
Be patient..... we are fetching your source code.
Objective
The main objective of this post is to describe that how to parse and write .CSV file in iOS.
You will get Final Output:
Following are the steps that give you a brief introduction to using CSVParser to interact with any generated .CSV file. CSV stands for comma-separated values. CSV file stores tabular data in the plain-text form. Plain text has the sequence of characters. A CSV file consists of any number of records, in which each record consists of fields, fields are separated by some other character or string, most commonly used characters are tab, space or comma.
Step 1 About CHCSVParser
CHCSVParser If you want to use CHCSVParser, following two files need to be added to your project:
- CHCSVParser.h
- CHCSVParser.m
A CHCSVParser is very similar to NSXMLParser and works in the same way, it parses data and call its delegate methods and inform us that if it has found a field or finished current line reading or finish document reading or else whether if an error has occurred in same. We can use CHCSVParser in three different ways:
- By providing the path to a file.
- By providing contents with use of NSString.
- With the help of NSInputStream.
You can clone their repository on https://github.com/davedelong/CHCSVParser. The first step is to download/clone CHCSVParser from the URL above. Once downloaded, drag everything and drop it into the folder named src in your project.
Step 2 Add .csv file
Next step is to drag .csv file that you want to parse into the folder named src. in our case, we use a CSV file named demo.csv.
Step 3 Import CHCSVParser.h file
Now import CHCSVParser.h file to your main view controller as well as set its appropriate parser delegate, you can have an idea from the following lines of code.
#import <UIKit/UIKit.h>
#import "CHCSVParser.h"
@interface ViewController : UIViewController
Step 4 Design UI
Prepare UserInterface as per following picture.
Step 5 CHCVParserDelegate methods
Apply following delegate methods into your main ViewController.m file as follows:
-(void) parserDidBeginDocument:(CHCSVParser *)parser
{
}
-(void) parserDidEndDocument:(CHCSVParser *)parser
{
}
- (void) parser:(CHCSVParser *)parser didFailWithError:(NSError *)error
{
NSLog(@"Parser failed with error: %@ %@", [error localizedDescription], [error userInfo]);
}
-(void)parser:(CHCSVParser *)parser didBeginLine:(NSUInteger)recordNumber
{
}
-(void)parser:(CHCSVParser *)parser didReadField:(NSString *)field atIndex:(NSInteger)fieldIndex
{
}
- (void) parser:(CHCSVParser *)parser didEndLine:(NSUInteger)lineNumber
{
}
Step 6 Code for parse .csv file
You can parse CSV data using the following line of code:
CHCSVParser *parser=[[CHCSVParser alloc] initWithContentsOfCSVFile:[NSHomeDirectory() stringByAppendingPathComponent:@"demo.csv"] delimiter:','];
parser.delegate=self;
[parser parse];
You can also write CSV data using the following line of code:
CHCSVParser *parser=[[CHCSVParser alloc] initWithContentsOfCSVFile:[NSHomeDirectory() stringByAppendingPathComponent:@"demo.csv"] delimiter:','];
parser.delegate=self;
[parser parse];
CHCSVWriter *csvWriter=[[CHCSVWriter alloc]initForWritingToCSVFile:[NSHomeDirectory() stringByAppendingPathComponent:@"demo.csv"]];
NSLog(@"%d",[currentRow count]);
[csvWriter writeField:@"Roll Number"];
[csvWriter writeField:@"Name"];
[csvWriter writeField:@"Marks"];
[csvWriter finishLine];
for(int i=1;i<[currentRow count];i++)
{
[csvWriter writeField:[[currentRow objectAtIndex:i] valueForKey:[NSString stringWithFormat:@"0"]]];
[csvWriter writeField:[[currentRow objectAtIndex:i] valueForKey:[NSString stringWithFormat:@"1"]]];
[csvWriter writeField:[[currentRow objectAtIndex:i] valueForKey:[NSString stringWithFormat:@"2"]]];
[csvWriter finishLine];
}
[csvWriter writeField:[txtRno text]];
[csvWriter writeField:[txtName text]];
[csvWriter writeField:[txtMarks text]];
[csvWriter closeStream];
When you parse or write CSV data following line of code with parser delegate methods should be applied.
