
Be patient..... we are fetching your source code.
Objective
The main objective of this blog post is to give you an idea about Camera Shake in Unity3D.
Are You looking for giving some real feel to your game ?
Do you want to add some screen shaking effect to your game ?
Then you are at the right place !
A great way to add some real feel to your game is camera shaking. It’s great for signifying the action and making your camera feel more real and part of game.
So, if you want to add camera shake in your game then you are at the right place.
In this blog, I will show you how to create your own camera shake effect in 3 ways.
Let’s try it with scripting first.
1. By scripting
First setup a scene like this in Unity.
Create an empty game object named Explodestar and a particle system in child to get more effective look.
Create a script named Explodestar attached it to ExplodeStar gameobject to play particle system on playing game.
Create another script named CameraShake and attach it to camera you want to give the shaking effect.
The code in the scripts is shown as below.
CameraShake.cs
using UnityEngine;
using System.Collections;
public class CameraShake : MonoBehaviour
{
public IEnumerator Shake(float duration, float magnitude)
{
Vector3 orignalPosition = transform.position;
float elapsed = 0f;
while (elapsed < duration)
{
float x = Random.Range(-1f, 1f) * magnitude;
float y = Random.Range(-1f, 1f) * magnitude;
transform.position = new Vector3(x, y, -10f);
elapsed += Time.deltaTime;
yield return 0;
}
transform.position = orignalPosition;
}
}
In this script, the coroutine is used for shaking the camera. If you don’t know how to use coroutine you can check this blog out the link is mentioned below.
Name | Method/variable/coroutine | Description |
---|---|---|
Shake() | coroutine | Coroutine used for shaking camera |
Vector3 orignalPosition | variable | To store the original position of camera |
float elapsed | variable | To Store Elapsed time |
float x | variable | To store random x value |
float y | variable | To store random y value |
ExplodeStar.cs
using UnityEngine;
using System.Collections;
public class ExplodeStar : MonoBehaviour
{
public ParticleSystem explodePartical;
public CameraShake cameraShake;
void Update()
{
if (Input.GetKeyDown(KeyCode.E))
{
explodePartical.Play();
StartCoroutine(cameraShake.Shake(0.15f, 0.4f));
}
}
}
Name | Method/variable/coroutine | Description |
---|---|---|
Update() | Unity Callback | To check whether User Presses Key E |
ParticleSystem explodePartical; | variable | To store reference of particle System |
CameraShake cameraShake; | variable | To store reference of Camera |
explodePartical.Play(); | method | To Play the particle system |
StartCoroutine(cameraShake.Shake(0.15f, 0.4f)); | method | To start coroutine for Camera Shaking |
Now go back to Unity and hit play button and press key (E).
Woooohooooo!
You can see the screen shaking .
This was simple and quick way to shake the camera.
But wait, It has some limitation. It doesn’t rotate the camera in any way and there is no way to control the roughness.
It just moves the camera every frame and using coroutine is bit clunky sometimes. So, if you want smoother camera shake this is not the way to go.
Let’s try it with another way using the animation and check if it fulfills our demands.
2. By Using Animation
First setup a scene like this in Unity.
Add animator to main camera and create 2 animations idle and shake in that animator.
In ideal animation start recording and set the camera to its original position.
And in shake animation move position of camera on every two frame as you want to shake it.
Now go to animator and create a trigger shake.
Make transition from idle to shake and uncheck the ‘has exit time’ checkbox and set transition time to 1.
Now make transition from shake to idle and set transition time to 1 but don’t uncheck has exit time.
Now make empty object named ShakeWithAnimation and add ShakeWithAnimation Script to it and has a particle system in child to see it more effective.
ShakeWithAnimation.cs
using UnityEngine;
using System.Collections;
public class ShakeWithAnim : MonoBehaviour
{
public Animator camAnim;
public ParticleSystem explodeStar;
void Update()
{
if (Input.GetKeyDown(KeyCode.A))
{
camAnim.SetTrigger("Shake");
explodeStar.Play();
}
}
}
Name | Method/variable/coroutine | Description |
---|---|---|
Update() | Unity Callback | Used for checking whether User Presses Key A |
ParticleSystem explodeStar; | variable | To store reference of particle System |
Animator camAnim; | variable | To store reference of Camera Animator |
explodePartical.Play(); | method | To Play the particle system |
camAnim.SetTrigger("Shake"); | method | To set Trigger for Camera Animation for Shaking. |
Go to the Unity and play the scene and press A.
Oh Yeah!
The screen shook again so you learned one more way to shake the camera.
But wait, all condition are fulfilled but,
what about roughness?
Was there any way to control it using animation?
Nahhhh!
Then how can we do that?
Yes, I know the same question is there in your mind.
Let’s try the last way and check it out.
3. By using plugin
First make new scene in Unity.
Now go to asset store and download the plugin EZ Camera Shake (this is recommended by me as I use this and is very good but you can use any other plug in of your choice).
After finishing downloading EZ CAMERA SHAKE, import it to your scene. The folder EZCameraShake will be added in your scene.
Add script CameraShaker of EZCameraShake to main camera and set the variables as you want.
Now create empty object and name it ExplodeHeart.
Then give particle system in child for more effective look.
Now create and add ExplodeHeart script to it.
Add EZCameraShake namespace to your script.
