Objective
The main objective of this post is to show you how to implement motion sensing mechanism in your game using Accelerometer in Unity.
Step 1 Introduction to Accelerometer
Remember the all time famous game Temple Run? We can switch lanes by just tilting our phone.
Many Racing games are also based on these motion sensors like Highway Rider and Asphalt.
Another example would be any flight simulator game.
And yes one of my all time favorites iStunt-2 !!
So how does that work?
How to implement a motion detection mechanism in Unity?
- The answer to these questions is Acceleronmeter.
What's an Accelerometer?
- To keep it short: an accelerometer is a small chip in our smart phones which detects the motion changes in phone.
Let's see how we can get data from the accelerometer in Unity.
Don't worry, its a simple script.
We just have to use Input.acceleration to fetch data from accelerometer.
Just like we get position from transform.position.
See a simple Demo here:
using UnityEngine;
using System.Collections;
public class AccelerometerInput : MonoBehaviour {
void Update () {
transform.Translate(Input.acceleration.x, Input.acceleration.y, 0);
}
}
I've used only the Input.acceleration.x & Input.acceleration.y component to get the cube moving.
Pretty easy, right?
Get directions from Input.acceleration and translate the object using them directly.
Well, using those inputs directly is not preferable.
In most cases you will find your player or object going crazy in the game with no sense of any direction.
So what do we do now?
We need to do some pre-processing before using them for any kind of input.
Let me give you a small sample of such pre-processing:
public class Controller : MonoBehaviour
{
public float smooth = 0.4f;
public Text inputValue;
public float newRotation;
public float sensitivity = 6;
private Vector3 currentAcceleration, initialAcceleration;
void Start()
{
initialAcceleration = Input.acceleration;
currentAcceleration = Vector3.zero;
}
void Update () {
//pre-processing
currentAcceleration = Vector3.Lerp(currentAcceleration, Input.acceleration - initialAcceleration, Time.deltaTime/smooth);
newRotation = Mathf.Clamp(currentAcceleration.x * sensitivity, -1, 1);
transform.Rotate(0, 0, -newRotation);
}
}
As you can see I've done some tweaking with Input.acceleration instead of direct using it.
Yes, that's pre-processing:
- Tweaking and adjusting input values until you get your desired result.
Step 2 Conclusion
I hope you can use accelerometer inputs in your game efficiently now and create some awesome stuff with it.
If you still have any doubt about this, drop a comment and I'll be there to set you in motion again. ;)
Learning Unity sounds fun, right? Why not check out our other Unity Tutorials?
Got an Idea of Game Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Unity 3D Game Development Company in India.
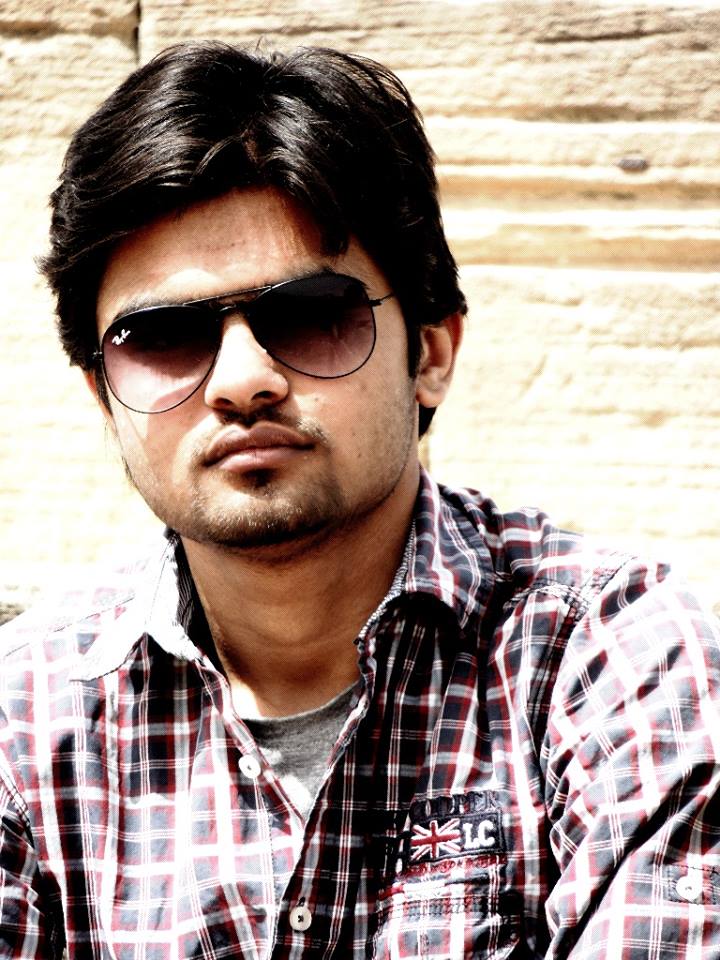
An Addictive Gamer turned into a Professional Game Developer. I work with Unity Engine. Part of TheAppGuruz Team. Ready to take on Challenging Games & increase my knowledge about Game Development.