
Be patient..... we are fetching your source code.
- Create Xcode Project
- Download Dropbox Thirdparty
- Add third party to Project
- Initialized Dropbox third party
- Add API_KEY in Info.plist
- DBSessionDelegate methods
- DBNetworkRequestDelegate methods
- Code for Login & Logout
- DBRestClientDelegate methods
- CDBRestClientDelegate handle response
- Code for downloading Image from Dropbox
- DBRestClientDelegate methods for Download
- Code for uploading Image to Dropbox
- DBRestClientDelegate methods for Upload
- Code for folder creating in Dropbox
- DBRestClientDelegate methods for Folder Creation
Objective
The main objective of this post is to describe how to integrate Dropbox framework in project and use it to upload and download files in iOS.
The Dropbox framework is third party that is available for file sharing with friends from your phone. Using Dropbox can upload, download and share files using the iOS device.
Following are the steps that give you a brief introduction to use Dropbox framework for file uploading and downloading.
Step 1 Create Xcode Project
Create new XCode project name it as DropboxIntegration. It contains Three ViewController one for file uploading, one for file downloading and another for create folder in Main.storyboard file.
Step 2 Download Dropbox Thirdparty
You can download DropboxSDK from https://www.dropbox.com/developers/core/sdks/ios. First download this project from given url. Once it downloaded add DropboxSDK.framework file in to your project’s ThirdParty folder.
Step 3 Add third party to Project
Now to link Dropbox library in Build Phase section open Link Binary With Libraries. Then press + to add library and then choose Add Other using this option you can choose custom library. Here choose DropboxSDK.framework file which we have added in Step - 1. Also add inbuilt Security.framework file.
Now you can use Dropbox framework file by importing DropboxSDK file.
#import <DropboxSDK/DropboxSDK.h>
Step 4 Initialized Dropbox third party
Generate your AppKey and AppSecret from your Dropbox account and then set this in your project. For Dropbox session and Network request set this DBSessionDelegate, DBNetworkRequestDelegate two delegate in your AppDelegate file. Now for Dropbox session setting add below code in your
(BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions method of AppDelegate class
NSString *dropBoxAppKey = @"YOUR_APP_KEY";
NSString *dropBoxAppSecret = @" YOUR_APP_SECRET";
NSString *root = kDBRootDropbox;
DBSession* session =
[[DBSession alloc] initWithAppKey:dropBoxAppKey appSecret:dropBoxAppSecret root:root];
session.delegate = self;
[DBSession setSharedSession:session];
[DBRequest setNetworkRequestDelegate:self];
Step 5 Add API_KEY in Info.plist
Also add this dropBoxAppKey (db- YOUR_APP_KEY) in your info.plist file to register your app for a custom URL scheme in order to receive an login response.
Step 6 DBSessionDelegate methods
In your AppDelegate file override below delegate methods of DBSessionDelegate.
#pragma mark - DBSessionDelegate methods
- (void)sessionDidReceiveAuthorizationFailure:(DBSession*)session userId:(NSString *)userId
{
relinkUserId = userId;
[[[UIAlertView alloc] initWithTitle:@"Dropbox Session Ended" message:@"Do you want to relink?" delegate:self
cancelButtonTitle:@"Cancel" otherButtonTitles:@"Relink", nil] show];
}
If in case you will get Failure in authorization then to Relink user add alert for confirmation and if user want to Relink then add below Delegate method of UIAlertView to Relink user.
#pragma mark UIAlertViewDelegate methods
- (void)alertView:(UIAlertView *)alertView clickedButtonAtIndex:(NSInteger)index
{
if (index != alertView.cancelButtonIndex) {
[[DBSession sharedSession] linkFromController:[self.navigationController visibleViewController]];
}
relinkUserId = nil;
}
Step 7 DBNetworkRequestDelegate methods
In your AppDelegate class also override the below delegate methods of DBNetworkRequestDelegate
#pragma mark DBNetworkRequestDelegate methods
static int outstandingRequests;
- (void)networkRequestStarted
{
outstandingRequests++;
if (outstandingRequests == 1) {
[[UIApplication sharedApplication] setNetworkActivityIndicatorVisible:YES];
}
}
- (void)networkRequestStopped
{
outstandingRequests--;
if (outstandingRequests == 0) {
[[UIApplication sharedApplication] setNetworkActivityIndicatorVisible:NO];
}
}
Step 8 Code for Login & Logout
Now for Login in Dropbox add below method. It first checks that user is already logged in or not. And if user is not logged in than it will open Login view.
if (![[DBSession sharedSession] isLinked]) {
[[DBSession sharedSession] linkFromController:self];
}
After successfully login it calls the below method of your delegate class
- (BOOL)application:(UIApplication *)application handleOpenURL:(NSURL *)url
{
if ([[DBSession sharedSession] handleOpenURL:url]) {
if ([[DBSession sharedSession] isLinked]) {
[[NSNotificationCenter defaultCenter] postNotification:[NSNotification notificationWithName:@"OPEN_DROPBOX_VIEW" object:nil]];
}
return YES;
}
return NO;
}
From this method to transfer control in a view from which you are running code of login here have added Post notification named OPEN_DROPBOX_VIEW. And add observer for this notification in class from which you are login for Dropbox.
