Objective
This blog post is about how to drag and drop any game object. We can simply use the mouse position to get the target object without using the rigid body component.
Step 1 Create Project
Create a new unity 3D project and set the environment as shown below. Take an empty game object and name it as DragAndDrop and create some other game objects like sphere, cube etc.
Step 2 Create Script
Make C# script and name it as GameobjectDragAndDrop.
Step 3 Click Detection
First of all you need to enter the code required to detect the gameobject when clicked on it.
Use the following method for it:
GameObject ReturnClickedObject(out RaycastHit hit)
{
GameObject target = null;
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
if (Physics.Raycast(ray.origin, ray.direction * 10, out hit))
{
target = hit.collider.gameObject;
}
return target;
}
Step 4 Update Method
Write the following code in Update method:
void Update()
{
if (Input.GetMouseButtonDown(0))
{
RaycastHit hitInfo;
target = ReturnClickedObject(out hitInfo);
if (target != null)
{
isMouseDrag = true;
Debug.Log("target position :" + target.transform.position);
//Convert world position to screen position.
screenPosition = Camera.main.WorldToScreenPoint(target.transform.position);
offset = target.transform.position - Camera.main.ScreenToWorldPoint(new Vector3(Input.mousePosition.x, Input.mousePosition.y, screenPosition.z));
}
}
if (Input.GetMouseButtonUp(0))
{
isMouseDrag = false;
}
if (isMouseDrag)
{
//track mouse position.
Vector3 currentScreenSpace = new Vector3(Input.mousePosition.x, Input.mousePosition.y, screenPosition.z);
//convert screen position to world position with offset changes.
Vector3 currentPosition = Camera.main.ScreenToWorldPoint(currentScreenSpace) + offset;
//It will update target gameobject's current postion.
target.transform.position = currentPosition;
}
}
Step 5 Apply Script
Now, apply this script onto DragAndDrop GameObject.
Step 6 Testing
Run the program and click on any object that you want to drag and drop.
It's Done.
I hope you find this blog post very helpful while working with Drag and Drop any Game object in Unity. Let me know in comment if you have any question regarding Game Object. I will reply you ASAP.
Got an Idea of Game Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Game Development Company in India.
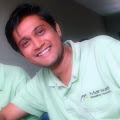
I am professional game developer, developing games in unity (for all platforms). I am very passionate about game development and aim to create addictive, interactive, high quality games.