Objective
Main objective of this blog post is to how to create custom packages in Laravel 4.
This blog has been written keeping in mind the reader’s knowledge regarding PHP and web server applications like XAMPP, WAMP, MAMP etc. Reader should know the concepts of PHP and MVC frameworks.
Step 1 Introduction
The idea of interoperability is the core concept or you may say 'The Idea' behind Laravel 4. It had been quite difficult to manage dependencies in PHP projects that lead to mismanagement in maintenance and coding. You might have heard about PEAR (PHP Extension and Application Repository). If not, let me explain it in this way, PEAR is a framework and distribution for reusable components. Developers use PEAR to download and use packages that are already available. So, they don’t need to reinvent the wheel.
Strongly inspired by node’s npm and ruby’s bundler, there comes the Composer. It is a dependency manager. It manages packages required in PHP projects and updates them on per-project basis, installing them in a directory ‘vendor’ inside your project.
Step 2 Installing Laravel
2.1 Installing Composer
Let’s start by installing composer on our system. Download composer from here. Its installation wizard is much easier. You just need to provide the location for the php.exe file of your web server (XAMPP / WAMP / MAMP etc.). Now to check whether composer has been installed correctly, open command prompt and type ‘composer’ (without quotes). If you get command list for composer, then it has been installed correctly (refer to composer website for more information on installing composer).
2.2 Open Root Folder
Now browse to your web server root folder in command prompt interface and type
composer create-project Laravel/Laravel your-project-name --prefer-dis
Here your-project-name should be your project name. You can see composer installing Laravel framework and its required packages. To installing Laravel framework on MAC or other system, please refer to Laravel website.
2.3 Exploring Laravel
Now assuming you are excited about exploring Laravel, let us create our first custom package in Laravel 4 (by default, you would be installing latest version of Laravel). You will find workbench.php file inside app/config folder. Open it and fill in your name and email address.
Open your command prompt and browse to project folder in it. Now type the following to create your first Laravel package.
php artisan workbench vendor/package --resources
Replace ‘vendor’ and ‘package’ name with your name and your package name respectively. Here ‘vendor’ can be anything like your name, your organization’s name, your spouse’s name etc. while ‘package’ will be your package name. For example, I would be creating my package as
php artisan workbench nirmal/firstpack --resources Package Structure
2.4 You Will See Package Name
You will see a directory created inside workbench directory having your package name. Before we proceed further, it’s good to install composer by typing following command
composer install
Note: In case you find yourself in a situation where you get a file not found error, you can always remap composer file by typing following command in console
composer dump-autoload
Now let’s understand the file structure or package structure.
Inside src directory, you keep your actual code for the package.
Inside tests directory, you keep your tests files.
Inside vendor directory, all your dependencies are stored.
Step 3 Package Service Provider
3.1 Find Your Package Services
You will find your package’s service provider inside src/yourname/yourpackagename/packageServiceProvider.
Mine is here: src/nirmal/firstpack/FirstpackServiceProvider.php
This file sets up everything after our package is installed. My service provider file looks as follows:
<?php namespace Nirmal\Firstpack; use Illuminate\Support\ServiceProvider; class FirstpackServiceProvider extends ServiceProvider { protected $defer = false; public function boot() { $this->package('nirmal/firstpack');
}
public function register()
{
//
}
public function provides()
{
return array();
}
}
See how boot() and provides() methods are initialized.
3.2 Register Service Provider
Next step is to register our service provider. So, open app/config/app.php file and add service provider to bottom of the “providers” array
'providers' => array(
// --
'Nirmal\Firstpack\FirstpackServiceProvider'
),
Now to confirm that our service provider has been added successfully, type following command in command prompt php artisan dump-autoload You will get the error (if any). Next let’s create our package class. Package Class
3.3 Locate Service Provider File
In the same directory where our service provider file is located, create a class file as below:
<?php namespace Nirmal\Firstpack;
class Firstpack {
public static function greet(){
return "Good morning guest!";
}
}
Note the namespaces, I have provided above each class. It is necessary to provide namespace to respective classes.
Back to our service provider file, let’s register our service provider.
Step 4 Register service provider
4.1 Inside Service Provider
Inside our service provider file, you will find register() method. Add following code to it, replacing the package name with your package name wherever necessary.
public function register()
{
$this->app['firstpack'] = $this->app->share(function($app) {
return new Firstpack;
});
}
Here $this->app is an array that holds all class instances while $this->app->share is a closure function that is returning an instance of our class. When our package is used, it will be resolved using this instance created from IoC container.