Step 9 DBRestClientDelegate methods
Now to fetch data from Dropbox use DBRestClient object and add below function in your class to initialize DBRestClient object. Set DBRestClientDelegate in your class.
- (DBRestClient *)restClient
{
if (restClient == nil) {
restClient = [[DBRestClient alloc] initWithSession:[DBSession sharedSession]];
restClient.delegate = self;
}
return restClient;
}
After that call below method to fetch data from Dropbox. While calling this method first time make sure to set loadData = @””;
[self.restClient loadMetadata:loadData];
Step 10 DBRestClientDelegate handle response
In your class also override the below delegate methods of DBRestClientDelegate’s to handle response of loadMetadata: function call.
- (void)restClient:(DBRestClient*)client loadedMetadata:(DBMetadata *)metadata
{
for (int i = 0; i < [metadata.contents count]; i++) {
DBMetadata *data = [metadata.contents objectAtIndex:i];
if (data.isDirectory) {
//Add folder object in your array
}else {
//Add file object in your array
}
}
}
- (void)restClient:(DBRestClient *)client loadMetadataFailedWithError:(NSError *)error
{ }
Here you can check is current object of DBMetadata is folder or file by checking the isDirectory flag and then add your data in array respectively.
Step 11 Code for downloading Image from Dropbox
Now to download file from Dropbox add below method. In that method you have to pass the filePath (filePath - you can get from the DBMetaData object’s path variable which we get from Step - 9) of file which you want to download and toPath at which place you want to download your file.
For below example of toPath it downloads the file in Library folder with Image.png name.
[self.restClient loadFile:filePath intoPath:toPath];
e.g. toPath = [[NSSearchPathForDirectoriesInDomains(NSLibraryDirectory, NSUserDomainMask, YES) objectAtIndex:0] stringByAppendingPathComponent:@"Image.png"]
Step 12 DBRestClientDelegate methods for Download
In your class also override the below delegate methods of DBRestClientDelegate’s to handle response of loadFile: function call.
- (void)restClient:(DBRestClient*)client loadedFile:(NSString*)destPath
{
//Here destPath is the path at which place your image is downloaded
}
-(void)restClient:(DBRestClient *)client loadFileFailedWithError:(NSError *)error
{ }
Step 13 Code for uploading Image to Dropbox
To upload your file in Dropbox account use below method. In this method you have to pass filePath (filePath - you can get from the DBMetaData object’s path variable which we get from Step - 9) which is a path of folder at which place you want to upload your file and FileName.png is filename which is used as a name of file in Dropbox.
And fromPath is a path from which you want to upload your file for below example of fromPath it will upload file from Library folder.
[self.restClient uploadFile:@"FileName.png" toPath:filePath withParentRev:@"" fromPath:fromPath];
e.g. fromPath = [[NSSearchPathForDirectoriesInDomains(NSLibraryDirectory, NSUserDomainMask, YES) objectAtIndex:0] stringByAppendingPathComponent:@"Image.png"]
<strong>Step 14:</strong> In your class also override the below delegate methods of DBRestClientDelegate’s to handle response of uploadFile: function call.
-(void)restClient:(DBRestClient *)client uploadedFile:(NSString *)destPath from:(NSString *)srcPath
{
//destPath is a path at which place your file is uploaded.
//srcPath is a path from which place your file is uploaded.
}
-(void)restClient:(DBRestClient *)client uploadFileFailedWithError:(NSError *)error
{
}
Step 14 DBRestClientDelegate methods for Upload
In your class also override the below delegate methods of DBRestClientDelegate’s to handle response of uploadFile: function call.
-(void)restClient:(DBRestClient *)client uploadedFile:(NSString *)destPath from:(NSString *)srcPath
{
//destPath is a path at which place your file is uploaded.
//srcPath is a path from which place your file is uploaded.
}
-(void)restClient:(DBRestClient *)client uploadFileFailedWithError:(NSError *)error
{
}
Step 15 Code for folder creating in Dropbox
To create folder in Dropbox programmatically add below method in which you have to pass your folder path.
e.g folderPath = [folderPath stringByAppendingPathComponent:folderName]
[self.restClient createFolder:folderPath];
Step 16 DBRestClientDelegate methods for Folder Creation
In your class also override the below delegate methods of DBRestClientDelegate’s to handle response of createFolder: function call.
- (void)restClient:(DBRestClient*)client createdFolder:(DBMetadata*)folder
{
//folder is a object of folder which you created.
}
- (void)restClient:(DBRestClient*)client createFolderFailedWithError:(NSError*)error
{
}
If you have any query related to DropBox Integration in iOS then comment them below.
Got an Idea of iPhone App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best iPhone Application Development Company in India.
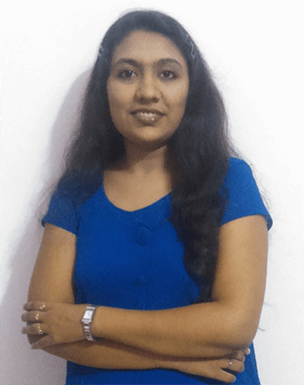
Being an iOS developer with experience of 3 years feels pretty awesome because much more than just playing around with code, software developers are the brains behind the design, installation, testing and maintenance of software systems.
Android - Intro to Box SDK for Android