
Be patient..... we are fetching your source code.
Objective
Main objective of this blog post is to give you an idea about Android Gestures Tutorial.
We will make a simple pinch zoom application here.
Touch gesture: It is when a user places one or more fingers on the touch screen, and the application understands that as a signal or GESTURE. It has mainly two phases:
- Detect and Track data about touch events.
- Interpretation of that data as certain gesture by the application.
Android provides some of the common touch events such as a pinch, double tap, scrolls, flinch, long presses etc. known as gestures.
Android provides GestureDetector class to detect some of the basic motion events like Single Tap up, Long Press etc. It gathers data and tells us whether it is a gesture or not. To use it, you need to create an object of GestureDetector and then extend another class with GestureDetector.SimpleOnGestureListener to act as a listener and override some methods.
Syntax:
GestureDetector myG;
myG = new GestureDetector(this,new Gesture());
class Gesture extends GestureDetector.SimpleOnGestureListener{
public boolean onSingleTapUp(MotionEvent ev) {
}
public void onLongPress(MotionEvent ev) {
}
public boolean onScroll(MotionEvent e1, MotionEvent e2, float distanceX,
float distanceY) {
}
public boolean onFling(MotionEvent e1, MotionEvent e2, float velocityX,
float velocityY) {
}
}
}
Now, to handle some gestures like pinch zoom you need to use ScaleGestureDetector class.
Now, we will make a simple application for pinch zoom.
If you are new in Android Studio and don't know how to create a new project then you should checkout our blog:
Step 1 activity_main.xml
Take imageview and set scaletype as matrix.
<span style="font-family: Lato, sans-serif;"><span style="font-size: 16px; line-height: 24px; white-space: normal;"> </span></span><RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<ImageView
android:id="@+id/ivDemo"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="matrix"
android:src="@drawable/ic_launcher" />
</RelativeLayout>
Step 2 Create a ScaleGestureDetector class object.
scaleGestureDetector = new ScaleGestureDetector(this, new ScaleListener());
Here you can see we are passing ScaleListner class. It is extended by ScaleGestureDetector.SimpleOnScaleGestureListener
private class ScaleListener extends
ScaleGestureDetector.SimpleOnScaleGestureListener {
@Override
public boolean onScale(ScaleGestureDetector detector) {
return false;
}
}
To know more about ScaleGestureDetector please refer following link:
To know more about ScaleGestureDetector.SimpleOnScaleGestureListener please refer following link:
This class will receive Pinch zoom event.
Step 3 Register ScaleGestureDetector class to TouchEvent method.
Here we are Overriding TouchEvent method so that TouchEvent of pinch can be detected by ScaleGestureDetector.
@Override
public boolean onTouchEvent(MotionEvent ev) {
scaleGestureDetector.onTouchEvent(ev);
return true;
}
Step 4 MainActivity.java file
Paste the following code in MainActivity.java
package com.tag.blog.androidgesturesdemo;
import android.app.Activity;
import android.graphics.Matrix;
import android.os.Bundle;
import android.view.MotionEvent;
import android.view.ScaleGestureDetector;
import android.widget.ImageView;
public class MainActivity extends Activity {
private ImageView img;
private Matrix matrix = new Matrix();
private float scale = 1f;
private ScaleGestureDetector scaleGestureDetector;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
img = (ImageView) findViewById(R.id.ivDemo);
scaleGestureDetector = new ScaleGestureDetector(this, new ScaleListener());
}
@Override
public boolean onTouchEvent(MotionEvent ev) {
scaleGestureDetector.onTouchEvent(ev);
return true;
}
private class ScaleListener extends
ScaleGestureDetector.SimpleOnScaleGestureListener {
@Override
public boolean onScale(ScaleGestureDetector detector) {
scale *= detector.getScaleFactor();
scale = Math.max(0.1f, Math.min(scale, 5.0f));
matrix.setScale(scale, scale);
img.setImageMatrix(matrix);
return true;
}
}
}
Step 5 Run Application
Launch the application in any real device. Now, use pinch zoom and see that the image zooms and shrinks as per the gesture.
Default
Zoom Out
Zoom In
I hope you find this blog post very helpful while working with Gestures Tutorial in Android. Let me know in comment if you have any questions regarding Android App Development. I will reply you ASAP.
