
Be patient..... we are fetching your source code.
Objective
The main objective of this post is to describe that how to integrate and use Social and MessageUI framework in iOS.
Introduction
Social framework provides very simple and user friendly interface by integrating with user’s social networking site accounts. It also allows to update every posts, share images, add URL and share directly through it. This frame work generally allows to share contents with social networking sites like Facebook, twitter etc.
MessageUI framework gives special facility by providing standard composer sheet for email and SMS services basically for text messaging. Basically this framework is used to provide message sending and delivery capabilities in which there is no need to leave your application.
Following demo describes how to integrate Social and MessageUI framework with your application:
Step 1 Design UI
For that you have to create one xCode project named it as ShareDemo and saved it to particular. Project has two view controller, one of them is allows you to give your choice about what you exactly want to share , other one is allows you to choose through which you want to share your stuff which allows you to choose from options like Facebook, twitter, iMessage and Email.
Step 2 Attach Frameworks
For that first you have to integrate framework with your application by adding framework from link binary with library. Now go to project, now select build Phases tab, then go to Link Binary With Library, Add framework as following.
- MessageUI.framework
- Social.framework
Step 3 Import Frameworks
Now to use various services provided by these framework import following header files in your sharing view controller.
#import
#import
Step 4 Implement Delegate Methods
Set various delegate to handle all the action related to acitivity that is performed by user or application. Following delegated you need to set.
- UINavigationControllerDelegate
- MFMessageComposeViewControllerDelegate
- MFMailComposeViewControllerDelegate
Step 5 Code for Social Connections
Now you are able to share various stuff like text, URL and images through various media, which is described one by one as following
5.1 Facebook
- (IBAction)btnFacebookClicked:(id)sender
{
[self shareFacebook];
}
Following method setup sharing with Facebook and add your image/ photo/ URL:
-(void) shareFacebook
{
SLComposeViewController *fvc = [SLComposeViewController composeViewControllerForServiceType:SLServiceTypeFacebook];
NSLog(@"%@",btnid);
if([btnid isEqualToString:@"1"])
{
[fvc setInitialText:@"Test"];
[fvc addImage:[UIImage imageNamed:@"demo.png"]];
}
else if([btnid isEqualToString:@"2"])
{
[fvc setInitialText:@"Test"];
[fvc addURL:[NSURL URLWithString:@"www.google.com"]];
}
else if([btnid isEqualToString:@"3"])
{
[fvc setInitialText:@"Text Sharing"];
}
[fvc setCompletionHandler:[self setComplitionHandlerFunction]];
if([self isInternetConnectionAvailable])
{
[self presentViewController:fvc animated:YES completion:nil];
}
else
{
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@""
message:@"Internet Connection is not available"
delegate:nil
cancelButtonTitle:@"Ok"
otherButtonTitles:nil, nil];
[alert show]; alert = nil;
}
}
-(BOOL)isInternetConnectionAvailable
{
Reachability *reachability = [Reachability reachabilityForInternetConnection];
NetworkStatus internetStatus = [reachability currentReachabilityStatus];
if (internetStatus == NotReachable) {
return NO;
} else {
return YES;
}
}
-(SLComposeViewControllerCompletionHandler)setComplitionHandlerFunction
{
SLComposeViewControllerCompletionHandler resultFB;
if([self isInternetConnectionAvailable])
{
resultFB = ^(SLComposeViewControllerResult result) {
NSString *output = @"";
switch (result)
{
case SLComposeViewControllerResultCancelled:
output = @"";
break;
case SLComposeViewControllerResultDone:
output = @"Post Successfull";
break;
default:
break;
}
if (![output isEqualToString:@""]) {
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@""
message:output
delegate:nil
cancelButtonTitle:@"Ok"
otherButtonTitles:nil, nil];
[alert show]; alert = nil;
}
};
}
return resultFB;
}
5.2 Twitter
- (IBAction)btnTwitterClicked:(id)sender
{
[self shareTwitter];
}
Following method setup sharing with Twitter and add your image/ photo/ URL:
-(void) shareTwitter
{
