
Be patient..... we are fetching your source code.
Objective
Main objective of this blog post is to help you to integrate Instagram in Android App
You will get Final Output:
Instagram has captured lot of eyeballs after it got acquire by Facebook, and Instagram is also growing its user base and getting more popular day by day. So, integrating Instagram sharing in your app can give you lot of leverage. For easy integration, Instagram provides quite rich API.
The API can help you in following kind of operations:
- To get all Media files uploaded by user on Instagram.
- To view like and comments for the media file.
- To get followers and following information.
- To get user information etc.
Instagram does not provide:
- API to upload image.
- To add follower.
- To follow someone etc.
It provides read only API, using which we can view all information.
I am creating an app in which I am fetching all the Instagram photos of a user and also getting the followers and followings list.
Following are the steps to Integrate Instagram in your Android application
Step 1 Register your Application
To Register the app follow the below link. After open the page click on Register your Application
Step 2 Register new Client ID
Fill in the app information After clicking on Register your Application you will be redirected to Client ID page. Enter all the information of your app and then click on register.
Step 3 Manage Clients
Manage Clients page will be displayed after registration done successfully.
To Integrate Instagram in our app, we will need 3 things
- Client id
- Client secret
- Redirect Uri
Copy client id, client secret and redirect Uri and paste it in ApplicationData class as following:
public static final String CLIENT_ID = "your client id";
public static final String CLIENT_SECRET = "your client secret";
public static final String CALLBACK_URL = "redirect uri here";
Step 4 Creating InstagramDialog Class
Create InstagramDialog class to display webview for login into Instagram as following:
public class InstagramDialog extends Dialog {
static final float[] DIMENSIONS_LANDSCAPE = { 460, 260 };
static final float[] DIMENSIONS_PORTRAIT = { 280, 420 };
static final FrameLayout.LayoutParams FILL = new FrameLayout.LayoutParams(
ViewGroup.LayoutParams.FILL_PARENT,
ViewGroup.LayoutParams.FILL_PARENT);
static final int MARGIN = 4;
static final int PADDING = 2;
private String mUrl;
private OAuthDialogListener mListener;
private ProgressDialog mSpinner;
private WebView mWebView;
private LinearLayout mContent;
private TextView mTitle;
private static final String TAG = "Instagram-WebView";
public InstagramDialog(Context context, String url,
OAuthDialogListener listener) {
super(context);
mUrl = url;
mListener = listener;
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
mSpinner = new ProgressDialog(getContext());
mSpinner.requestWindowFeature(Window.FEATURE_NO_TITLE);
mSpinner.setMessage("Loading...");
mContent = new LinearLayout(getContext());
mContent.setOrientation(LinearLayout.VERTICAL);
setUpTitle();
setUpWebView();
Display display = getWindow().getWindowManager().getDefaultDisplay();
final float scale = getContext().getResources().getDisplayMetrics().density;
float[] dimensions = (display.getWidth() < display.getHeight()) ? DIMENSIONS_PORTRAIT : DIMENSIONS_LANDSCAPE; addContentView(mContent, new FrameLayout.LayoutParams( (int) (dimensions[0] * scale + 0.5f), (int) (dimensions[1] * scale + 0.5f))); CookieSyncManager.createInstance(getContext()); CookieManager cookieManager = CookieManager.getInstance(); cookieManager.removeAllCookie(); } private void setUpTitle() { requestWindowFeature(Window.FEATURE_NO_TITLE); mTitle = new TextView(getContext()); mTitle.setText("Instagram"); mTitle.setTextColor(Color.WHITE); mTitle.setTypeface(Typeface.DEFAULT_BOLD); mTitle.setBackgroundColor(Color.BLACK); mTitle.setPadding(MARGIN + PADDING, MARGIN, MARGIN, MARGIN); mContent.addView(mTitle); } private void setUpWebView() { mWebView = new WebView(getContext()); mWebView.setVerticalScrollBarEnabled(false); mWebView.setHorizontalScrollBarEnabled(false); mWebView.setWebViewClient(new OAuthWebViewClient()); mWebView.getSettings().setJavaScriptEnabled(true); mWebView.loadUrl(mUrl); mWebView.setLayoutParams(FILL); mContent.addView(mWebView); } private class OAuthWebViewClient extends WebViewClient { @Override public boolean shouldOverrideUrlLoading(WebView view, String url) { Log.d(TAG, "Redirecting URL " + url); if (url.startsWith(InstagramApp.mCallbackUrl)) { String urls[] = url.split("="); mListener.onComplete(urls[1]); InstagramDialog.this.dismiss(); return true; } return false; } @Override public void onReceivedError(WebView view, int errorCode, String description, String failingUrl) { Log.d(TAG, "Page error: " + description); super.onReceivedError(view, errorCode, description, failingUrl); mListener.onError(description); InstagramDialog.this.dismiss(); } @Override public void onPageStarted(WebView view, String url, Bitmap favicon) { Log.d(TAG, "Loading URL: " + url); super.onPageStarted(view, url, favicon); mSpinner.show(); } @Override public void onPageFinished(WebView view, String url) { super.onPageFinished(view, url); String title = mWebView.getTitle(); if (title != null && title.length() > 0) {
mTitle.setText(title);
}
Log.d(TAG, "onPageFinished URL: " + url);
mSpinner.dismiss();
}
}
public interface OAuthDialogListener {
public abstract void onComplete(String accessToken);
public abstract void onError(String error);
}
}
Step 5 Create InstagramApp Class
Create InstagramApp class which is used to store Instagram user session information by calling API as following:
public class InstagramApp {
private InstagramSession mSession;
private InstagramDialog mDialog;
private OAuthAuthenticationListener mListener;
private ProgressDialog mProgress;
private String mAuthUrl;
private String mTokenUrl;
private String mAccessToken;
private Context mCtx;
private String mClientId;
private String mClientSecret;
private static int WHAT_FINALIZE = 0;
private static int WHAT_ERROR = 1;
private static int WHAT_FETCH_INFO = 2;
/**
* Callback url, as set in 'Manage OAuth Costumers' page
* (https://developer.github.com/)
*/
public static String mCallbackUrl = "";
private static final String AUTH_URL = "https://api.instagram.com/oauth/authorize/";
private static final String TOKEN_URL = "https://api.instagram.com/oauth/access_token";
private static final String API_URL = "https://api.instagram.com/v1";
private static final String TAG = "InstagramAPI";
public InstagramApp(Context context, String clientId, String clientSecret,
String callbackUrl) {
mClientId = clientId;
mClientSecret = clientSecret;
mCtx = context;
mSession = new InstagramSession(context);
mAccessToken = mSession.getAccessToken();
mCallbackUrl = callbackUrl;
mTokenUrl = TOKEN_URL + "?client_id=" + clientId + "&client_secret="
+ clientSecret + "&redirect_uri=" + mCallbackUrl + "&grant_type=authorization_code";
mAuthUrl = AUTH_URL + "?client_id=" + clientId + "&redirect_uri="
+ mCallbackUrl + "&response_type=code&display=touch&scope=likes+comments+relationships";
OAuthDialogListener listener = new OAuthDialogListener() {
@Override
public void onComplete(String code) {
getAccessToken(code);
}
@Override
public void onError(String error) {
mListener.onFail("Authorization failed");
}
};
mDialog = new InstagramDialog(context, mAuthUrl, listener);
mProgress = new ProgressDialog(context);
mProgress.setCancelable(false);
}
private void getAccessToken(final String code) {
mProgress.setMessage("Getting access token ...");
mProgress.show();
new Thread() {
@Override
public void run() {
Log.i(TAG, "Getting access token");
int what = WHAT_FETCH_INFO;
try {
URL url = new URL(TOKEN_URL);
//URL url = new URL(mTokenUrl + "&code=" + code);
