
Be patient..... we are fetching your source code.
Objective
Main objective of this blog post is to help you create scroll view in Unity3d.
Step 1 ScrollView
When something is too big to fit to screen, then ScrollView is the solution. Let’s imagine if you have player selection in your game, having multiple players.
The number of players can’t fit physical display of any device. Now in this case, use ScrollView to get easy and smooth scrolling to select players.
Follow the steps mentioned bellow to integrate ScrollView in your Unity project.
Step 2 Create Object
Create a blank object, say ScrollViewPanel. Drop ScrollViewScript on it.
Step 3 Ceate Child Object
Create 2 blank child objects:
- ObjectsParent, which will have all scrolling objects.
- PositionIndicatorParent, which will have indicators for total objects with currently selected object.
Step 4 Add to Child
Add objects to ObjectsParent as child on which you want scroll view (without concern of objects X position – as it will be arranged later on).
Step 5 Object Indicator Prefab
Follow these steps to create object indicator prefab:
- Create blank object, say indicator.
- Drop SpriteRenderer on it.
- Drag and drop this indicator object to your Prefabs folder and delete from hierarchy.
We have created base of our ScrollView till now.
The hierarchy view is something like this:
Step 6 Apply To Script
Now select ScrollViewPanel. Drop all required objects to ScrollViewScript and assign values to variables.
1. Drop ObjectsParent to ObjectsParent.
2. Drop PositionIndicatoParent to PositionIndicatorsParent.
3. Drop Indicator prefab to IndicatorPrefabRef.
4. Drop blank sprite and selected sprite indicator to BlankSpriteRef and SelectedSpriteRef, to be used to display current selection.
5. Specify total number of objects to size of Objects, in Inspector of ScrollViewScript. Drop all object to Objects in the same sequence you want to display to user.
6. Specify MinDistanceToScorll - this is the minimum distance user needs to move to shift current selected object.
7. Specify DistanceBetweenIndicators - the distance to be displayed between two indicators.
8. Specify TwoObjectsDistance - the distance to be maintained between two main objects.
Step 7 Scripting
ScrollViewScript - For detailed description check specified step numbers at the end of script.
ScrollViewScript:
using UnityEngine;
using System.Collections;
// Following collection is for List<>
using System.Collections.Generic;
public class ScrollViewScript : MonoBehaviour
{
// Public variable declaration section
public List objects;
public Transform objectsParent, positionIndicatorsParent;
public float minDistanceToScroll = 0, distanceBetweenIndicators = 1,
twoObjectsDistance = 0;
public GameObject indicatorPrefabRef;
public Sprite blankSpriteRef, SelectedSpriteRef;
// Private variable declaration section
private float[] positions;
private GameObject[] indicators;
private Vector3 targetPosition, positionDiff;
private int maxScreens, currentScreenNum;
private bool moving = false, performLerp = false;
private float currentPosition, startingPosition;
void Start()
{
initializeScrollView();
}
void initializeScrollView()
{
initializeVariables();
arrangeObjectsAndInitializePositions();
instantiatePositionIndicator();
refreshCurrentScreenIndicator();
}
// Initialize all variables
void initializeVariables()
{
moving = false;
currentScreenNum = 1;
maxScreens = objects.Count;
positions = new float[maxScreens];
}
// [step 6.1]
void arrangeObjectsAndInitializePositions()
{
currentPosition = 0;
int counter = 0;
foreach (Transform currentTransform in objects)
{
currentTransform.localPosition = new Vector3 (currentPosition,
currentTransform.position.y, currentTransform.position.z);
positions[counter] = -currentPosition;
currentPosition += twoObjectsDistance;
counter++;
}
}
// [step 6.2]
void instantiatePositionIndicator()
{
float pos = maxScreens * distanceBetweenIndicators;
float startingPosition = 0;
startingPosition -= (maxScreens%2 == 0) ? pos/2 :