Got an Idea of Android App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Android App Development Company in India.
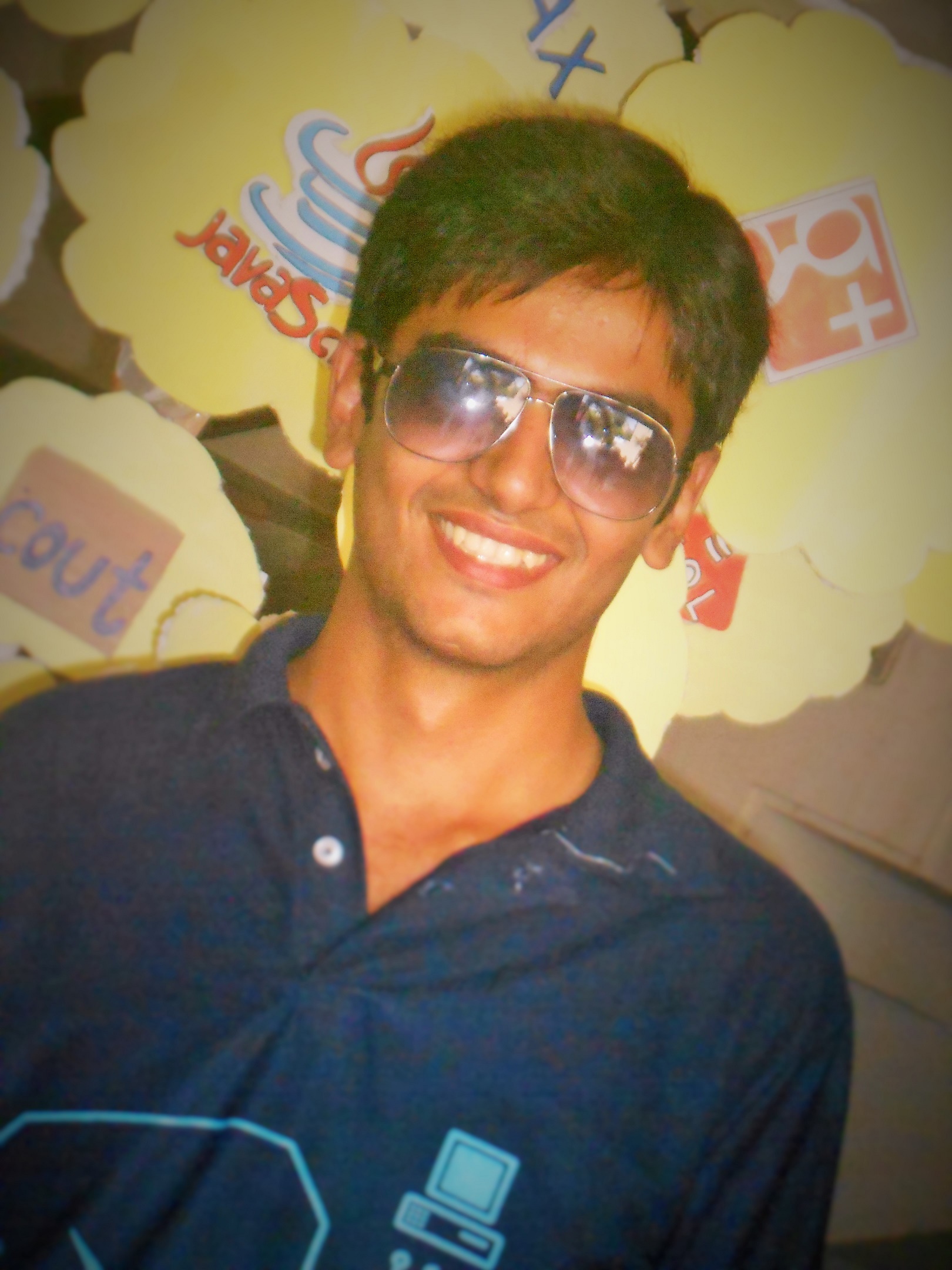
I am dedicated and very enthusiastic Android developer. I love to develop unique and creative apps and also like to learn new programing languages and technology.
Attribute In Unity
Create Custom Inspector In Unity