
Be patient..... we are fetching your source code.
Objective
The main objective of this blog post is to explain how to Integrate LinkedIn Mobile SDK in your iOS application.
Step 1 Introduction
LinkedIn Mobile SDK provides following features:
- Allow a user to log in through the LinkedIn account.
- Get posts from the LinkedIn account.
- Add a post to the LinkedIn account.
In this blog post, I will explain how a user can log into our application through his/her LinkedIn Account. First of all, you will need an Application ID for LinkedIn SDK. And for that, you need a LinkedIn Account.
Step 2 Get LinkedIn Application ID
To use the LinkedIn SDK, we need LinkedIn Application ID. LinkedIn Application ID is an ID through which we can make calls to LinkedIn API from our iOS Application.
Follow these steps to get Application ID for your app:
- Sign into LinkedIn Developer using your LinkedIn Account.
- Create Application if you haven’t created it yet.
- Fill your new application details and click on Submit button.
- Now you will be redirected to Authenticate your application. Here you will find Client ID, Client Secret Key, and Application Permissions.
- Select Mobile from left menu.
Add your iOS Bundle Identifier in iOS Settings and click on Update button.
- Now you will find your iOS Bundle Identifier added to your application.
You can also find your Application ID there. Just copy and store it, we’ll need it later.
Step 3 Project Configuration
- Now create a new Xcode project. If you don’t know how to create a new project in Xcode, you can check the link given below to get more details on creating Xcode project.
- To integrate LinkedIn Mobile SDK, I would be using LinkedIn-SDK framework. You can get LinkedIn Mobile SDK by this link.
- Now, You will find LinkedIn-SDK.framework in the downloaded folder. Drag LinkedIn-SDK.framework to the project in Xcode.
- A new window appears to choose the options for adding files. Check Copy items if needed checkbox and click on Finish button.
- Now, you’ll find LinkedIn-SDK.framework framework in your Xcode project.
Step 4 Configure info.plist
Locate info.plist file in your Xcode project
Add LinkedIn App ID.
Add your LinkedIn App ID in URLTypes >> URLSchemes with the prefix of li:
If you are using secure (HTTPS) connections between your app and server, there will be no issues. But, if you are using insecure (HTTP) connections between your app and server, you must specify the insecure domain in your info.plist file.
Here you have to specify linkedin.com servers in App Transport Security:
Step 5 Use LinkedIn SDK in your application
5.1 Initial method
First of all, import LISDK.h into AppDelegate.h file.
#import <linkedin-sdk/LISDK.h>
Add the following method in your AppDelegate.m file.
(BOOL)application:(UIApplication *)application openURL:(NSURL *)url sourceApplication:(NSString *)sourceApplication annotation:(id)annotation
{
if ([LISDKCallbackHandler shouldHandleUrl:url])
{
return [LISDKCallbackHandler application:application openURL:url sourceApplication:sourceApplication annotation:annotation];
}
return YES;
}
This method will handle all the callbacks from the LinkedIn API call to your iOS application.
5.2 Create session with permission
import LISDK.h file to ViewController.h file.
#import <linkedin-sdk/LISDK.h>
First of all, you need to create a session before requesting to any API.
The following lines of code will create session to the LinkedIn app:
NSArray *permissions = [NSArray arrayWithObjects:LISDK_BASIC_PROFILE_PERMISSION, nil];
[LISDKSessionManager createSessionWithAuth:permissions state:nil showGoToAppStoreDialog:YES successBlock:^(NSString *returnState)
{
NSLog(@"%s","success called!");
LISDKSession *session = [[LISDKSessionManager sharedInstance] session];
NSLog(@"Session : %@", session.description);
} errorBlock:^(NSError *error)
{
NSLog(@"%s","error called!");
}];
Once a session is created, you can call the API to the LinkedIn server.
For example, I would be calling the getRequest() method to fetch the basic information of the logged in user.
[[LISDKAPIHelper sharedInstance] getRequest:@"https://api.linkedin.com/v1/people/~"
success:^(LISDKAPIResponse *response)
{
NSData* data = [response.data dataUsingEncoding:NSUTF8StringEncoding];
NSDictionary *dictResponse = [NSJSONSerialization JSONObjectWithData:data options:NSJSONReadingMutableContainers error:nil];
NSLog(@"Authenticated user name : %@ %@", [dictResponse valueForKey: @"firstName"], [dictResponse valueForKey: @"lastName"]);
} error:^(LISDKAPIError *apiError)
{
NSLog(@"Error : %@", apiError);
}];
By using this method, you can get user’s basic information. Here, I have Logged the Authenticated username using NSLog().
Challenge
- Add a post to user’s LinkedIn account.
- Get user’s post from the LinkedIn account.
You can get more details from following LinkedIn official document link:
I hope you will find this blog post helpful while working with LinkedIn Mobile SDK in iOS Application. Let me know in comment if you have any questions regarding LinkedIn Mobile SDK in iOS Application. Please put a comment here and we will get back to you ASAP.
Got an Idea of iPhone App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best iPhone App Development Company in India.
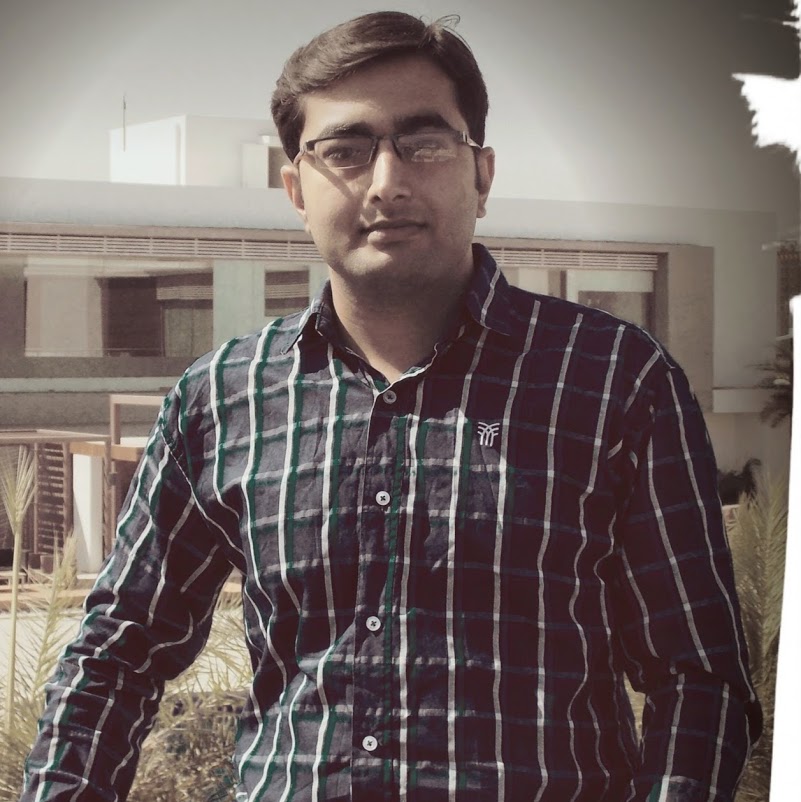
I am 2D Game developer and iOS Developer with an aspiration of learning new technology and creating a bright future in Information Technology.
Integrate Pushwoosh SDK In iOS