
Be patient..... we are fetching your source code.
Objective
The main objective of Different String Manipulation post is to describe the various transform that can be applied to String in iOS.
String Manipulation in text like flips text, rotating text or generating mirror image of text is described here.
Step 1 Create XCode Project
Create xCode project named it “FlippedText” and saved it. Project has only one view controller which will become main view controller.
Step 2 Design UI
For text flipping you need one UITextField which allows to get input from user. You also need another UIView inside your main view and one UILabel (named lblTitle) inside it for displaying flipped text as an output.
Step 3 UIlabel Initialization
Write following lines of code in viewDidLoad() of main view controller.
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
UILabel *lblTitle = [[UILabel alloc] init];//
lblTitle.text = @"Flipped Text";
lblTitle.font = [UIFont systemFontOfSize:20];
[lblTitle sizeToFit];
self.navigationItem.titleView = lblTitle;
}
Step 4 UITextFieldDelegate Method
When user gives input from UITextField; below mentioned code will get executed by handling its delegate method. You can have brief idea from following method.
- (BOOL)textView:(UITextView *)textView shouldChangeTextInRange:(NSRange)range replacementText:(NSString *)text
{
if([text isEqualToString:@"\n"]) {
[textView resignFirstResponder];
// Add text in lblFlipped
lblFlipped.text = tvText.text;
[lblFlipped sizeToFit];
return NO;
}
return YES;
}
Step 5 Manipulating Text
There are basically three ways to manipulate text; code for each of this is mentioned below:
FLIP
- (IBAction)btnFlipClicked:(id)sender
{
lblFlipped.layer.transform = CATransform3DMakeRotation(M_PI, 1, 0, 0);
}
ROTATE
- (IBAction)btnRotateClicked:(id)sender
{
lblFlipped.layer.transform = CATransform3DMakeRotation(M_PI, 0, 0, 1);
}
MIRROR
- (IBAction)btnMirrorClicked:(id)sender
{
lblFlipped.layer.transform = CATransform3DMakeRotation(M_PI, 0, 1, 0);
}
ORIGINAL
- (IBAction)btnOriginalClicked:(id)sender
{
lblFlipped.layer.transform = CATransform3DMakeRotation(M_PI, 0, 0, 0);
}
Step 6 Text Screenshot
Following are the screen shots when various transform applied to text.
I hope you found this blog helpful while working on Diffrent String Manipulation or Text Manipulation in iOS. Let me know if you have any questions or concerns regarding iOS, please put a comment here and we will get back to you ASAP.
Got an Idea of iPhone App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best iPhone App Development Company in India.
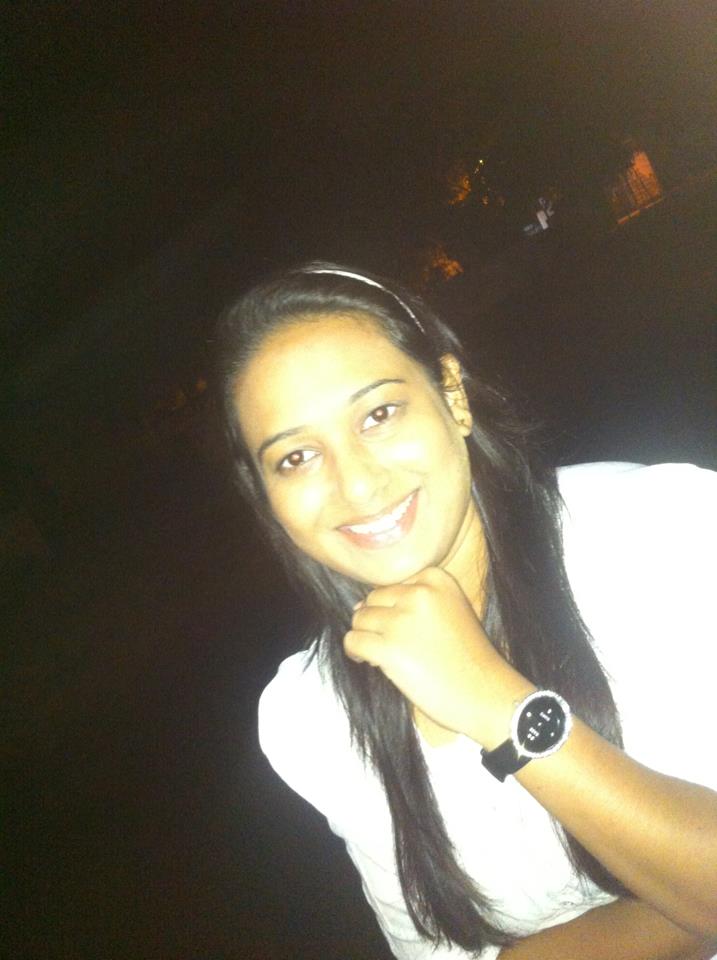
I am iOS developer, as a developer my basic goal is continue to learn and improve my development skills , to make application more user friendly.