Creating a Facade
4.2 Accessed as class::mathod()
Those who have used Laravel before might know about some methods accessed as Class::method(). This is actually called a facade. It appears as if Laravel is allowing methods to be called statically. But in reality, it is actually resolving all these classes out of IoC container.
4.3 To create a facade
To create a facade, let’s create a directory inside our package folder. In this directory, create a file Firstpack.php and write following code:
<?php namespace Nirmal\Firstpack\Facades;
use Illuminate\Support\Facades\Facade;
class Firstpack extends Facade {
protected static function getFacadeAccessor()
{
return 'firstpack';
}
}
4.4 Append code to function in service provider class
Now append following code to our register function in service provider class
$this->app->booting(function()
{
$loader = \Illuminate\Foundation\AliasLoader::getInstance();
$loader->alias('Firstpack', 'Nirmal\Firstpack\Facades\Firstpack');
});
Execute
4.5 Now Access your package
You can now access your package by Firstpack::greet(). Now open routes.php file and add following code to it or you can add your own logic to print message
Route::get('/firstpack', function(){
echo Firstpack::greet();
});
You should now be greeted with the message 'Good morning guest!'
Conclusion
So far, we have created a package that greets us when executed. But you can explore a lot about it and customize your package in your own way. You can even publish your package on Packagist.
I will be back with more tutorials on Laravel. Till then stay safe and be happy. If you are facing problem in Creating Custom Packages in Laravel. comment here I will help you ASAP.
Got an Idea of Web Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Web Development Company in India.
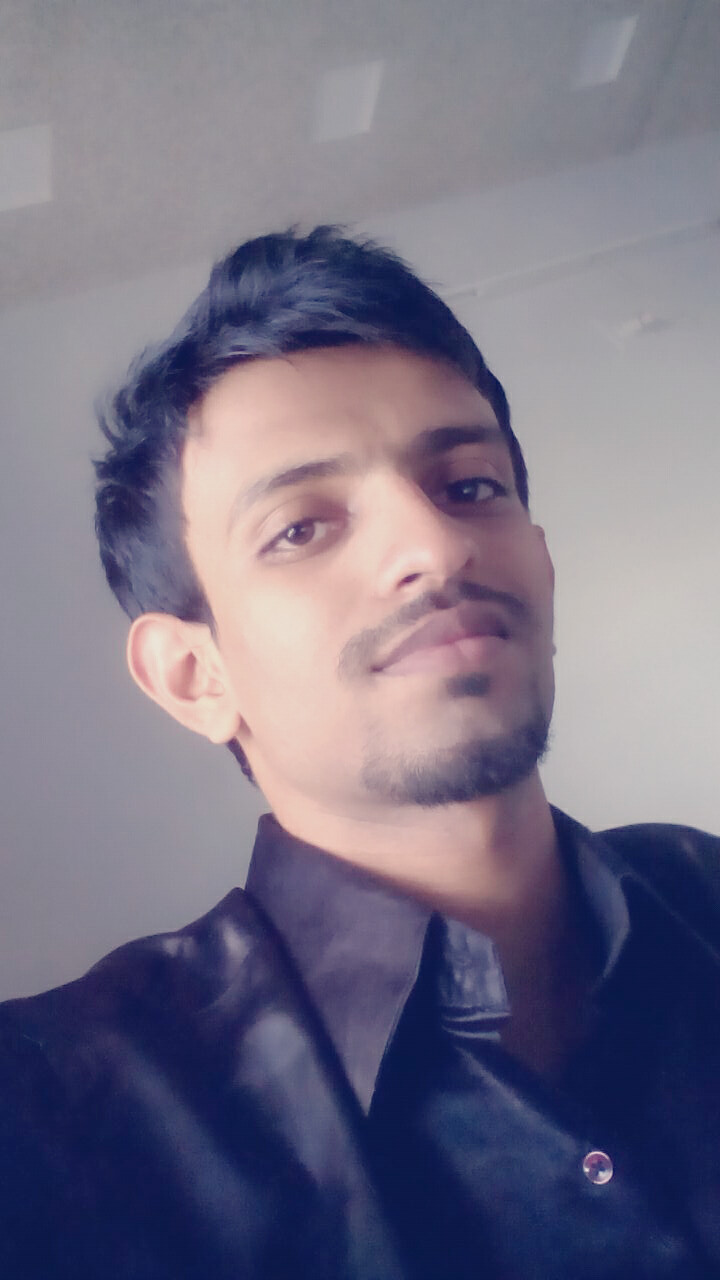
How to setup Laravel 4 on MAC
Working With Crop Tools in Photoshop