG_UPDATE_CURRENT);
notificationBuilder.setContentIntent(contentIntent);
Notification notification = notificationBuilder.build();
notification.flags |= Notification.FLAG_AUTO_CANCEL;
notification.defaults |= Notification.DEFAULT_SOUND;
currentNotificationID++;
int notificationId = currentNotificationID;
if (notificationId == Integer.MAX_VALUE - 1)
notificationId = 0;
notificationManager.notify(notificationId, notification);
}
You need to call sendNotification() method after setting notifiactionBuilder object as per your need. In this method I have defined Intent and PendingIntent object to redirect user to some activity when user touches the notification.
To assign some action when user touches the notification, I need to set PendingIntent object in notificationBuilder.setContentIntent() method. If I don’t define notificationBuilder.setContentIntent() method then it will do nothing when user touches the notification.
In Intent object (here notificationIntent) I have defined MainActivity.class so it will be redirected to MainActivity of our app when user touches the notification. For more detail about pendingIntent.getActivity() refer this. This method is responsible for sending notifications to the user.
NotificationCompat.Builder is used to set notification icon, text, style, title etc...
For more information about NotificationCompat.Builder refer this.
For different types of notifications we have to set notificationBuilder object as per requirements. Now let’s learn how to send different types of notifications
After Setting all the things just change the notification builder object to get different types of notifications.
5.4.1 Simple Notification
To send the simple notification to the user just set the notificationBuilder object as follows.
private void setDataForSimpleNotification() {
notificationBuilder = new NotificationCompat.Builder(this)
.setSmallIcon(R.mipmap.ic_launcher)
.setLargeIcon(icon)
.setContentTitle(notificationTitle)
.setContentText(notificationText);
}
This type of notifications cannot be expanded.
5.4.2 Expanded Layout Notification
To send the Expanded layout notification to the user just set the notificationBuilder object as follows.
private void setDataForExpandLayoutNotification() {
notificationBuilder = new NotificationCompat.Builder(this)
.setSmallIcon(R.mipmap.ic_launcher)
.setLargeIcon(icon)
.setContentTitle(notificationTitle)
.setStyle(new NotificationCompat.BigTextStyle().bigText(notificationText))
.setContentText(notificationText);
}
5.4.3 Notification With Action Buttons
To add action buttons in the notification, just follow these simple steps.
private void setDataForNotificationWithActionButton() {
notificationBuilder = new NotificationCompat.Builder(this)
.setSmallIcon(R.mipmap.ic_launcher)
.setLargeIcon(icon)
.setContentTitle(notificationTitle)
.setStyle(new NotificationCompat.BigTextStyle().bigText(notificationText))
.setContentText(notificationText);
Intent answerIntent = new Intent(this, AnswerReceiveActivity.class);
answerIntent.setAction("Yes");
PendingIntent pendingIntentYes = PendingIntent.getActivity(this, 1, answerIntent, PendingIntent.FLAG_UPDATE_CURRENT);
notificationBuilder.addAction(R.drawable.thumbs_up, "Yes", pendingIntentYes);
answerIntent.setAction("No");
PendingIntent pendingIntentNo = PendingIntent.getActivity(this, 1, answerIntent, PendingIntent.FLAG_UPDATE_CURRENT);
notificationBuilder.addAction(R.drawable.thumbs_down, "No", pendingIntentNo);
sendNotification();
}
After setting notification builder, create pending intent for button actions. Here when user clicks on YES action I am setting intent action to yes and for NO action I am setting intent action to no.
So that in AnswerReceiveActivity I can get action name by getIntent().getAction() method as shown below.
Now I am creating empty activity named AnswerReceiveActivity.
activity_answer_receive.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.tag.localnotificationdemo.AnswerReceiveActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="You have selected : "
android:textSize="20sp" />
<TextView
android:id="@+id/tvAnswerReceiveText"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="20sp"
android:textStyle="bold" />
</LinearLayout>
AnswerReceiveActivity.java
public class AnswerReceiveActivity extends AppCompatActivity {
private TextView tvAnswerReceiveText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_answer_receive);
tvAnswerReceiveText = (TextView) findViewById(R.id.tvAnswerReceiveText);
Log.d("Main", getIntent().getAction());
tvAnswerReceiveText.setText(getIntent().getAction());
}
}
5.4.4 Notifications With Priority
You can set the priority of the notification whether it is in High Priority, Low Priority etc...
private void setDataForMaxPriorityNotification() {
notificationBuilder = new NotificationCompat.Builder(this)
.setSmallIcon(R.mipmap.ic_launcher)
.setLargeIcon(icon)
.setContentTitle(notificationTitle)
.setStyle(new NotificationCompat.BigTextStyle().bigText(notificationText))
.setPriority(Notification.PRIORITY_MAX)
.setContentText(notificationText);
sendNotification();
}
private void setDataForMinPriorityNotification() {
notificationBuilder = new NotificationCompat.Builder(this)
.setSmallIcon(R.mipmap.ic_launcher)
.setLargeIcon(icon)
.setContentTitle(notificationTitle)
.setStyle(new NotificationCompat.BigTextStyle().bigText(notificationText))
.setPriority(Notification.PRIORITY_MIN)
.setContentText(notificationText);
sendNotification();
}
There are different types of priorities as listed below:
Here I have given the example of PRIORITY_MAX and PRIORITY_MIN.
To set priority you just need to set setPriority() of notificationBuilder object.
