
Be patient..... we are fetching your source code.
- Setup for Swift Project
- Main files of project
- Make UI for Search functionality in TableView
- Make Local Variable
- Initialize TableView with Country Data
- Implement TableView datasource & delegate method
- Write code for SearchBar Delegate & DataSource method
- Update TableView Delegate / DataSource method
- Run the App
Objective
This blog post helps you to make application in Swift and how to filter tableview data with Swift.
For this purpose Follow the below steps which make your filter functionality enable successfully.
You will get final output:
After Search Keyword, It's filter TableView which look like:
So, let’s start filter the tableview data in Swift.
Step 1 Setup for Swift Project
Xcode latest version is an IDE to create all apps using Swift Language but it requires OS X latest version installed in your mac. Now, please open your Project.
The below screen will appered on your screen:
Select Create a new Xcode project center option.
The below screen will appered on your screen:
Now, select third option Single View Application and press next.
It appears Detail of Project:
The Project screen contains some data. User must provide information into above field, Set app name TableViewSearchInSwift or whatever you want to give name and click next. Here, Project Language must be Swift for Swift language.
Give project path and click Create for create project.
Step 2 Main files of project
File structure in your project is looks like following. Here, we use ViewController.swift file.
So, please make a design like following given screenshot:
Step 3 Make UI for Search functionality in TableView
Now, Create layout which is shown in above screenshot. Which is One TableViewController and Make .h & .m file for it give name SearchTableViewController.swift and embeded NavigationController.
Now, put SearchBar and Search Display controller in TableView just above the cell which is shown in above fig.(in left side panel).
Your UI looks like:
Step 4 Make Local Variable
Now, to filter the tableview data, we use two variable named marrCountryList and marrFilteredCountryList and also create IBOutlet for UITableView.
It looks like:
var marrCountryList = [String]()
var marrFilteredCountryList = [String]()
@IBOutlet var tblCountry: UITableView!
Step 5 Initialize TableView with Country Data
Now, our first of all we fill table with Country name list. For that we used marrCountryList array and in viewDidLoad() method.
We have to write following code:
override func viewDidLoad()
{
super.viewDidLoad()
marrCountryList = ["USA", "Bahamas", "Brazil", "Canada", "Republic of China", "Cuba", "Egypt", "Fiji", "France", "Germany", "Iceland", "India", "Indonesia", "Jamaica", "Kenya", "Madagascar", "Mexico", "Nepal", "Oman", "Pakistan", "Poland", "Singapore", "Somalia", "Switzerland", "Turkey", "UAE", "Vatican City"]
self.tblCountry.reloadData()
}
Here, we load data with country list and after that we simple reload the tableview.
Step 6 Implement TableView datasource & delegate method
Now, we fill the tableview data by marrCountryList array. For that we must write below methods for tableview.
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int
{
return self.marrFilteredCountryList.count
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell
{
let cellCountry = self.tblCountry.dequeueReusableCellWithIdentifier("cell", forIndexPath: indexPath)
var countryName : String!
countryName = marrCountryList[indexPath.row]
cellCountry.textLabel?.text = countryName
return cellCountry
}
Now, run your project with cmd + r & cmd + b its gives output with country name filled in tableview.
Step 7 Write code for SearchBar Delegate & DataSource method
Now for searching functionality we must add delegate & data source method for SearchBar and SearchDisplay control.
So, now make below changes in your code:
class SearchTableViewController: UITableViewController, UISearchDisplayDelegate, UISearchBarDelegate
{
}
Here, we implement UISearchBarDelegate & UISearchDisplayDelegate method. Now, put this delegate & data source methods in your code which looks like:
func filterTableViewForEnterText(searchText: String) {
let searchPredicate = NSPredicate(format: "SELF CONTAINS[c] %@", searchText)
let array = (self.marrCountryList as NSArray).filteredArrayUsingPredicate(searchPredicate)
self.marrFilteredCountryList = array as! [String]
self.tblCountry.reloadData()
}
This function filterTableViewForEnterText is used for search text which is in our array or not. This function made by us and this is not a part of any delegate or data source methods. In this function, we passed searchText string which checks the given search keyword contains in our country name list or not.
func searchDisplayController(controller: UISearchDisplayController, shouldReloadTableForSearchString searchString: String?) -> Bool {
self.filterTableViewForEnterText(searchString!)
return true
}
This method is used for reload the tableview by entering the search string. This method calls our normal method filterTableViewForEnterText which checks the search keyword is in our list or not and after that it’s reload the tableview data.
func searchDisplayController(controller: UISearchDisplayController,
shouldReloadTableForSearchScope searchOption: Int) -> Bool {
self.filterTableViewForEnterText(self.searchDisplayController!.searchBar.text!)
return true
}
Here this method is defined a scope of searchbar which is used for search text area where user enter their search keyword and make filter with that keyword by calling our funciton filterTableViewForEnterText.
