
Be patient..... we are fetching your source code.
Objective
Main objective of this JSON Swift Tutorial is to give you an idea about Swift JSON Parser Example.
Introduction:
In this tutorial, we will create simple application called JSONDemo which describes how to parse Swift JSON data. JSON is stands for JavaScript Object Notation, JSON provides simple interface for storing and exchanging data. JSON Parsing example is very simple and easy human readable format that is used to send and receive data over network.
Array of three strings can be represented in JSON format as per the following:
- [“Example1”, "Example2”, "Example3”]
JSON representation for Student object which has three member variables named RollNumber, Name and Class can be described as per following.
{“RollNumber”: 10, “Name”: “Ram”, “Class”: 3}
Because of number of its advantages and features, nowadays it is very popular in use. It can be read in few minutes as well as many third party make web services that will return JSON formatted data when you provide appropriate query string. In creation of your own web service JSON provide easy way to convert data in JSON format before sending to another party.
First of all create a project in XCode if not created.
You can get more detail about creating XCode project from following link:
Step 1 Setup UI for Swift JSON Parsing
Set following UI in Xcode where we display JSON data after parsing. Here we take one UItableView, you can get more idea about it from following figure.
You can get more detail about UITableView from following link:
Step 2 Copy JSON file to your project repository
File named days.json contains following JSON format data. To parse JSON, we have to copy days.json file into our project repository. Here is the JSON that we will be parsing.
{
"MONDAY": [
{
"TITLE": "TEST DRIVEN DEVELOPMENT",
"SPEAKER": "JASON SHAPIRO",
"TIME": "9:00 AM",
"ROOM": "MATISSE",
"DETAILS": "EXPLORE THE TOPICS AND TOOLS RELATED TO TEST DRIVEN DEVELOPMENT."
},
{
"TITLE": "JAVA TOOLS",
"SPEAKER": "JIM WHITE",
"TIME": "9:00 AM",
"ROOM": "ROTHKO",
"DETAILS": "DISCUSS THE LATEST SET OF TOOLS USED TO HELP EASE SOFTWARE DEVELOPMENT."
}
],
"TUESDAY": [
{
"TITLE": "MONGODB",
"SPEAKER": "DAVINMICKELSON",
"TIME": "1: 00PM",
"ROOM": "PICASSO",
"DETAILS": "LEARNABOUT\"NOSQL\"DATABASES."
},
{
"TITLE": "DEBUGGINGWITHXCODE",
"SPEAKER": "JASONSHAPIRO",
"TIME": "1: 00PM",
"ROOM": "VANGOGH",
"DETAILS": "EXPLOREDIFFERENTPATTERNSFORDEBUGGINGYOURIOSAPPS."
}
],
"WEDNESDAY": [
{
"TITLE": "SCRUM MASTER",
"SPEAKER": "DAVIN MICKELSON",
"TIME": "1:00 PM",
"ROOM": "MATISSE",
"DETAILS": "LEARN THE ROLES AND RESPONSIBILITIES OF A SCRUM MASTER"
},
{
"TITLE": "DESIGN PATTERNS",
"SPEAKER": "JIM WHITE",
"TIME": "1:00 PM",
"ROOM": "ROTHKO",
"DETAILS": "APPLY BEST PRACTICES AND SOUND ARCHITECTURES TO SOFTWARE DESIGN."
}
]
}
You can also use JSON data from web link url. In our demo we are using JSON from the following link:
Step 3 Line of Code that Safely Parse JSON
Following method describes how to convert JSON file into NSData. Then pass that data object in NSJSONSerialization method JSONObjectWithData:options:error. That will return array or dictionary as per JSON format.
func jsonParsingFromFile()
{
let path: NSString = NSBundle.mainBundle().pathForResource("days", ofType: "json")!
let data : NSData = try! NSData(contentsOfFile: path as String, options: NSDataReadingOptions.DataReadingMapped)
self.startParsing(data)
}
Following method describes how to convert JSON file into NSData for web url JSON data:
func jsonParsingFromURL () {
let url = NSURL(string: "http://theappguruz.in//Apps/iOS/Temp/json.php")
let request = NSURLRequest(URL: url!)
NSURLConnection.sendAsynchronousRequest(request, queue: NSOperationQueue.mainQueue()) {(response, data, error) in
self.startParsing(data!)
}
}
startParsing() method looks like:
func startParsing(data :NSData)
{
let dict: NSDictionary!=(try! NSJSONSerialization.JSONObjectWithData(data, options: NSJSONReadingOptions.MutableContainers)) as! NSDictionary
for var i = 0 ; i < (dict.valueForKey("MONDAY") as! NSArray).count ; i++
{
arrDict.addObject((dict.valueForKey("MONDAY") as! NSArray) .objectAtIndex(i))
}
for var i = 0 ; i < (dict.valueForKey("TUESDAY") as! NSArray).count ; i++
{
arrDict.addObject((dict.valueForKey("TUESDAY") as! NSArray) .objectAtIndex(i))
}
for var i = 0 ; i < (dict.valueForKey("WEDNESDAY") as! NSArray).count ; i++
{
arrDict.addObject((dict.valueForKey("WEDNESDAY") as! NSArray) .objectAtIndex(i))
}
tvJSON .reloadData()
}
Step 4 Set delegate and data source for UITableView
Following line of code shows how to set delegate and data source for table view. In ViewController.swift file write following line of code.
class ViewController: UIViewController,UITableViewDataSource,UITableViewDelegate
Step 5 Create Custom cell class for UITableViewCell
Following line of code describes how to create custom cell named TableViewCell.swift for table view and set its property accordingly.
class TableViewCell: UITableViewCell
{
@IBOutlet weak var lblTitle: UILabel!