-(void) parserDidBeginDocument:(CHCSVParser *)parser
{
currentRow = [[NSMutableArray alloc] init];
}
-(void) parserDidEndDocument:(CHCSVParser *)parser
{
for(int i=0;i<[currentRow count];i++)
{
NSLog(@"%@ %@ %@",[[currentRow objectAtIndex:i] valueForKey:[NSString stringWithFormat:@"0"]],[[currentRow objectAtIndex:i] valueForKey:[NSString stringWithFormat:@"1"]],[[currentRow objectAtIndex:i] valueForKey:[NSString stringWithFormat:@"2"]]);
}
}
- (void) parser:(CHCSVParser *)parser didFailWithError:(NSError *)error
{
NSLog(@"Parser failed with error: %@ %@", [error localizedDescription], [error userInfo]);
}
-(void)parser:(CHCSVParser *)parser didBeginLine:(NSUInteger)recordNumber
{
dict=[[NSMutableDictionary alloc]init];
}
-(void)parser:(CHCSVParser *)parser didReadField:(NSString *)field atIndex:(NSInteger)fieldIndex
{
[dict setObject:field forKey:[NSString stringWithFormat:@"%d",fieldIndex]];
}
- (void) parser:(CHCSVParser *)parser didEndLine:(NSUInteger)lineNumber
{
[currentRow addObject:dict];
dict=nil;
}
If you have got any query related to CSV Parser & writer in iOS comment them below.
Got an Idea of iPhone App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best iPhone App Development Company in India.
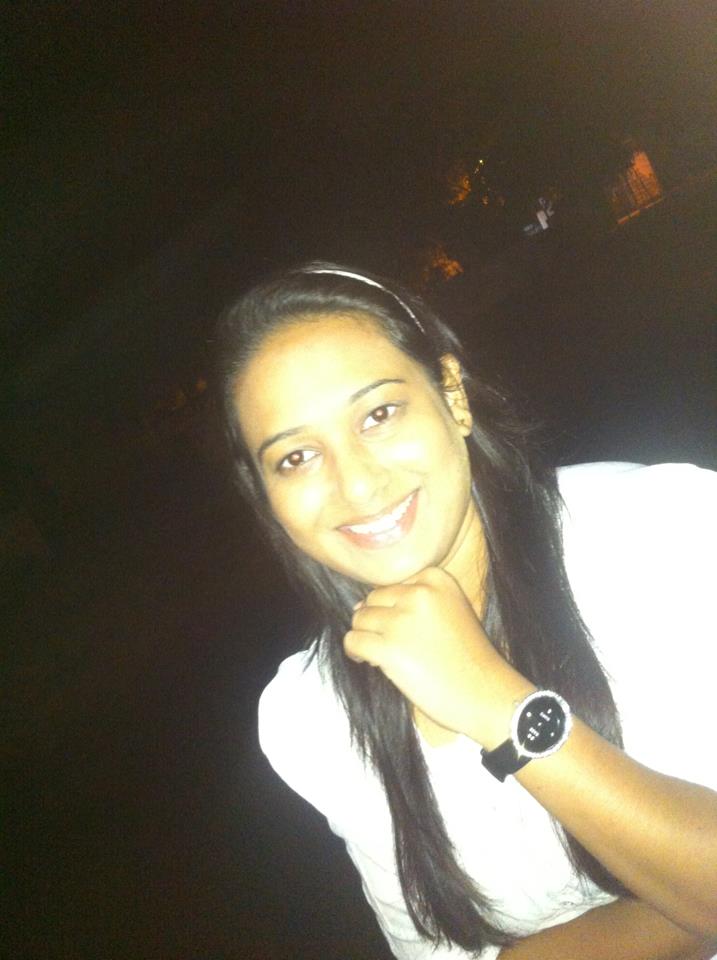
I am iOS developer, as a developer my basic goal is continue to learn and improve my development skills , to make application more user friendly.
Android - Flashlight / TorchLight Implementation