
Be patient..... we are fetching your source code.
Objective
Main objective of this tutorial is to create Notification Alerts with the use of NotificationManager. Notification is located at Title bar of the activity when notification is arrives.
Step 1 Introduction
A simple action bar of the activity displays Notification message and icon. This simple Notification in our Activity is used to notify that the message is arrives. To add this simple Notification in our application, we need to create a particular notification. To support notification above API level 4 we use android supportV4 notification compact.
In Notification setTicker() Method is used to show Message like Popup when notification arrives. We can set notification icon using setSmallIcon() method. Pending intent is used for open activity on notification click. We can set number of notification using setNumber() Method.
Following are the steps to add Notification:
Step 2 Display Notification
Add following code in Activity to Display notification.
NotificationCompat.Builder nBuilder = new NotificationCompat.Builder(this);
nBuilder.setContentTitle("Notification");
nBuilder.setContentText("You have received a new Notification");
nBuilder.setTicker("New Message");
nBuilder.setAutoCancel(true);
nBuilder.setSmallIcon(R.drawable.ic_tag_logo);
nBuilder.setNumber(++totalMessages);
Intent intent = new Intent(this, NotificationClass.class);
TaskStackBuilder stackBuilder = TaskStackBuilder.create(this);
stackBuilder.addParentStack(NotificationClass.class);
stackBuilder.addNextIntent(intent);
PendingIntent pendingIntent = stackBuilder.getPendingIntent(0,
PendingIntent.FLAG_UPDATE_CURRENT);
nBuilder.setContentIntent(pendingIntent);
mNotificationManager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
mNotificationManager.notify(notificationID, nBuilder.build());
For more information about NotificationCompat please refer following link:
Step 3 Update Notification
Add following code in Activity to Update Notification.
NotificationCompat.Builder mBuilder = new NotificationCompat.Builder(this);
mBuilder.setContentTitle("Updated Notification");
mBuilder.setContentText("You've got updated Notification.");
mBuilder.setTicker("Updated Notification Alert!");
mBuilder.setAutoCancel(true);
mBuilder.setSmallIcon(R.drawable.ic_tag_logo);
mBuilder.setNumber(++totalMessages);
Intent resultIntent = new Intent(this, NotificationClass.class);
TaskStackBuilder stackBuilder = TaskStackBuilder.create(this);
stackBuilder.addParentStack(NotificationClass.class);
stackBuilder.addNextIntent(resultIntent);
PendingIntent resultPendingIntent = stackBuilder.getPendingIntent(0,
PendingIntent.FLAG_UPDATE_CURRENT);
mBuilder.setContentIntent(resultPendingIntent);
mNotificationManager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
mNotificationManager.notify(notificationID, mBuilder.build());
Step 4 InBox Style Notification
Add following code in Activity to Set InBox Style Notification.
NotificationCompat.Builder mBuilder = new NotificationCompat.Builder(this);
mBuilder.setContentTitle("New Message");
mBuilder.setContentText("You've received new message.");
mBuilder.setTicker("New Message Alert!");
mBuilder.setAutoCancel(true);
mBuilder.setSmallIcon(R.drawable.ic_tag_logo);
mBuilder.setNumber(++totalMessages);
NotificationCompat.InboxStyle inboxStyle = new NotificationCompat.InboxStyle();
String[] notificationArray = new String[6];
notificationArray[0] = new String("First Notification...");
notificationArray[1] = new String("Second Notification...");
notificationArray[2] = new String("Third Notification ....");
notificationArray[3] = new String("Fourth Notification.....");
notificationArray[4] = new String("Fifth Notification....");
notificationArray[5] = new String("Sixth Notification....");
inboxStyle.setBigContentTitle("Notification Details.");
for (int i = 0; i < notificationArray.length; i++) {
inboxStyle.addLine(notificationArray[i]);
}
mBuilder.setStyle(inboxStyle);
Intent resultIntent = new Intent(this, NotificationClass.class);
TaskStackBuilder stackBuilder = TaskStackBuilder.create(this);
stackBuilder.addParentStack(NotificationClass.class);
stackBuilder.addNextIntent(resultIntent);
PendingIntent resultPendingIntent = stackBuilder.getPendingIntent(0,
PendingIntent.FLAG_UPDATE_CURRENT);
mBuilder.setContentIntent(resultPendingIntent);
mNotificationManager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
mNotificationManager.notify(notificationID, mBuilder.build());
Step 5 Cancel Notification
Add following code in Activity to Cancel Notification.
if (mNotificationManager != null) {
mNotificationManager.cancel(notificationID);
}
Step 6 activity_main.xml file
Add following code in activity_main.xml File.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<Button
android:id="@+id/start"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/start_note" />
<Button
android:id="@+id/cancel"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/cancel_note" />
<Button
android:id="@+id/update"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/update_note" />
<Button
android:id="@+id/bigNotification"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/big_notification" />
</LinearLayout>
Step 7 notif.xml
Create another XML File with name notif.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<TextView
android:layout_width="fill_parent"
android:layout_height="400dp"
android:text="Hi, Your Detailed notification view goes here...." />
</LinearLayout>
Screenshots:
I hope you found this blog helpful while Create Notification Alert using NotificationManager. Let me know if you have any questions or concerns regarding Android Application Development, please put a comment here and we will get back to you ASAP.
Got an Idea of Android App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Android App Development Company in India.
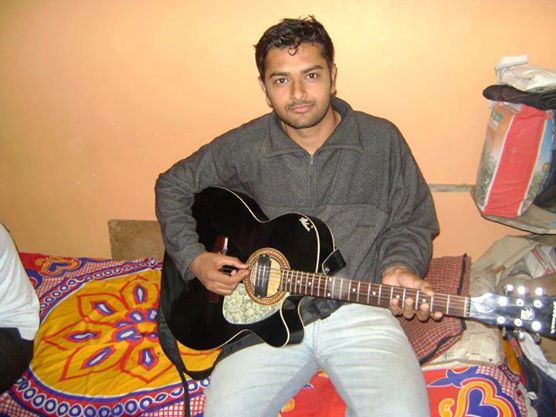
I am professional Android application developer with experience of 1 years in Android Application Development. I worked with so many technology but android is the only one which interests me.