Log.i(TAG, "Opening Token URL " + url.toString());
HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection();
urlConnection.setRequestMethod("POST");
urlConnection.setDoInput(true);
urlConnection.setDoOutput(true);
//urlConnection.connect();
OutputStreamWriter writer = new OutputStreamWriter(urlConnection.getOutputStream());
writer.write("client_id="+mClientId+
"&client_secret="+mClientSecret+
"&grant_type=authorization_code" +
"&redirect_uri="+mCallbackUrl+
"&code=" + code);
writer.flush();
String response = streamToString(urlConnection.getInputStream());
Log.i(TAG, "response " + response);
JSONObject jsonObj = (JSONObject) new JSONTokener(response).nextValue();
mAccessToken = jsonObj.getString("access_token");
Log.i(TAG, "Got access token: " + mAccessToken);
String id = jsonObj.getJSONObject("user").getString("id");
String user = jsonObj.getJSONObject("user").getString("username");
String name = jsonObj.getJSONObject("user").getString("full_name");
mSession.storeAccessToken(mAccessToken, id, user, name);
} catch (Exception ex) {
what = WHAT_ERROR;
ex.printStackTrace();
}
mHandler.sendMessage(mHandler.obtainMessage(what, 1, 0));
}
}.start();
}
private void fetchUserName() {
mProgress.setMessage("Finalizing ...");
new Thread() {
@Override
public void run() {
Log.i(TAG, "Fetching user info");
int what = WHAT_FINALIZE;
try {
URL url = new URL(API_URL + "/users/" + mSession.getId() + "/?access_token=" + mAccessToken);
Log.d(TAG, "Opening URL " + url.toString());
HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection();
urlConnection.setRequestMethod("GET");
urlConnection.setDoInput(true);
urlConnection.connect();
String response = streamToString(urlConnection.getInputStream());
System.out.println(response);
JSONObject jsonObj = (JSONObject) new JSONTokener(response).nextValue();
String name = jsonObj.getJSONObject("data").getString("full_name");
String bio = jsonObj.getJSONObject("data").getString("bio");
Log.i(TAG, "Got name: " + name + ", bio [" + bio + "]");
} catch (Exception ex) {
what = WHAT_ERROR;
ex.printStackTrace();
}
mHandler.sendMessage(mHandler.obtainMessage(what, 2, 0));
}
}.start();
}
private Handler mHandler = new Handler() {
@Override
public void handleMessage(Message msg) {
if (msg.what == WHAT_ERROR) {
mProgress.dismiss();
if(msg.arg1 == 1) {
mListener.onFail("Failed to get access token");
}
else if(msg.arg1 == 2) {
mListener.onFail("Failed to get user information");
}
}
else if(msg.what == WHAT_FETCH_INFO) {
fetchUserName();
}
else {
mProgress.dismiss();
mListener.onSuccess();
}
}
};
public boolean hasAccessToken() {
return (mAccessToken == null) ? false : true;
}
public void setListener(OAuthAuthenticationListener listener) {
mListener = listener;
}
public String getUserName() {
return mSession.getUsername();
}
public String getId() {
return mSession.getId();
}
public String getName() {
return mSession.getName();
}
public void authorize() {
//Intent webAuthIntent = new Intent(Intent.ACTION_VIEW);
//webAuthIntent.setData(Uri.parse(AUTH_URL));
//mCtx.startActivity(webAuthIntent);
mDialog.show();
}
private String streamToString(InputStream is) throws IOException {
String str = "";
if (is != null) {
StringBuilder sb = new StringBuilder();
String line;
try {
BufferedReader reader = new BufferedReader(
new InputStreamReader(is));
while ((line = reader.readLine()) != null) {
sb.append(line);
}
reader.close();
} finally {
is.close();
}
str = sb.toString();
}
return str;
}
public void resetAccessToken() {
if (mAccessToken != null) {
mSession.resetAccessToken();
mAccessToken = null;
}
}
public interface OAuthAuthenticationListener {
public abstract void onSuccess();
public abstract void onFail(String error);
}
}
In the above code getAccessToken() method uses.
https://api.instagram.com/oauth/access_token API with post method to get user id, username etc. and stores it to session using InstagramSession class object.
