
Be patient..... we are fetching your source code.
Objective
Main objective of this post is to give you an idea about XML Parsing using NSXMLParse in Swift
Introduction:
In this Swift XML Parser tutorial, we will create simple application called XMLParsingDemo, which describes how to parse XML data. XML stands for Extensible Markup Language. XML is a meta language which is used to define own customized markup language.
For this XML parsing main class is NSXMLParse class, which is the XML Parser and inbuilt class of iPhone SDK. NSXMLParser is SAX Parser in which it calls the parser delegate method base on the data of XML document.
Following are the steps, which explains how to fetch and read XML data and display it in tableview.
Step 1 Create Single View Application
First in our XML Parser Swift example, open Xcode and create one new project with single view application template as shown in below figure.
In the next step, as my tutorial is regarding Swift XML Parsing demo I am entering XMLParsingDemo as a product name and also select swift as a language as shown in below figure.
You can get more detail about creating XCode project from following link:
Step 2 Design User Interface
To design user interface open Main.storyboard file and in that one ViewController is there as shown in below figure. In that ViewController add one tableview to display data.
You can get more detail about UITableView from following link:
Step 3 Implementation of XML Parsing
To parse XML Data declare following variable in your class.
var parser = NSXMLParser()
var posts = NSMutableArray()
var elements = NSMutableDictionary()
var element = NSString()
var title1 = NSMutableString()
var date = NSMutableString()
Here parser is the object, which is used to download and parse the xml file. Post is a object of mutable array used to store feed data. Element is a mutabledictionary which contains the data of feed like title and date separately. title1 and date used to store string data of feed.
Now from viewDidLoad method calling beginParsing method in that doing initialization of parser object, set NSXMLParserDelegate and then start XML parsing.
func beginParsing()
{
posts = []
parser = NSXMLParser(contentsOfURL:(NSURL(string:"http://images.apple.com/main/rss/hotnews/hotnews.rss"))!)!
parser.delegate = self
parser.parse()
tbData!.reloadData()
}
Step 4 Implementation of Parser Delegate
To implement the parser delegate method have to set delegate in class as shown below. It informs the compiler that ViewController class implements the NSXMLParserDelegate.
class ViewController: UIViewController, NSXMLParserDelegate
During parsing, when parser finds any new element it calls the below delegate method. In this method allocate variable when parser find the item element.
func parser(parser: NSXMLParser, didStartElement elementName: String, namespaceURI: String?, qualifiedName qName: String?, attributes attributeDict: [String : String])
{
element = elementName
if (elementName as NSString).isEqualToString("item")
{
elements = NSMutableDictionary()
elements = [:]
title1 = NSMutableString()
title1 = ""
date = NSMutableString()
date = ""
}
}
After that when it finds new character it calls the below delegate method. In this method append all character in mutable string for particular element.
func parser(parser: NSXMLParser!, foundCharacters string: String!)
{
if element.isEqualToString("title") {
title1.appendString(string)
} else if element.isEqualToString("pubDate") {
date.appendString(string)
}
}
When parser finds the end of element it calls the below delegate method. In that just store the feed data in dictionary and then add that dictionary in array.
func parser(parser: NSXMLParser!, didEndElement elementName: String!, namespaceURI: String!, qualifiedName qName: String!)
{
if (elementName as NSString).isEqualToString("item") {
if !title1.isEqual(nil) {
elements.setObject(title1, forKey: "title")
}
if !date.isEqual(nil) {
elements.setObject(date, forKey: "date")
}
posts.addObject(elements)
}
}
Step 5 Display XML data in tableview
After xml parsing display data from array in tableview using below methods.
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int
{
return posts.count
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell
{
var cell : UITableViewCell = tableView.dequeueReusableCellWithIdentifier("Cell")!
if(cell.isEqual(NSNull)) {
cell = NSBundle.mainBundle().loadNibNamed("Cell", owner: self, options: nil)[0] as UITableViewCell;
}
cell.textLabel?.text = posts.objectAtIndex(indexPath.row).valueForKey("title") as NSString
cell.detailTextLabel?.text = posts.objectAtIndex(indexPath.row).valueForKey("date") as NSString
return cell as UITableViewCell
}
OutPut:
I hope you find this blog is very helpful while working with XML Parsing using NSXMLParse in Swift. Let me know in a comment if you have any question regarding Swift I will reply you ASAP.
Got an Idea of iPhone App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best iPhone App Development Company in India.
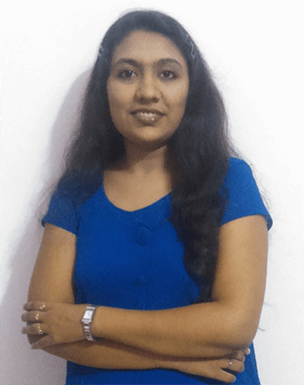
Being an iOS developer with experience of 3 years feels pretty awesome because much more than just playing around with code, software developers are the brains behind the design, installation, testing and maintenance of software systems.