
Be patient..... we are fetching your source code.
Objective
The main objective of this code sample is to explain how to write an AI for enemy player to detect and avoid obstacle easily using Physics.RayCast.
Video:
Step 1 Physic.RayCast
Understanding About Physics.RayCast:
A Physics.RayCast is used to detect if there is any object having collider within the given range and specified direction. Physics.RayCast is a static method which returns boolean value i.e true if there is anything and false if there is nothing.
Static bool Raycast(Vector3 origin, Vector3 direction, RaycastHit hit, float distance = 10f , int layerMask = DefaultRaycastLayers);
origin | Starting Point of ray. |
direction | Direction in which to ray cast. |
hit | It will return more info about collider that was hit. |
distance | Range or length of the ray. |
layerMask | A Layer mask that is used to selectively ignore colliders when casting a ray. |
Step 2 Example
In the given example, Phyics.RayCast() is used on a cube which moves towards the targets and overcome the obstacles,
Here 4 rays are used to show how to avoid the obstacles.
- Two rays are set in front: The front rays are used to detect the obstacles, if obstacles are found the cube is rotated in either left or right direction to avoid the obstacle. When front rays detect the obstacle, it should focus only on the obstacle for that point in time.
- Two rays are set in backside of the cube: The backside rays are used to confirm that obstacles are avoided which front rays previously detected. As soon as obstacle is passed through it again focuses on target.
Screenshots:
2.1 Enemy AI
using UnityEngine;
using System.Collections;
public class EnemyAI: MonoBehaviour {
// Fix a range how early u want your enemy detect the obstacle.
private int range;
private float speed;
private bool isThereAnyThing = false;
// Specify the target for the enemy.
public GameObject target;
private float rotationSpeed;
private RaycastHit hit;
// Use this for initialization
void Start() {
range = 80;
speed = 10 f;
rotationSpeed = 15 f;
}
// Update is called once per frame
void Update() {
//Look At Somthly Towards the Target if there is nothing in front.
if (!isThereAnyThing) {
Vector3 relativePos = target.transform.position - transform.position;
Quaternion rotation = Quaternion.LookRotation(relativePos);
transform.rotation = Quaternion.Slerp(transform.rotation, rotation, Time.deltaTime);
}
// Enemy translate in forward direction.
transform.Translate(Vector3.forward * Time.deltaTime * speed);
//Checking for any Obstacle in front.
// Two rays left and right to the object to detect the obstacle.
Transform leftRay = transform;
Transform rightRay = transform;
//Use Phyics.RayCast to detect the obstacle
if (Physics.Raycast(leftRay.position + (transform.right * 7), transform.forward, out hit, range) || Physics.Raycast(rightRay.position - (transform.right * 7), transform.forward, out hit, range)) {
if (hit.collider.gameObject.CompareTag("Obstacles")) {
isThereAnyThing = true;
transform.Rotate(Vector3.up * Time.deltaTime * rotationSpeed);
}
}
// Now Two More RayCast At The End of Object to detect that object has already pass the obsatacle.
// Just making this boolean variable false it means there is nothing in front of object.
if (Physics.Raycast(transform.position - (transform.forward * 4), transform.right, out hit, 10) ||
Physics.Raycast(transform.position - (transform.forward * 4), -transform.right, out hit, 10)) {
if (hit.collider.gameObject.CompareTag("Obstacles")) {
isThereAnyThing = false;
}
}
// Use to debug the Physics.RayCast.
Debug.DrawRay(transform.position + (transform.right * 7), transform.forward * 20, Color.red);
Debug.DrawRay(transform.position - (transform.right * 7), transform.forward * 20, Color.red);
Debug.DrawRay(transform.position - (transform.forward * 4), -transform.right * 20, Color.yellow);
Debug.DrawRay(transform.position - (transform.forward * 4), transform.right * 20, Color.yellow);
}
}
Note
Add this script to enemy player or the enemy object and assign target to the enemy.
If you have got any query related to Unity 3D - Enemy Obstacle Awareness then comment them below we will try to solve them out. Got an Idea of Game Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best 3D Game Development Company in India.
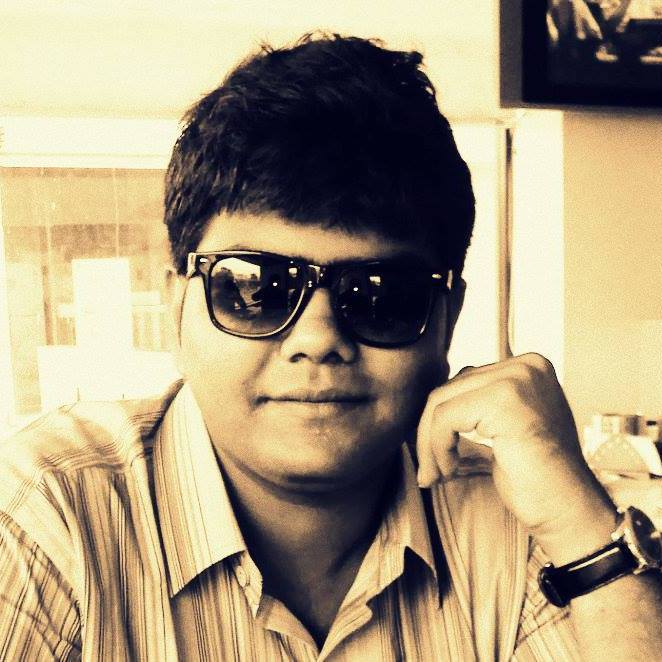
I am professional game developer, developing games in Unity 3D and I am very passionate about my work.