((pos - distanceBetweenIndicators)/2);
indicators = new GameObject[maxScreens];
for (int i = 0; i < maxScreens; i++)
{
GameObject indicator = (GameObject)Instantiate
(indicatorPrefabRef);
indicator.transform.parent = positionIndicatorsParent;
indicator.transform.position = new Vector3 (startingPosition, indicator.transform.position.y, indicator.transform.position.z);
startingPosition += distanceBetweenIndicators;
indicators[i] = indicator;
}
}
// [step 6.3]
void refreshCurrentScreenIndicator ()
{
for (int i = 0; i < maxScreens; i++)
{
if (i + 1 == currentScreenNum) indicators[i].GetComponent().sprite =
SelectedSpriteRef;
else
indicators[i].GetComponent().sprite = blankSpriteRef;
}
}
// [6.4]
void Update ()
{
if (Input.GetMouseButtonDown (0))
{
targetPosition = objectsParent.position;
positionDiff = Camera.main.ScreenToWorldPoint
(Input.mousePosition) - objectsParent.position;
moving = true;
startingPosition = Camera.main.ScreenToWorldPoint (Input.mousePosition).x;
}
else if (Input.GetMouseButtonUp (0))
{
if (moving)
{
performLerp = true;
setEndPosition();
}
moving = false;
}
if (moving && GetTouchState ())
{
targetPosition.x = Camera.main.ScreenToWorldPoint
(Input.mousePosition).x - positionDiff.x;
objectsParent.position = targetPosition;
}
else if (performLerp)
performLerpToTargetPosition();
}
// [6.5]
private void setEndPosition()
{
if ((startingPosition - Camera.main.ScreenToWorldPoint
(Input.mousePosition).x) > minDistanceToScroll)
{
if (currentScreenNum < maxScreens)
currentScreenNum ++;
}
else if ((startingPosition - Camera.main.ScreenToWorldPoint
(Input.mousePosition).x) < -minDistanceToScroll) { if (currentScreenNum > 1)
currentScreenNum --;
}
refreshCurrentScreenIndicator();
targetPosition = objectsParent.position;
targetPosition.x = positions [currentScreenNum - 1];
}
private void performLerpToTargetPosition()
{
positionDiff = objectsParent.position;
positionDiff.x = Mathf.Lerp (objectsParent.position.x,
targetPosition.x, 0.1f);
objectsParent.position = positionDiff;
if (Mathf.Abs (objectsParent.position.x - targetPosition.x) < 0.1f) { performLerp = false; objectsParent.position = targetPosition; } } private bool GetTouchState() { if(Input.touchCount>0 && Input.touchCount<2)
{
Touch touch = Input.GetTouch(0);
if(touch.phase == TouchPhase.Began)
return true;
else if(touch.phase == TouchPhase.Moved)
return true;
else if(touch.phase == TouchPhase.Stationary)
return true;
else if(touch.phase == TouchPhase.Canceled)
return false;
else if(touch.phase == TouchPhase.Ended)
return false;
}
else
{
#if UNITY_EDITOR || UNITY_STANDALONE_WIN || UNITY_STANDALONE_OSX || UNITY_METRO
if (Input.GetMouseButtonDown(0))
return true;
if (Input.GetMouseButton(0))
return true;
else if (Input.GetMouseButtonUp(0))
return false;
#endif
}
return false;
}
}
Step 8 Description
8.1 Arrange all main objects at given distance specified in twoObjectDistance in inspector and initializes positions array to be used in shifting of objects.
8.2 Instantiates indicator objects as per total objects inside objects list and arrange to its proper positions.
8.3 Refreshes and indicates current selected object.
8.4 Detects and manages touch states and according actions,
i.e.
- On touch down - store touch position and sets moving state.
- On touch move - move objectParent with touch.
- On touch up - arrange object at its center position and refreshes indicator.
8.5 Decides auto shifting directions and sets current object to center.
I hope you find this blog very helpful. Please feel free to contact me if you face any issues or have any questions regarding Unity ScrollView Script project comment here and we will help you ASAP.
Got an Idea of Game Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Game Development Company in India.
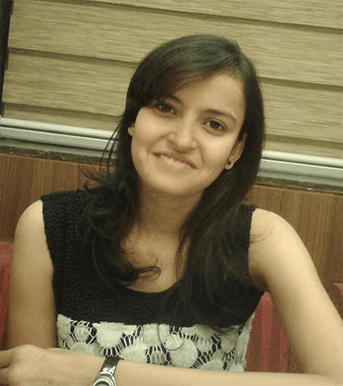
I am skilled game developer, working in iOS and Unity3D game development since 1.5 years and loving this. Perfection and optimization is what I am focused on.
Implementing Facebook SDK for Unity Project
Create Pixel Art in Photoshop