PRIORITY_MAX | Use for critical and urgent notifications. |
PRIORITY_HIGH | Use primarily for important communications. |
PRIORITY_DEFAULT | Use for all notifications that don't fall into any of the other priorities described here. |
PRIORITY_LOW | Use for notifications that you want the user to be informed about, but that are less urgent. |
PRIORITY_MIN | Use for contextual or background information notifications. |
5.4.5 Combine Multiple Notifications
You can combine multiple notifications in to one notification.
Set notificationBuilder object as follow:
private void setDataForCombinedNotification() {
combinedNotificationCounter++;
notificationBuilder = new NotificationCompat.Builder(this)
.setSmallIcon(R.mipmap.ic_launcher)
.setLargeIcon(icon)
.setContentTitle(notificationTitle)
.setGroup("group_emails")
.setGroupSummary(true)
.setContentText(combinedNotificationCounter + " new messages");
NotificationCompat.InboxStyle inboxStyle = new NotificationCompat.InboxStyle();
inboxStyle.setBigContentTitle(notificationTitle);
inboxStyle.setSummaryText("[email protected]");
for (int i = 0; i < combinedNotificationCounter; i++) {
inboxStyle.addLine("This is Test" + i);
}
currentNotificationID = 500;
notificationBuilder.setStyle(inboxStyle);
}
Each time when I click send combined notification button, one notification would be added into the previous notification and all the notifications would be combined.
Note
When notifications are combined, the counter would be increased too.
"I hope you find this blog post very helpful while Easy Send Local Notification To User In Android. Let me know in comment if you have any questions regarding Tab Layout. I will reply you ASAP."
Learning Android sounds fun, right? Why not check out our other Android Tutorials?
Got an Idea of Android App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Android Application Development Company in India.
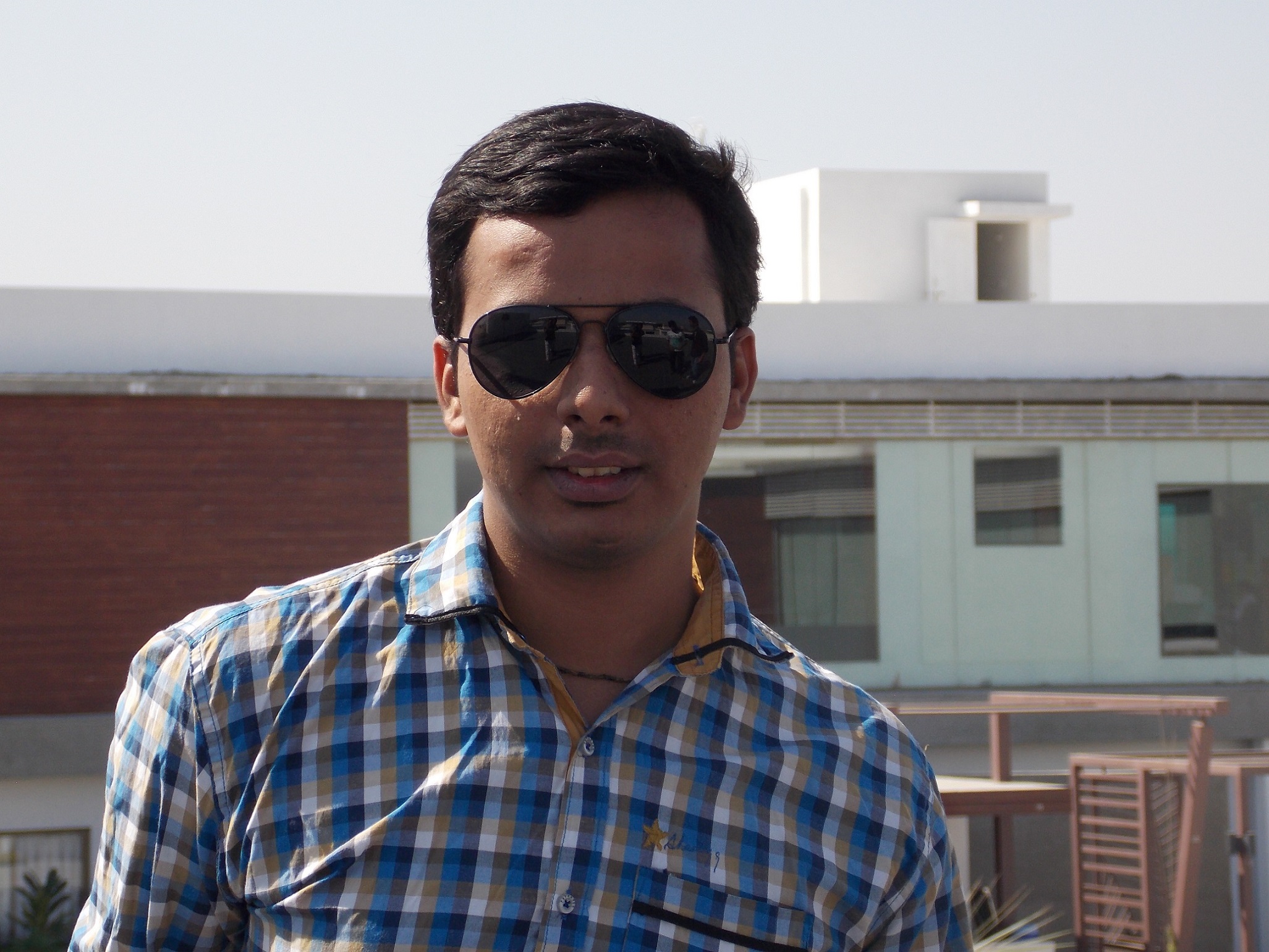
I am professional Android App & Game developer. I love to develop unique and creative Games & Apps. I am very passionate about my work.