
Be patient..... we are fetching your source code.
Objective
Main objective of this tutorial is to explain how to cut an object and how fake mesh cutter works.
Given example will cut a wood stick from all the points where bombs are placed.
Step 1 Basic Setup
- Place this script on wood object(main object).
- Wood object should have box collider with NO SCALING.
- woodObjectPrefeb is an object which will be instantiated, So woodObjectPrefeb should have same texture as wood.
- The woodObjectPrefeb which will be instantiated should have box collider and rigidbody.
- It is important to place bombs as child of wood object and must be placed only on the wood.
Step 2 Video
Unity - Fake Mesh Cutter For 2D Object
In this video you can see Fake Mesh Cutter for 2D Object in Action.
Step 3 Code Implementation
public class StickScript : MonoBehaviour
{
//object which will be instanciated
public GameObject woodObjectPrefeb;
// bombs are placed as child of wood transform
private GameObject[] bombs;
private float woodColliderSize, storeRotation = 0, startingPoint, endPoint, distance, newScale, radius = 15.0F, power = 2000.0F;
private GameObject tempGameObject;
public void blowClicked()
{
//if there is no bomb to blast
if (transform.childCount == 0)
return;
//initializing bomb array
bombs = new GameObject[transform.childCount];
for (int i = 0; i < transform.childCount; i++)
bombs[i] = transform.GetChild(i).gameObject;
//setting rotation to zero if wood is rotated to any other angle
if (transform.eulerAngles.z != 0)
{
storeRotation = transform.eulerAngles.z;
transform.eulerAngles = Vector3.zero;
}
//bombs array will be arranges in ascending order by their y coordinate.
arrangeBombs();
//fetching size of collider
woodColliderSize = GetComponent().size.y;
//creating object above first bomb.
//new objects will be instantiated as child of wood transform.
createFirstObject();
//creating objects below each bomb.
for (int i = 0; i < bombs.Length; i++)
createObjectBelowBomb(bombs[i], i);
//rotating wood object on its initial rotation.
transform.eulerAngles = new Vector3(0, 0, storeRotation);
//removing wood object's rendering and collider
Destroy(GetComponent() as SpriteRenderer);
Destroy(GetComponent() as BoxCollider);
//new objects will be blasted and bombs will be destroyed.
manageChildren();
}
//bubble sort for arranging bombs array.
private void arrangeBombs()
{
GameObject tempY;
for (int i = 0; i < bombs.Length - 1; i++)
{
for (int j = 0; j < bombs.Length - i - 1; j++)
{
if (bombs[j].transform.position.y < bombs[j + 1].transform.position.y)
{
tempY = bombs[j];
bombs[j] = bombs[j + 1];
bombs[j + 1] = tempY;
}
}
}
}
private void createFirstObject()
{
startingPoint = findStartingPointY(gameObject);
endPoint = findStartingPointY(bombs[0]);
distance = Mathf.Abs(startingPoint - endPoint);
//if distance between two bombs is less than 0.1 object will not be created between them.
if (distance <= 0.1f)
return;
//calculating scale of instantiated object.
newScale = (Mathf.Abs(startingPoint - endPoint)) / woodColliderSize;
tempGameObject = (GameObject)Instantiate(woodObjectPrefeb, new Vector3(transform.position.x, endPoint + (distance / 2), transform.position.z), Quaternion.identity);
tempGameObject.transform.localScale = new Vector3(1, newScale, 1);
//attaching created object as child to wood object.
tempGameObject.transform.parent = bombs[0].transform.parent;
}
//this will work same as create first object.
private void createObjectBelowBomb(GameObject currentBomb, int index)
{
startingPoint = findEndingPointY(currentBomb);
if (index + 1 < bombs.Length)
endPoint = findStartingPointY(bombs[index + 1]);
else
endPoint = findEndingPointY(gameObject);
distance = Mathf.Abs(startingPoint - endPoint);
if (distance <= 0.1f)
return;
newScale = (Mathf.Abs(startingPoint - endPoint)) / woodColliderSize;
tempGameObject = (GameObject)Instantiate(woodObjectPrefeb, new Vector3(transform.position.x, startingPoint - (distance / 2), transform.position.z), Quaternion.identity);
tempGameObject.transform.localScale = new Vector3(1, newScale, 1);
tempGameObject.transform.parent = bombs[0].transform.parent;
}
private void manageChildren()
{
foreach (Transform childTransform in transform)
{
//code for blasting new created objects and destroying bombs
if (childTransform.name.Equals("Bomb"))
{
Collider[] colliders = Physics.OverlapSphere(childTransform.position, radius);
foreach (Collider hit in colliders)
{
if (hit && hit.rigidbody)
{
hit.rigidbody.AddExplosionForce(power, childTransform.position, radius, 3.0F);
}
}
Destroy(childTransform.gameObject);
}
}
}
private float findStartingPointY(GameObject tempObject)
{
return (tempObject.transform.position.y + ((tempObject.GetComponent().size.y) / 2));
}
private float findEndingPointY(GameObject tempObject)
{
return (tempObject.transform.position.y - ((tempObject.GetComponent().size.y) / 2));
}
}
Try the code and implement Fake Mesh Cutter for 2D Object. If you find any query fire them in comment below we will try to solve them out.
Got an Idea of Game Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Game Development Company in India.
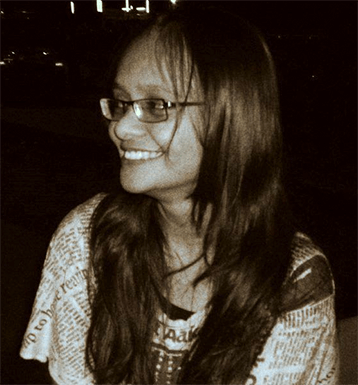
I am game programmer, always had keen interest in games which lead me to learn and develop games. I’ve been developing games for over a year. I develop games using andangine for android and unity3d for all platform compatibility.
Introduction to SQLite Database in iOS
Unity - Rotate object by 90 Degree