
Be patient..... we are fetching your source code.
Objective
This blog post gives introduction to the UIPageViewController in iOS.
Introduction:
UIPageViewController is used to navigate between the screens or in simple words pages with animations. The screen navigation is controlled by the user gestures. Smart phone users are aware of the scrolling between the pages in the Home Screen of the device. This is done by the use of UIPageViewController. UIPageViewController in iOS is a highly configurable class.
We are allowed to define:
Orientation of the page views | vertical or horizontal |
Transition style | page curl transition style or scrolling transition style |
Ocation of the spine | only applicable to page curl transition style |
Space between pages | only applicable to scrolling transition style to define the inter-page spacing |
In this post I am going to create a simple example of UIPageViewController which uses the scroll transition style to navigate between three screens which will have 3 different images of cartoon characters. So, lets get started.
Step 1 Create Xcode Project for UIPageViewController
Create a new xcode project for UIPageViewController example under the iOS Application and create single view application. Here we can see that page based application is also available for navigating through pages, but in this post I am concentrating upon navigation between different pages or screens using UIPageViewController, which is easier than page based application.
Here, I am giving the iOS Page View Controller product name as PageViewDemo. And the and organization name as com.TheAppGuruz. You can enter the product name and organization name as per your preference. Press next and store the Pagve View Controller Tutorial project according to your preference.
Press create, and the project for Xcode Page View Controller in iOS is created.
You can get more detail about creating XCode project from following link:
Step 2 Design UI
Drag a new PageViewController which is subclass of UIPageViewController. And a new ViewController on to the storyboard.
The UIPageViewController storyboard shall look as shown below:
Step 3 Add ViewControllers
In this iOS PageViewController tutorial, the original view controller is used as the root view controller for holding the page view controller. The view controller we've just added will be used for displaying the page content. Throughout the post, we will refer the original view controller as the root view controller and the other view controller as page content controller. For that delete the default viewcontroller.h and viewcontroller.m files and create new class under the UIViewController Class and name it as RootViewController.
In the 3rd inspector of default view controller in the class section select RootViewController, hence the default view controller inherits from the RootViewController class. Similarly for the page content view controller create a new class under the UIViewController and name it as PageContentViewController. And in the 3rd inspector of new view controller select the class as PageContentViewController which means the new view controller inherits from PageContentViewController.
Now the two view controllers titles will appear as shown below:
Step 4 Set storBoard ID
Next we will assign Storyboard ID for PageViewController and PageContentViewController which we will use later in our code. For that select the PageViewController and in the identity inspector enter the text PageViewController, also select PageContentViewController and in the identity inspector enter text PageContentViewController.
By default, the transition style of the page view controller is set as Page Curl. The page curl style is perfect for book apps. For navigating between screens, we will use scrolling style. So change the transition style to Scroll under Attribute Inspector.
Step 5 Design UI
Now we will create the user interface for the PageContentViewController for that drag ImageView and Label on to the PageContentViewController’s view put the label on to the ImageView in the upper portion. The ImageView will contain different images for different screens and the label will have some text about the image. Change the label text color to pink color.
The PageContentViewController will look as shown below:
For the root view controller, add a "Start Again" button and put it at the below of the screen.
Step 6 Create IBOutlet
Now create an Outlet from the ImageView and Label in the PageContentViewController.h file and name it as ivScreenImage and lblScreenLabel respectively.
Next we create NString for holding the current images file and current image text for that we add the following line of code in PageViewController.h file. Also we will have NSUInteger for keeping page index.
@property NSUInteger pageIndex;
@property NSString *imgFile;
@property NSString *txtTitle;
And in the PageContentViewController.m file in the ViewDidiLoad Method implement the following line of code:
- (void)viewDidLoad
{
[super viewDidLoad];
self.ivScreenImage.image = [UIImage imageNamed:self.imgFile];
self.lblScreenLabel.text = self.txtTitle;
}
Step 7 Intialized UIPageViewController
In order to make UIPageViewController work, we must adopt the UIPageViewControllerDataSource protocol. The data source for a page view controller is responsible for providing the content view controllers on demand. By implementing the data source protocol, we tell the page view controller what to display for each page.
In this case, we use the RootViewController class as the data source for the UIPageViewController instance. Therefore it is necessary to declare the RootViewController class as implementing the UIPageViewControllerDataSource protocol.
The RootViewController class is also responsible to provide the data of the page content (i.e. images and titles). Open the RootViewController.h.
Add the following line of code:
@interface RootViewController : UIViewController
<UIPageViewControllerDataSource>
@property (nonatomic,strong) UIPageViewController *PageViewController;
@property (nonatomic,strong) NSArray *arrPageTitles;
@property (nonatomic,strong) NSArray *arrPageImages;
- (PageContentViewController *)viewControllerAtIndex:(NSUInteger)index;
- (IBAction)btnStartAgain:(id)sender;
@end
In the RootViewController.m file initialize the arrays for pageimages and pagetitles in ViewDidLoad Method:
[super viewDidLoad];
arrPageTitles = @[@"This is The App Guruz",@"This is Table Tennis 3D",@"This is Hide Secrets"];
arrPageImages =@[@"1.jpg",@"2.jpg",@"3.jpg"];
Step 8 Delegate & Datasource method
Next we will add the two required methods for the UIPageViewDatasource in the RootViewController as follows:
- (UIViewController *)pageViewController:(UIPageViewController *)pageViewController viewControllerBeforeViewController:(UIViewController *)viewController
{
NSUInteger index = ((PageContentViewController*) viewController).pageIndex;
if ((index == 0) || (index == NSNotFound))
{
return nil;
}
index--;
return [self viewControllerAtIndex:index];
}
- (UIViewController *)pageViewController:(UIPageViewController *)pageViewController viewControllerAfterViewController:(UIViewController *)viewController
{
NSUInteger index = ((PageContentViewController*) viewController).pageIndex;
if (index == NSNotFound)
{
return nil;
}
index++;
if (index == [self.arrPageTitles count])
{
return nil;
}
return [self viewControllerAtIndex:index];
}
Here you can see that we have created a helper method i.e. viewControllerAtIndex:index.
