
Be patient..... we are fetching your source code.
Objective
Main Objective of this blog post is to give you an idea about how to Access Wi-Fi data in Android.
Final Output:
Step 1 Introduction
Android gives access to applications to view the state of the wireless connections at very low level. Android Application can access almost all the information of a Wi-Fi connection. The information that an application can access includes connected network's Hostname, link speed, IP address, negotiation state, other networks information and Channel.
Applications can also add, save, terminate, scan and initiate Wi-Fi connections.
Step 2 WifiManager
Android provides WifiManager API to manage all aspects of Wi-Fi connectivity. You can instantiate this class by calling the getSystemService() method.
Its syntax is as given below:
private WifiManager mainWifi;
mainWifi = (WifiManager) getSystemService(Context.WIFI_SERVICE);
For more about WifiManager class pleaser refer the following link:
Step 3 BroadcastReciver
In order to scan your list of wireless networks, you also need to register your BroadcastReceiver.
For this make a BroadcastReceiver class as shown below:
class WifiReceiver extends BroadcastReceiver {
public void onReceive(Context c, Intent intent) {
}
}
It can be registered using registerReceiver() method with argument of your receiver class object.
Its syntax is as given below:
receiverWifi = new WifiReceiver();
registerReceiver(receiverWifi, new IntentFilter(WifiManager.SCAN_RESULTS_AVAILABLE_ACTION));
For more about BroadcastReceiver class please refer the following link:
Step 4 Add permission into the AndroidManifest.xml file
Now for the availability of scanning through Wi-Fi, you need to add required permission in your AndroidManifest.xml file.
<uses-permissionandroid:name="android.permission.ACCESS_NETWORK_STATE"/>
<uses-permissionandroid:name="android.permission.ACCESS_WIFI_STATE"/>
<uses-permissionandroid:name="android.permission.CHANGE_WIFI_STATE"/>
Step 5 startScan() Method
Wi-Fi scan can be started by calling the startScan() method of the WifiManager class. This method returns a list of the ScanResult objects. We can access any object by calling the get() method of the list.
Its syntax is as given below:
mainWifi.startScan();
ListwifiList = mainWifi.getScanResults();
Now we need to get our required information of Wi-Fi from the available wifilist. It stores a lot of information of wifi like SSID (name of WIFI), Strength, Frequency, Capability etc… We can get all this information through get() method.
Its syntax is given below:
holder.tvDetails.setText("SSID :: " + wifiList.get(0).SSID
+ "\nStrength :: " + wifiList.get(0).level
+ "\nBSSID :: " + wifiList.get(0).BSSID
+ "\nChannel :: "
+ convertFrequencyToChannel(wifiList.get(0).frequency)
+ "\nFrequency :: " + wifiList.get(0).frequency
+ "\nCapability :: " + wifiList.get(0).capabilities);
Example:-
Here is an example demonstrating how to access Wi-Fi data from android application. We create a basic application that scans a list of wireless networks and populate details or information of Wi-Fi in a list view.
Here is an example; you need to run this on your mobile device wherein Wi-Fi is turned on.