@IBOutlet weak var lbDetails: UILabel!
init(style: UITableViewCellStyle, reuseIdentifier: String) {
super.init(style: style, reuseIdentifier: reuseIdentifier)
// Initialization code
}
override func awakeFromNib() {
super.awakeFromNib()
// Initialization code
}
override func setSelected(selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
// Configure the view for the selected state
}
}
Step 6 UITableView delegate methods
Following are the table view delegate methods that display parsed JSON formatted data. Following line of code you have to put in ViewController.swift file.
func numberOfSectionsInTableView(tableView: UITableView) -> Int
{
return 1
}
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int
{
return arrDict.count
}
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell
{
let cell : TableViewCell! = tableView.dequeueReusableCellWithIdentifier("Cell") as! TableViewCell
let strTitle : NSString=arrDict[indexPath.row] .valueForKey("TITLE") as NSString
let strDescription : NSString=arrDict[indexPath.row] .valueForKey("DETAILS") as NSString
cell.lblTitle.text=strTitle
cell.lbDetails.text=strDescription
return cell as TableViewCell
}
Step 7 viewDidLoad() method
Now, use one global variable for JSON data type it’s JSONFile or JSONUrl. for that put below line:
let yourJsonFormat: String = "JSONFile"
Here,
- JSONFile: JSON data from file.
- JSONUrl: Data from Web link url.
Now, call jsonParsinFromURL() and jsonParsinFromFile() method from viewDidLoad() method based on JsonFormat which code looks like:
override func viewDidLoad()
{
super.viewDidLoad()
if yourJsonFormat == "JSONFile" {
jsonParsingFromFile()
} else {
jsonParsingFromURL()
}
}
iOS Simulator Screenshot:
I hope you find this blog is very helpful while working with Swift JSON Parsing Example. Let me know in comment if you have any question regarding Swift Application Development please comment here. I will reply you ASAP.
Got an Idea of iPhone App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best iPhone App Development Company in India.
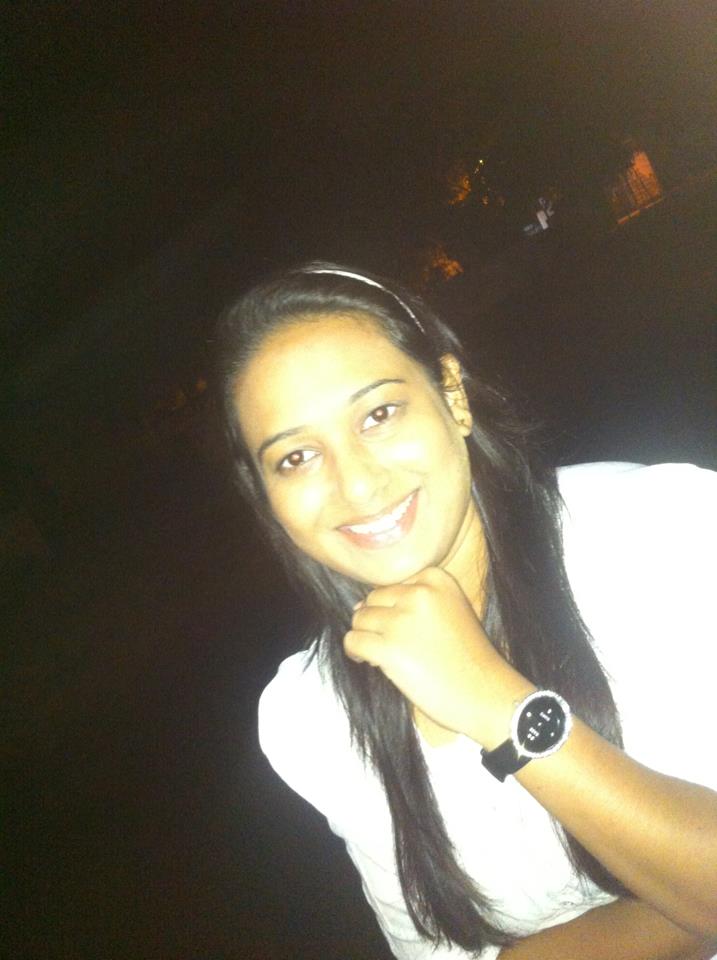
I am iOS developer, as a developer my basic goal is continue to learn and improve my development skills , to make application more user friendly.
iOS | Create a Playground Area for Swift Programming
Create UITableView Control in iOS