The definition for this method goes as below:
- (PageContentViewController *)viewControllerAtIndex:(NSUInteger)index
{
if (([self.arrPageTitles count] == 0) || (index >= [self.arrPageTitles count])) {
return nil;
}
// Create a new view controller and pass suitable data.
PageContentViewController *pageContentViewController = [self.storyboard instantiateViewControllerWithIdentifier:@"PageContentViewController"];
pageContentViewController.imgFile = self.arrPageImages[index];
pageContentViewController.txtTitle = self.arrPageTitles[index];
pageContentViewController.pageIndex = index;
return pageContentViewController;
}
To display a page indicator, you have to tell iOS the number of pages (i.e. dots) to display in the page view controller and which page must be selected at the beginning. Add the following two methods at the end of the RootViewController.m file.
-(NSInteger)presentationCountForPageViewController:(UIPageViewController *)pageViewController
{
return [self.arrPageTitles count];
}
- (NSInteger)presentationIndexForPageViewController:(UIPageViewController *)pageViewController
{
return 0;
}
Step 9 Add PageView Controller
In this final step we will create and initiate the UIPageViewController and for that we will make use of the Storyboard ID of the PageViewController. Remember we discussed that we will use the Storyboard ID further in our code. Hence change the ViewDidLoad method to:
- (void)viewDidLoad
{
[super viewDidLoad];
arrPageTitles = @[@"This is The App Guruz",@"This is Table Tennis 3D",@"This is Hide Secrets"];
arrPageImages =@[@"1.jpg",@"2.jpg",@"3.jpg"];
// Create page view controller
self.PageViewController = [self.storyboard instantiateViewControllerWithIdentifier:@"PageViewController"];
self.PageViewController.dataSource = self;
PageContentViewController *startingViewController = [self viewControllerAtIndex:0];
NSArray *viewControllers = @[startingViewController];
[self.PageViewController setViewControllers:viewControllers direction:UIPageViewControllerNavigationDirectionForward animated:NO completion:nil];
// Change the size of page view controller
self.PageViewController.view.frame = CGRectMake(0, 0, self.view.frame.size.width, self.view.frame.size.height - 30);
[self addChildViewController:PageViewController];
[self.view addSubview:PageViewController.view];
[self.PageViewController didMoveToParentViewController:self];
}
Step 10 Set PageViewController in AppDelegate
In this step we customize the look of the PageView i.e. we will change the color of the dots in the PageView. For that add the following lines of code in the AppDelegate.m file in didFinishLaunchingWithOptions method:
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
UIPageControl *pageControl = [UIPageControl appearance];
pageControl.pageIndicatorTintColor = [UIColor lightGrayColor];
pageControl.currentPageIndicatorTintColor = [UIColor blackColor];
pageControl.backgroundColor = [UIColor whiteColor];
return YES;
}
I hope you will find this blog post very useful. While working with UIPageViewController in IOS. Let me know in comment if you have any questions regarding UIPageViewController in IOS. I will reply you ASAP.
Got an Idea of iPhone App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best iPhone App Development Company in India.
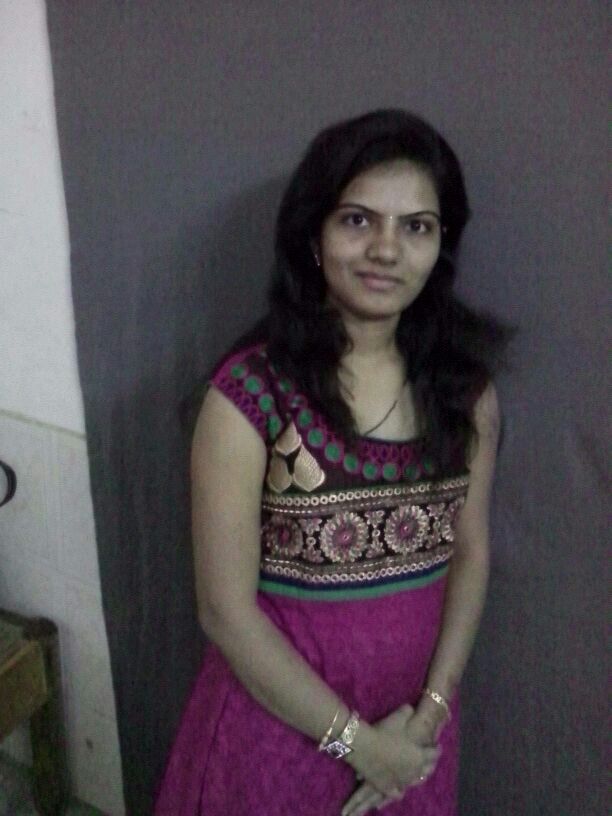
I am a Techno Freak who likes to explore new technologies and as an iOS developer and enthusiast I try to learn new things which polish my development skills, so that applications are made more users friendly.
Create Classic Snake Game in Unity 2D