Step 6 MainActivity.java
Following is the content of the modified main activity file src >> com.bhavin.AccessWifiData >> MainActivity.java
public class MainActivity extends Activity {
private WifiManager mainWifi;
private WifiReceiver receiverWifi;
private Button btnRefresh;
ListAdapter adapter;
ListView lvWifiDetails;
List wifiList;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
lvWifiDetails = (ListView) findViewById(R.id.lvWifiDetails);
btnRefresh = (Button) findViewById(R.id.btnRefresh);
mainWifi = (WifiManager) getSystemService(Context.WIFI_SERVICE);
receiverWifi = new WifiReceiver();
registerReceiver(receiverWifi, new IntentFilter(
WifiManager.SCAN_RESULTS_AVAILABLE_ACTION));
scanWifiList();
btnRefresh.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
scanWifiList();
}
});
}
private void setAdapter() {
adapter = new ListAdapter(getApplicationContext(), wifiList);
lvWifiDetails.setAdapter(adapter);
}
private void scanWifiList() {
mainWifi.startScan();
wifiList = mainWifi.getScanResults();
setAdapter();
}
class WifiReceiver extends BroadcastReceiver {
public void onReceive(Context c, Intent intent) {
}
}
}
Step 7 ListAdapter.java
Following is the content of the modifedListAdapter file src >> com.bhavin.AccessWifiData >> ListAdapter.java
packagecom.example.wifidataaccess;
importjava.util.List;
importandroid.content.Context;
importandroid.net.wifi.ScanResult;
importandroid.view.LayoutInflater;
importandroid.view.View;
importandroid.view.ViewGroup;
importandroid.widget.BaseAdapter;
importandroid.widget.TextView;
public class ListAdapter extends BaseAdapter {
Context context;
LayoutInflater inflater;
List wifiList;
publicListAdapter(Context context, List wifiList) {
this.context = context;
this.wifiList = wifiList;
inflater = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
}
@Override
public int getCount() {
returnwifiList.size();
}
@Override
public Object getItem(int position) {
returnnull;
}
@Override
public long getItemId(int position) {
return 0;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
Holder holder;
System.out.println("viewpos" + position);
View view = convertView;
if (view == null) {
view = inflater.inflate(R.layout.dataset, null);
holder = new Holder();
holder.tvDetails = (TextView) view.findViewById(R.id.tvDetails);
view.setTag(holder);
} else {
holder = (Holder) view.getTag();
}
holder.tvDetails.setText("SSID :: " + wifiList.get(position).SSID
+ "\nStrength :: " + wifiList.get(position).level
+ "\nBSSID :: " + wifiList.get(position).BSSID
+ "\nChannel :: "
+ convertFrequencyToChannel(wifiList.get(position).frequency)
+ "\nFrequency :: " + wifiList.get(position).frequency
+ "\nCapability :: " + wifiList.get(position).capabilities);
return view;
}
public static int convertFrequencyToChannel(intfreq) {
if (freq>= 2412 &&freq<= 2484) { return (freq - 2412) / 5 + 1; } elseif (freq>= 5170 &&freq<= 5825) {
return (freq - 5170) / 5 + 34;
} else {
return -1;
}
}
class Holder {
TextView tvDetails;
}
}
Step 8 activity_main.xml
Following is the modified content of the xml res >> layout >> activity_main.xml.
<LinearLayoutxmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/LinearLayout1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context="${relativePackage}.${activityClass}">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="0.9"
android:orientation="vertical">
<ListView
android:id="@+id/lvWifiDetails"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="0.1"
android:orientation="vertical">
<Button
android:id="@+id/btnRefresh"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:background="@drawable/rounded_corner"
android:text="Refresh"/>
</LinearLayout>
</LinearLayout>
Step 9 dataset.xml
Following is the modified content of the xml res >> layout >> dataset.xml.
<?xmlversion="1.0"encoding="utf-8"?>
<LinearLayoutxmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/tvDetails"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@drawable/rounded_corner"
android:text="Medium Text"
android:textAppearance="?android:attr/textAppearanceMedium"
android:textColor="#000000"/>
</LinearLayout>
I hope you find this blog very helpful while Access Wi-Fi data in Android. Let me know in comment if you have any question regarding Android. I will reply you ASAP.
Got an Idea of Android App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Android App Development Company in India.
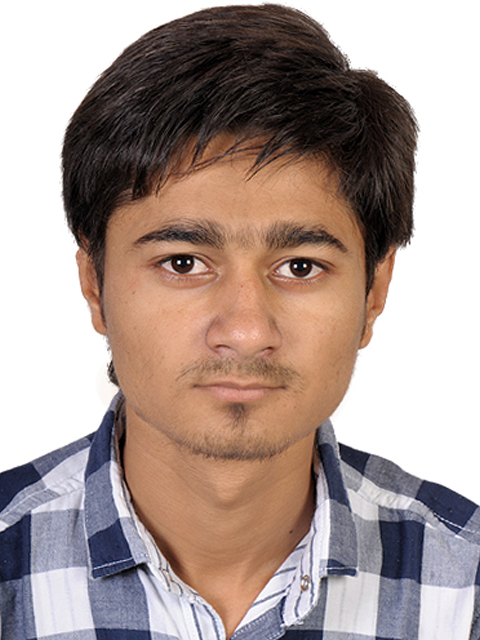
I am passionate Android Application Developer. Love to develop Application with Unique thing. And also like to share tips & tricks about latest Application trends.
Lighting in Photoshop Using Alpha Channel