
Be patient..... we are fetching your source code.
Objective
The main objective of this blog post is to explain what is delegate and how it is helpful in iOS applications.
You will get Final Output:
What is delegate?
Delegate is simply just a means of communication between objects of iOS applications. You can think delegation as a simple way of connecting objects and communicate with each other. In other words we can say that delegate allows one object to send message to other object when any event occurs. Lets understand this by a real life example. Suppose there is any office with 5 counters. You enter into the office and go to counter 1 and submit some details on that counter.
Now your details need to pass through some process that counter 3 know but counter 1 doesn’t. So counter 1 will tell you to wait for some time and pass your details to counter 3 to get the process complete. In this case counter 3 is the delegate that handles your events, which is referred by counter 1. After counter 3 completes processing your data, it sends back the message to counter 1 informing that the process is done and you can proceed further now.
Example:
You might have worked with UIAlertViews.
E.g:
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@"Title" message:@"This is an alert view" delegate:self cancelButtonTitle:@"OK" otherButtonTitles:nil, nil];
The initializer method of UIAlertView has a parameter named delegate. Setting this parameter to self, which means that the current object is responsible for handling all event fired by this instance of UIAlertView class.
If you don’t want to handle the events then you can simply set this parameter to nil as shown below:
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@"Title" message:@"This is an alert view" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles:nil, nil];
If you have multiple buttons on alert and you want to know which button is tapped, then you can implement the below method defined in UIAlertViewDelegate protocol.
-(void)alertView:(UIAlertView *)alertView clickedButtonAtIndex:(NSInteger)buttonIndex
{
NSLog(@"You have tapped button # %@",[NSString stringWithFormat:@"%d",buttonIndex]);
}
This was an example of pre-defined delegate. But what if we want to create our own delegate? So lets learn how to create your own delegate:
How to create user-defined delegate?
Lets learn how to create user-defined delegate by creating a simple example step-by-step. We will create an example to get first name and last name from the user and display the full name. To understand the concept of delegate, we will get the last name from different ViewController where we will set the delegate to pass the last name to the first ViewController.
Step 1 Create Project & Design UI
First of all create a new Xcode project named DelegateDemo and save it. It will contain one ViewController.
Now open the Main_iPhone.storyboard file and modify it as shown in below figure:
Step 2 Create new Class
Create a new class named LastNameViewController which will be the subclass of UIViewController class and assign this class to the second ViewController.
Step 3 Segue for Open new view
Now create segue from the button in first ViewController to the second ViewController.
Step 4 Create Protocol in .h file
Now it’s time to create your own delegate. Go to the LastNameViewController.h file and modify it as below:
#import <UIKit/UIKit.h>
@protocol LastNameViewControllerDelegate
-(void)setLastName:(NSString *)lastName;
@end
@interface LastNameViewController : UIViewController
@property (nonatomic,retain) id delegate;
@property (weak, nonatomic) IBOutlet UITextField *txtLastName;
- (IBAction)btnDoneTapped:(id)sender;
@end
Here, to create your own delegate, first of all you have to create a protocol and declare the necessary methods without its implementation. Secondly you have to create a delegate @property. Also IBOutlet the last name text field as txtLastName and create IBAction btnDoneTapped() on done button in second ViewController.
Step 5 Call Protocol methods
Now go to LastNameViewController.m file and modify btnDoneTapped() method as below:
- (IBAction)btnDoneTapped:(id)sender
{
[txtLastName resignFirstResponder];
[self.delegate setLastName:txtLastName.text];
[self.navigationController popViewControllerAnimated:YES];
}
Here, we set the value of last name to the delegate we have created in previous step. Now follow the next step to get this value in first view controller
i.e ViewController.m file
Step 6 Set Delegate of Created Protocol
To get the value from LastNameViewController.m file to ViewController.m file, first of all we have to set the delegate to itself in ViewController.m file. To set the deleagte to itself, we have to add the below method in ViewController.m file:
-(void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender
{
LastNameViewController *lastNameViewController = [segue destinationViewController];
lastNameViewController.delegate = self;
}
We implement this method to set the delegate to itself because we are loading the LastNameViewController through segue. But for this you have to modify the ViewController.h file as shown below.
#import "LastNameViewController.h"
@interface ViewController : UIViewController
Step 7 Implement Delegate method
To get the value of last name, implement the delegate method in ViewController.m file as below:
-(void)setLastName:(NSString *)lastName
{
NSString *fullName = [NSString stringWithFormat:@"%@ %@",txtFirstName.text,lastName];
txtFullName.text = fullName;
}
If you have got any query related to iOS Delegates in comment them below.
I hope you found this blog helpful while working on iOS Delegates. Let me know if you have any questions or concerns regarding iOS, please put a comment here and we will get back to you ASAP. Got an Idea of iPhone App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best iPhone App Development Company in India.
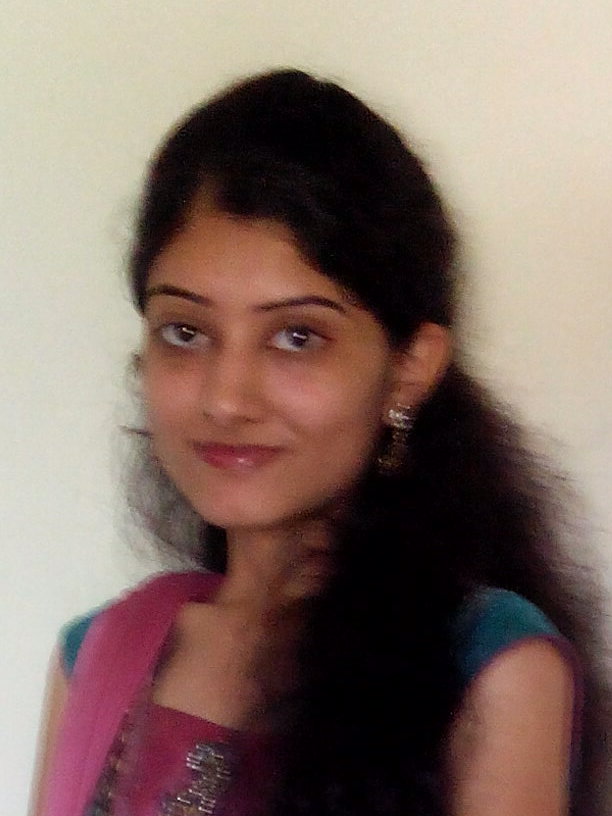
I am iOS Developer. I like to learn new technologies. I believe that any fool can write code that a computer can understand but good programmers write code that humans can understand.
Unity - Fire bullet with trail effect
iOS Image Picker Controller