Step 6 Create InstagramSession Class
InstagramSession class is used to store user info as following:
public class InstagramSession {
private SharedPreferences sharedPref;
private Editor editor;
private static final String SHARED = "Instagram_Preferences";
private static final String API_USERNAME = "username";
private static final String API_ID = "id";
private static final String API_NAME = "name";
private static final String API_ACCESS_TOKEN = "access_token";
public InstagramSession(Context context) {
sharedPref = context.getSharedPreferences(SHARED, Context.MODE_PRIVATE);
editor = sharedPref.edit();
}
/**
*
* @param accessToken
* @param expireToken
* @param expiresIn
* @param username
*/
public void storeAccessToken(String accessToken, String id, String username, String name) {
editor.putString(API_ID, id);
editor.putString(API_NAME, name);
editor.putString(API_ACCESS_TOKEN, accessToken);
editor.putString(API_USERNAME, username);
editor.commit();
}
public void storeAccessToken(String accessToken) {
editor.putString(API_ACCESS_TOKEN, accessToken);
editor.commit();
}
/**
* Reset access token and user name
*/
public void resetAccessToken() {
editor.putString(API_ID, null);
editor.putString(API_NAME, null);
editor.putString(API_ACCESS_TOKEN, null);
editor.putString(API_USERNAME, null);
editor.commit();
}
/**
* Get user name
*
* @return User name
*/
public String getUsername() {
return sharedPref.getString(API_USERNAME, null);
}
/**
*
* @return
*/
public String getId() {
return sharedPref.getString(API_ID, null);
}
/**
*
* @return
*/
public String getName() {
return sharedPref.getString(API_NAME, null);
}
/**
* Get access token
*
* @return Access token
*/
public String getAccessToken() {
return sharedPref.getString(API_ACCESS_TOKEN, null);
}
}
Step 7 Fetching User Data
After creating above classes in MainActivity, create object of InstagramApp and add set
OAuthAuthenticationListener() to that object as following in onCreate() method :
mApp = new InstagramApp(this, ApplicationData.CLIENT_ID,
ApplicationData.CLIENT_SECRET, ApplicationData.CALLBACK_URL);
mApp.setListener(new OAuthAuthenticationListener() {
@Override
public void onSuccess() {
// tvSummary.setText("Connected as " + mApp.getUserName());
btnConnect.setText("Disconnect");
llAfterLoginView.setVisibility(View.VISIBLE);
// userInfoHashmap = mApp.
mApp.fetchUserName(handler);
}
@Override
public void onFail(String error) {
Toast.makeText(MainActivity.this, error, Toast.LENGTH_SHORT).show();
}
});
In onSuccess() method, I have called another method fetchUserName() of InstagramApp class which calls https://api.instagram.com/v1/users/1574083/?access_token=ACCESS-TOKEN API which is used to get all information such as
- User full name
- User name
- Profile photo path
- Number of photos uploaded by user
- No of following and followers etc.
I have stored this information in Hashmap. On view info button click, all the information will be displayed as alert dialog.
Code for the MainActivity is as following:
private InstagramApp mApp;
private Button btnConnect, btnViewInfo, btnGetAllImages;
private LinearLayout llAfterLoginView;
private HashMap<String, String> userInfoHashmap = new HashMap<String, String>();
private Handler handler = new Handler(new Callback() {
@Override
public boolean handleMessage(Message msg) {
if (msg.what == InstagramApp.WHAT_FINALIZE) {
userInfoHashmap = mApp.getUserInfo();
} else if (msg.what == InstagramApp.WHAT_FINALIZE) {
Toast.makeText(MainActivity.this, "Check your network.",
Toast.LENGTH_SHORT).show();
}
return false;
}
});
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
mApp = new InstagramApp(this, ApplicationData.CLIENT_ID,
ApplicationData.CLIENT_SECRET, ApplicationData.CALLBACK_URL);
mApp.setListener(new OAuthAuthenticationListener() {
@Override
public void onSuccess() {
// tvSummary.setText("Connected as " + mApp.getUserName());
btnConnect.setText("Disconnect");
llAfterLoginView.setVisibility(View.VISIBLE);
// userInfoHashmap = mApp.