SLComposeViewController *tvc = [SLComposeViewController composeViewControllerForServiceType:SLServiceTypeTwitter];
if([btnid isEqualToString:@"1"])
{
[tvc setInitialText:@"Test"];
[tvc addImage:[UIImage imageNamed:@"demo.png"]];
}
else if([btnid isEqualToString:@"2"])
{
[tvc setInitialText:@"Test"];
[tvc addURL:[NSURL URLWithString:@"www.google.com"]];
}
else if([btnid isEqualToString:@"3"])
{
[tvc setInitialText:@"Text Sharing"];
}
[tvc setInitialText:@"Test"];
[tvc addImage:[UIImage imageNamed:@"demo.png"]];
[tvc setCompletionHandler:[self setComplitionHandlerFunction]];
[self presentViewController:tvc animated:YES completion:nil];
}
5.3 iMessage
- (IBAction)btniMessageClicked:(id)sender
{
[self shareiMessage];
}
Following method setup sharing with iMessage and add your image/ photo/ URL:
-(void)shareiMessage
{
MFMessageComposeViewController *controller = [[MFMessageComposeViewController alloc] init];
if([MFMessageComposeViewController canSendText])
{
if([btnid isEqualToString:@"1"])
{
[controller addAttachmentData:UIImageJPEGRepresentation([UIImage imageNamed:@"demo.png"], 1) typeIdentifier:@"image/jpg" filename:@"Image.png"];
}
else if([btnid isEqualToString:@"2"])
{
controller.body=@"www.google.com";
}
else if([btnid isEqualToString:@"3"])
{
controller.body=@"This is test";
}
controller.delegate = self;
controller.messageComposeDelegate = self;
[self presentViewController:controller animated:YES completion:nil];
}
}
-(void)messageComposeViewController:(MFMessageComposeViewController *)controller didFinishWithResult:(MessageComposeResult)result
{
switch (result) {
case MessageComposeResultCancelled:
break;
case MessageComposeResultFailed:
break;
case MessageComposeResultSent:
[self fileSendSuccessfully:@"Message sent successfully"];
break;
default:
break;
}
[self dismissViewControllerAnimated:YES completion:nil];
}
-(void)fileSendSuccessfully:(NSString *)strMessage
{
UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@""
message:strMessage
delegate:nil
cancelButtonTitle:@"Ok"
otherButtonTitles:nil, nil];
[alert show]; alert = nil;
}
5.4 Email
- (IBAction)btnEmailClicked:(id)sender
{
[self showPicker];
}
Following method setup sharing with Email and add your image/ photo/ URL:
-(void)showPicker
{
Class mailClass = (NSClassFromString(@"MFMailComposeViewController"));
if (mailClass != nil) {
if ([mailClass canSendMail]) { [self displayComposerSheet]; }
else { [self launchMailAppOnDevice]; }
} else {
[self launchMailAppOnDevice];
}
}
- (void)launchMailAppOnDevice
{
NSString *recipients = @"mailto:&subject=";
NSString *body = @"&body=";
NSString *email = [NSString stringWithFormat:@"%@%@", recipients, body];
email = [email stringByAddingPercentEscapesUsingEncoding:NSUTF8StringEncoding];
[[UIApplication sharedApplication] openURL:[NSURL URLWithString:email]];
}
- (void)displayComposerSheet
{
MFMailComposeViewController *picker = [[MFMailComposeViewController alloc] init];
picker.mailComposeDelegate = self;
[picker setSubject:@"Test"];
if([btnid isEqualToString:@"1"])
{
[picker addAttachmentData:UIImagePNGRepresentation([UIImage imageNamed:@"demo.png"]) mimeType:@"image/jpg" fileName:@"test.jpg"];
}
else if([btnid isEqualToString:@"2"])
{
[picker setMessageBody:@"www.google.com" isHTML:YES];
}
else if([btnid isEqualToString:@"3"])
{
[picker setMessageBody:@"This is demo." isHTML:YES];
}
[self presentViewController:picker animated:YES completion:nil];
}
- (void)mailComposeController:(MFMailComposeViewController*)controller didFinishWithResult:(MFMailComposeResult)result error:(NSError*)error
{
switch (result)
{
case MFMailComposeResultCancelled:
break;
case MFMailComposeResultSaved:
break;
case MFMailComposeResultSent:
[self fileSendSuccessfully:@"Mail sent successfully"];
break;
case MFMailComposeResultFailed:
break;
default:
break;
}
[self dismissViewControllerAnimated:YES completion:nil];
}
If you have got any query related to iOS - Social & MessageUI Framework then comment them below.
Got an Idea of iPhone App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best iPhone App Development Company in India.
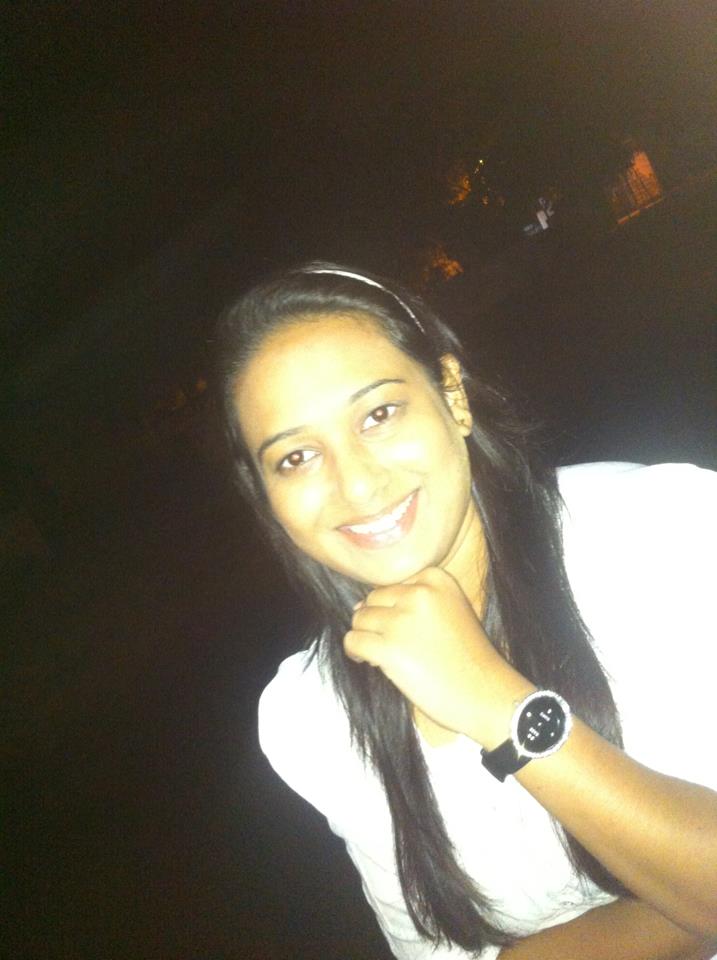
I am iOS developer, as a developer my basic goal is continue to learn and improve my development skills , to make application more user friendly.
iOS - Text Overlay on Image
iOS - Overlap Multiple Videos