ExplodeHeart.cs
using UnityEngine;
using System.Collections;
using EZCameraShake;
public class ExplodeHeart : MonoBehaviour
{
public ParticleSystem explodePartical;
void Update()
{
if (Input.GetKeyDown(KeyCode.H))
{
explodePartical.Play();
CameraShaker.Instance.ShakeOnce(4f, 4f, 0.15f, 1f);
}
}
}
Name | Method/variable/coroutine | Description |
---|---|---|
Update() | Unity Callback | Used for checking whether User Presses Key H |
ParticleSystem explodePartical; | variable | To store reference of particle System |
explodePartical.Play(); | method | To Play the particle system |
CameraShaker.Instance.ShakeOnce(4f, 4f, 0.15f, 1f); | method | To call ShakeOnce Method of CameShaker Script Of EZCAmeShake passing magnitude, roughness, fadInTIme and fadeOutTime. |
Now go to Unity and play the scene and press H.
Yeah! The screen shaking was so smoother than the both the other way, wasn’t it?
By this way you created your camera shake with the plugin. But wait, does this mean that the shaking camera with the script and animation was bad idea.
Nope, instead the last way would give more load on your memory while running so if you don’t need this smoother shaking and roughness controlled camera shake the scripting one is really quick and easy.
So you have to be wise while choosing any one of the methods of camera shaking in your game according to your requirement.
Feel free to contact us if you really liked this blog. And don’t forget to share your comments below!
TheAppGuruz is a leading Mobile Game development company in India. We are proficient in Unity and we have design & developed 500+ Android and iOS mobile games for various client across the globe. If you have any game project that need professional help feel free to contact us.
We provide mobile game development services in countries like US, UK, Australia, UAE, Kuwait, Qatar, India, China, Indonesia, Singapore and European countries
Tune in to our social media for the latest on Facebook | Twitter | Linkedin | YouTube.
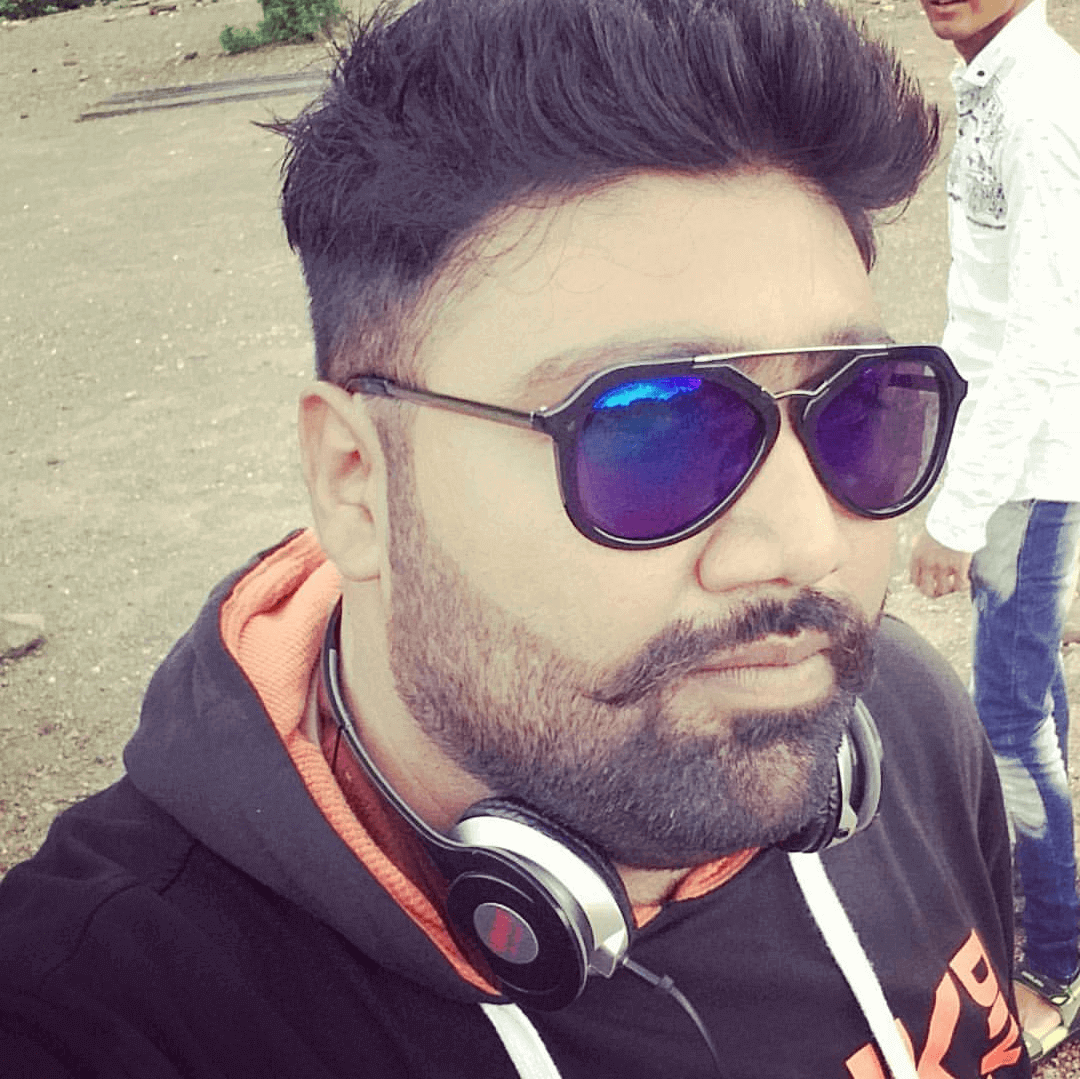
A gameholic person who is now also a Game Developer. Working in Unity3D Game Engine and making games for all platforms. Always ready to learn new things in game developing and take challenging tasks.