mApp.fetchUserName(handler);
}
@Override
public void onFail(String error) {
Toast.makeText(MainActivity.this, error, Toast.LENGTH_SHORT).show();
}
});
setWidgetReference();
bindEventHandlers();
if (mApp.hasAccessToken()) {
// tvSummary.setText("Connected as " + mApp.getUserName());
btnConnect.setText("Disconnect");
llAfterLoginView.setVisibility(View.VISIBLE);
mApp.fetchUserName(handler);
}
}
private void bindEventHandlers() {
btnConnect.setOnClickListener(this);
btnViewInfo.setOnClickListener(this);
btnGetAllImages.setOnClickListener(this);
}
private void setWidgetReference() {
llAfterLoginView = (LinearLayout) findViewById(R.id.llAfterLoginView);
btnConnect = (Button) findViewById(R.id.btnConnect);
btnViewInfo = (Button) findViewById(R.id.btnViewInfo);
btnGetAllImages = (Button) findViewById(R.id.btnGetAllImages);
}
// OAuthAuthenticationListener listener ;
@Override
public void onClick(View v) {
if (v == btnConnect) {
connectOrDisconnectUser();
} else if (v == btnViewInfo) {
displayInfoDialogView();
} else if (v == btnGetAllImages) {
startActivity(new Intent(MainActivity.this, AllMediaFiles.class)
.putExtra("userInfo", userInfoHashmap));
}
}
private void connectOrDisconnectUser() {
if (mApp.hasAccessToken()) {
final AlertDialog.Builder builder = new AlertDialog.Builder(
MainActivity.this);
builder.setMessage("Disconnect from Instagram?")
.setCancelable(false)
.setPositiveButton("Yes",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int id) {
mApp.resetAccessToken();
// btnConnect.setVisibility(View.VISIBLE);
llAfterLoginView.setVisibility(View.GONE);
btnConnect.setText("Connect");
// tvSummary.setText("Not connected");
}
})
.setNegativeButton("No",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int id) {
dialog.cancel();
}
});
final AlertDialog alert = builder.create();
alert.show();
} else {
mApp.authorize();
}
}
private void displayInfoDialogView() {
AlertDialog.Builder alertDialog = new AlertDialog.Builder(
MainActivity.this);
alertDialog.setTitle("Profile Info");
LayoutInflater inflater = (LayoutInflater) getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View view = inflater.inflate(R.layout.profile_view, null);
alertDialog.setView(view);
ImageView ivProfile = (ImageView) view
.findViewById(R.id.ivProfileImage);
TextView tvName = (TextView) view.findViewById(R.id.tvUserName);
TextView tvNoOfFollwers = (TextView) view
.findViewById(R.id.tvNoOfFollowers);
TextView tvNoOfFollowing = (TextView) view
.findViewById(R.id.tvNoOfFollowing);
new ImageLoader(MainActivity.this).DisplayImage(
userInfoHashmap.get(InstagramApp.TAG_PROFILE_PICTURE),
ivProfile);
tvName.setText(userInfoHashmap.get(InstagramApp.TAG_USERNAME));
tvNoOfFollowing.setText(userInfoHashmap.get(InstagramApp.TAG_FOLLOWS));
tvNoOfFollwers.setText(userInfoHashmap
.get(InstagramApp.TAG_FOLLOWED_BY));
alertDialog.create().show();
}
}
I hope you find this Instagram Integration in android tutorial blog helpful. If you are facing any issues or have any questions regarding instagram integration please feel free to comment over here, I would be glad to help you ASAP.
Also check out following Android Integration:
Learning Android sounds fun, right? Why not check out our other Android Tutorials?
Got an Idea of Android App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the Best Android App Development Company in India.
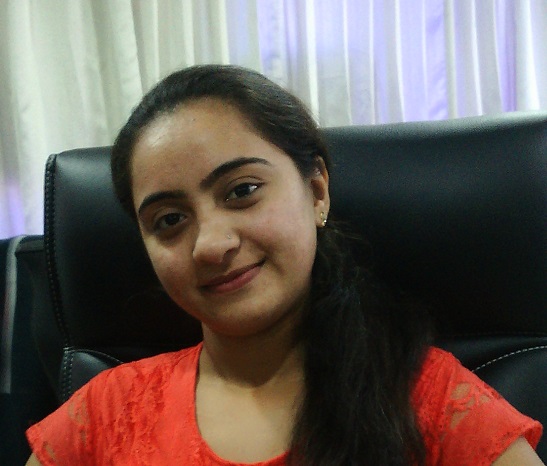
I am android developer, and do development not because to get paid or get adulation by the public, but because it is fun to program. I have been working in this field since one and half year.
Facebook Integration in Android Studio Tutorial