
Be patient..... we are fetching your source code.
Objective
The main objective of the blog post is to give idea about how to create and use delegates in unity.
You will get final output:
Step 1 Delegate
1.1 Concept
Delegates are somewhat reference type of variables, used to hold the reference of methods having the same signature as that of the declared delegate. Delegate reference can be changed at runtime. When called, it notifies all the methods, which are being referenced by it.
1.2 Application
You can use delegates in scripting when:
- Want to invoke multiple methods on single event at runtime.
- Used to trigger callback methods.
1.3 Types
Basically there are two types of delegates:
- Single Delegate
- Multicast Delegate
1.4 Syntax
To declare delegates in unity C#:
//delegate (<Parameters_list>)
public delegate void ButtonClick();
The Parameters_list is optional.
Step 2 Events
2.1 Concept
Events are a type of special delegates which are used when you want to notify other classes when something happens. Like, on button click, Coin collected, on level completion. Any class interested in that event can subscribe it and can call it when that particular event occurs. Events can be declared using Delegates.
2.2 Syntax
To declare event in unity C#: -
//delegate()
public delegate void ButtonClick();
//event
public static event ButtonClick Click;
Step 3 Example
To change the color and position of the ball at runtime, click on Play button.
3.1 DelegateHandler.cs Script
Create an empty gameobject and apply the script called DelegateHandler.cs
using UnityEngine;
using System.Collections;
public class DelegateHandler : MonoBehaviour {
//delegate ()
public delegate void ButtonClick();
//event
public static event ButtonClick Click;
//On Button Click the event named Click can be called
public void OnButtonClick()
{
//call the event
Click ();
}
}
3.2 ColorAndMovement.cs Script
using UnityEngine;
using System.Collections;
using UnityEngine.UI;
public class ColorAndMovement : MonoBehaviour {
public Text textposition,textcolor;
//add the methods to events
void OnEnable () {
DelegateHandler.Click += ChangePosition;
DelegateHandler.Click += ChangeColor;
}
// Update is called once per frame
void Update () {
}
//move the ball to right
void ChangePosition()
{
textposition.text = "Position Changed";
transform.position = new Vector2 (transform.position.x + 1, transform.position.y);
}
//change the color of ball and text
void ChangeColor()
{
Color color=new Color (Random.value, Random.value, Random.value);
textcolor.text = "Color Changed";
//set the color on ball and text
transform.renderer.material.color = color;
textcolor.color=color;
}
//remove the methods added before
void OnDisable()
{
DelegateHandler.Click -= ChangePosition;
DelegateHandler.Click -= ChangeColor;
}
}
I hope you find this blog is very helpful while using Delegates and events in Unity. Let me know in comment if you have any questions regarding in Unity. I will reply you ASAP
Got an Idea of Game Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Game Development Company in India.
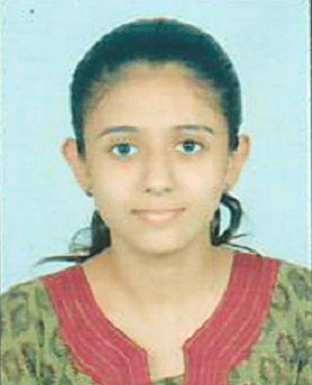
I am a game developer trying to explore my knowledge by implementing new concepts so that games can be made more creative and innovative.
Share Extension in iOS 8
Notification in iOS