Step 8 Update TableView Delegate/ DataSource method
Now, for searching functionality we used another array named marrFilteredCountryList So, we must reload the table with new data after enter the search keyword.
Tableview methods looks like:
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int
{
if tableView == self.searchDisplayController!.searchResultsTableView {
return self.marrFilteredCountryList.count
} else {
return self.marrCountryList.count
}
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell
{
let cellCountry = self.tblCountry.dequeueReusableCellWithIdentifier("cell", forIndexPath: indexPath)
var countryName : String!
if tableView == self.searchDisplayController!.searchResultsTableView {
countryName = marrFilteredCountryList[indexPath.row]
} else {
countryName = marrCountryList[indexPath.row]
}
cellCountry.textLabel?.text = countryName
return cellCountry
}
Step 9 Run the App
Now, finally first build the app with cmd + b and Run the app with cmd + r.
You get message looks like:
I hope you find this blog is very helpful while working with Tableview search in Swift. Let me know in comment if you have any questions regarding Swift. I will reply ASAP.
Got an Idea of iPhone App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best iPhone App Development Company in India.
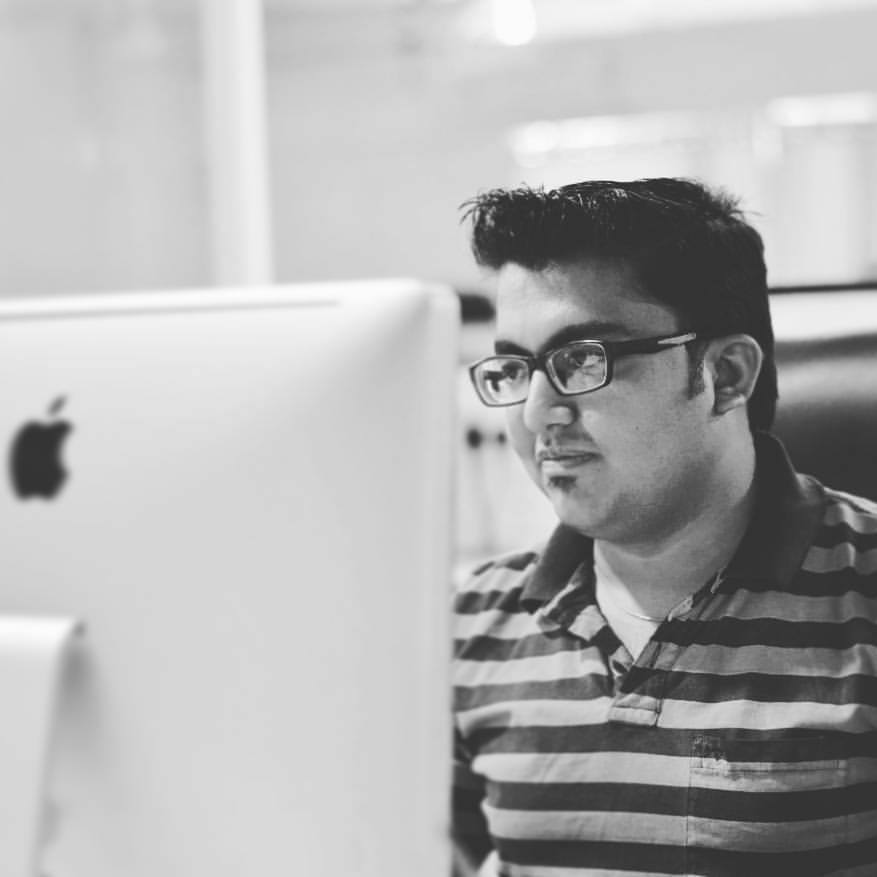
I am iOS Application Developer & I personally believed that When a programming language is created that allows programmers to program in simple English, it will be discovered that programmers cannot speak English.
Enemy Follow Script (AI) in Unity