
Be patient..... we are fetching your source code.
Objective
The main objective of this blog post is to explain how to do CSV parsing in unity.
Step 1 Introduction
1.1 CSV File
What is CSV file?
CSV stands for comma separated values and sometimes it is also called character separated values. CSV file is used to store data (in table structure format) in plaintext separated by comma (or any other character). The extension of CSV file is .csv. CSV file can contain number of records and each record can have multiple fields. Generally records are separated by line break character and fields are separated by comma or other character. It is a plaintext file and so it is very easy to share data on web or between two different applications.
1.2 Advantages of CSV
The basic advantages of using CSV format are,
- Primary use of CSV file is to store record based data (like student details, product details, customer details, etc.)
- CSV file is used to transfer data between two incompatible systems.
- Mostly CSV file is used to store sequential records where each record has identical set of fields.
- CSV files are used for data exchange because it is common for all computer operating systems and is supported by all spreadsheets and database management systems.
Step 2 CSV File Example
Following is the example of CSV file where comma (,) is used to distinguish each field.
Roll No,Name,Marks
1,Name1,70
2,Name2,60
3,Name3,80
Example:
The following C# code demonstrate the use of CSV Parsing.
Note:
- Create an empty game object and insert the following script in it.
- Place .csv file in Assets folder.
2.1 Screenshots
1) When project is initialized, .csv file is read and following data is displayed.
2) Now let’s enter some dummy data in Input Fields to add new record
3) When you click on ADD button, new record will be added to the CSV file.
2.2 CSVParsing Script
using UnityEngine;
using System.Collections;
using UnityEngine.UI;
using System.IO;
public class CSVParsing : MonoBehaviour
{
public TextAsset csvFile; // Reference of CSV file
public InputField rollNoInputField;// Reference of rollno input field
public InputField nameInputField; // Reference of name input filed
public Text contentArea; // Reference of contentArea where records are displayed
private char lineSeperater = '\n'; // It defines line seperate character
private char fieldSeperator = ','; // It defines field seperate chracter
void Start ()
{
readData ();
}
// Read data from CSV file
private void readData()
{
string[] records = csvFile.text.Split (lineSeperater);
foreach (string record in records)
{
string[] fields = record.Split(fieldSeperator);
foreach(string field in fields)
{
contentArea.text += field + "\t";
}
contentArea.text += '\n';
}
}
// Add data to CSV file
public void addData()
{
// Following line adds data to CSV file
File.AppendAllText(getPath() + "/Assets/StudentData.csv",lineSeperater + rollNoInputField.text + fieldSeperator +nameInputField.text);
// Following lines refresh the edotor and print data
rollNoInputField.text = "";
nameInputField.text = "";
contentArea.text = "";
#if UNITY_EDITOR
UnityEditor.AssetDatabase.Refresh ();
#endif
readData();
}
// Get path for given CSV file
private static string getPath(){
#if UNITY_EDITOR
return Application.dataPath;
#elif UNITY_ANDROID
return Application.persistentDataPath;// +fileName;
#elif UNITY_IPHONE
return GetiPhoneDocumentsPath();// +"/"+fileName;
#else
return Application.dataPath;// +"/"+ fileName;
#endif
}
// Get the path in iOS device
private static string GetiPhoneDocumentsPath()
{
string path = Application.dataPath.Substring(0, Application.dataPath.Length - 5);
path = path.Substring(0, path.LastIndexOf('/'));
return path + "/Documents";
}
}
I hope you find this blog is very helpful while working with CSV Parsing in Unity. Let me know in comment if you have any questions regarding Unity. I will reply you ASAP.
Got an Idea of Game Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Game Development Company in India.
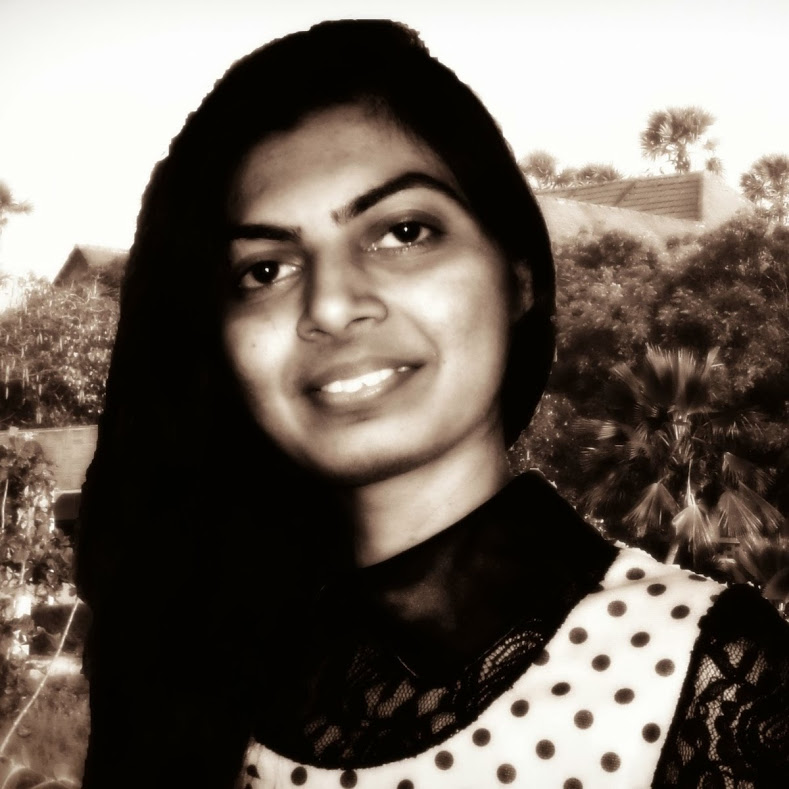
I am professional Game Developer, developing games in cocos2d(for iOS) and unity(for all platforms). Games are my passion and I aim to create addictive, high quality games. I have been working in this field since 1 year.
Pixel Tile Set
Dynamic Scroll View in Unity 4.6 UI