Objective
Main objective of this blog post is to give you a brief idea on how to use WaitUntil & WaitWhile in your project.
Step 1 Concept of WaitUntil & WaitWhile in Unity
Getting confused with WaitUntil & WaitWhile?
Don’t know how to use WaitUntil & WaitWhile?
If that’s the case, then I’m at your service.
I assume you already know about coroutines before reading this blog post, as WaitUntil and WaitWhile works only with a yield statement in coroutines.
If not, then have a look here:
Step 2 Understand about WaitUntil
- By definition, it holds back the execution until the condition gets true.
- In other words, it will not continue the execution when our condition has a false value. Unity will wait for the condition to get a true value to continue execution.
Okay, I got the Idea. What next?
Now we’ll move on to a simple demo so that you know how and where to use it.
Say you are developing a car racing game and you want to give a notification to player as soon as the tank gets empty.
For that case, check out following code:
using UnityEngine;
using System.Collections;
public class Demo : MonoBehaviour {
public int counter;
void Start() {
counter = 20;
StartCoroutine(FuelNotification());
}
IEnumerator FuelNotification() {
Debug.Log("Waiting for tank to get empty");
yield return new WaitUntil(()=> counter <= 0);
Debug.Log("Tank Empty!"); //Notification
}
void Update() {
if (counter > 0)
{
Debug.Log("Fuel Level: " + counter);
counter--;
}
}
}
When counter becomes zero, player will get the notification. And until then console will display fuel level.
Pretty simple, isn’t it?
Yeah! But what is this mysterious “() =>” thing with our WaitUntil?
Well, I was expecting that question from you.
That thing is called A Lambda expression. (Only => part, not the parentheses)
It is an Anonymous method (another C# concept) which implicitly gets the info about the variable passed with it. It is mostly used in LINQ expressions.
Lambda expression is a very convenient tool, give it a try. See how that works here:
However, if that messes up your head, we’ve another way for doing things:
using UnityEngine;
using System.Collections;
public class Demo : MonoBehaviour {
public int counter;
void Start() {
counter = 20;
StartCoroutine(FuelNotification());
}
IEnumerator FuelNotification() {
Debug.Log("Waiting for tank to get empty");
yield return new WaitUntil(IsTankEmpty);
Debug.Log("Tank Empty!");
}
void Update() {
if (counter > 0)
{
Debug.Log("Fuel Level: " + counter);
counter--;
}
}
public bool IsTankEmpty(){
if(counter>0){ return false; }
else { return true; }
}
}
Check console; output is the same, right?
Got the point? A whole method in just one line, see how powerful a Lambda Expression is.
I hope that cleared your thoughts on WaitUntil.
Step 3 Understand about WaitWhile
- WaitWhile is the opposite of WaitUntil.
- It holds back the execution while the condition is true.
- Unlike WaitUntil, it will wait for the condition to get a false value to execute next code block.
The same example as before using WaitWhile would be as following:
using UnityEngine;
using System.Collections;
public class Demo : MonoBehaviour {
public int counter;
void Start() {
counter = 20;
StartCoroutine(FuelNotification());
}
IEnumerator FuelNotification() {
Debug.Log("Waiting for tank to get empty");
yield return new WaitWhile(()=> counter > 0);
Debug.Log("Tank Empty!");
}
void Update() {
if (counter > 0)
{
Debug.Log("Fuel Level: " + counter);
counter--;
}
}
}
As you can see, only the mechanism of dealing with conditions is different. Rest is the same.
Step 4 Conclusion
But keep in mind that using these concepts is like a double edged sword.
If your calculations are huge, this will slow down your game’s performance as these functions are called in each frame.
With this, I would like to end this post on WaitUntil & WaitWhile.
If you still find anything in this post confusing, drop a comment below.
Stay connected for more posts like this.
Learning Unity sounds fun, right? Why not check out our other Unity Tutorials?
Got an Idea of Game Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Unity 3D Game Development Company in India.
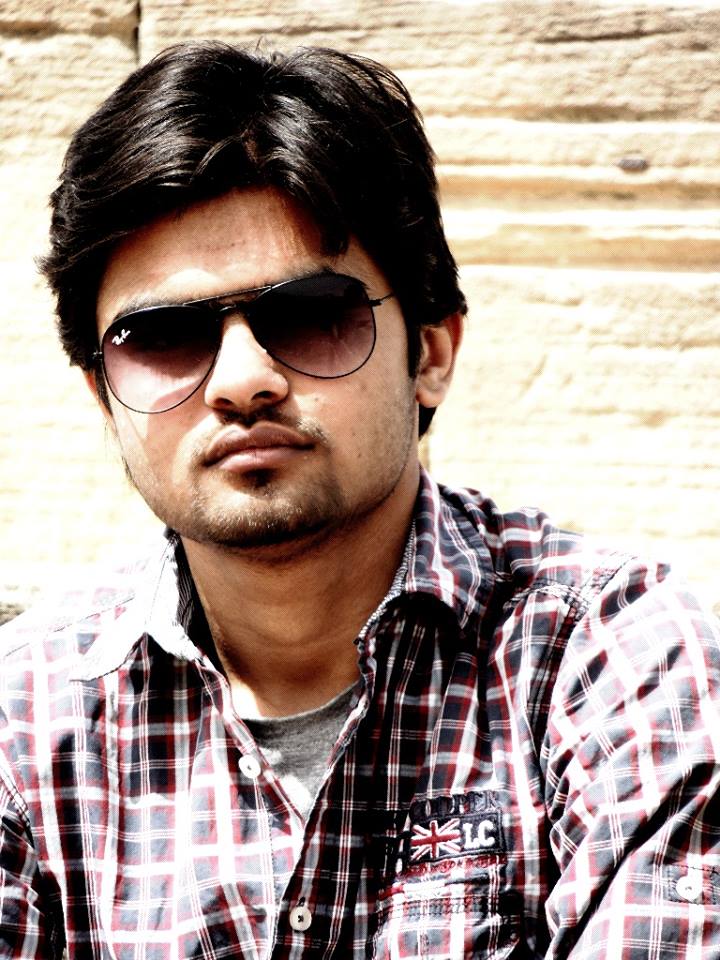
An Addictive Gamer turned into a Professional Game Developer. I work with Unity Engine. Part of TheAppGuruz Team. Ready to take on Challenging Games & increase my knowledge about Game Development.