
Be patient..... we are fetching your source code.
Objective
The main objective of this blog post is to show how to do XML Parsing in unity.
Step 1 XML
XML (eXtensible Markup Language) is a markup language which is designed to transport and store data.
XML is most common tool for data transmission between applications.
XML data is stored in plain text format that provides a software and hardware independent way of storing data.
XML is very useful when we want to exchange data between incompatible systems over network.
Step 2 Use Of XML
Here are basic uses of XML:
- As Database: XML is really an interesting file that we can use as database in which we can store a set of data.
- Web Publishing: XML allows the developers to save the data into XML files and the use the XSLT transformation API's to generate the content in required format such as HTML, XHTML or WML.
- Web Searching: In our application we can use the XML data and then the search the data from the XML file and display to the user. Similar data can be displayed in different format to the user.
- Data transfer: We can use XML to save configuration or business data for our application. The XML file can be stored, used, transferred to another program.
- e-business applications: XML is ideal solution for Electronic Data Interchange (EDI).
- Metadata applications: In many applications, XML file is used as Metadata.
- Create other Languages: Many languages are created using XML. Examples are WML, MathML etc.
- Use in Databases: Modern database such as Oracle and MSSQL server is providing the XML support in their databases.
Step 3 XML Document
XML documents use a self-describing and simple syntax. First line in xml document is XML declaration; it defines XML version and encoding type. Second line describes the root element (Players) of the document and next line is its child element(Player).
<!--?xml version=”1.0” encoding=”UTF-8”?-->
Player1
10
3.1 Different Entities
Basically XML Document has following entities:
XML Tags | In XML document, we can define our own tags, which are case sensitive. There must be opening as well as closing tag in same case and all tags must be properly nested. |
XML Elements | An XML element is consist of an opening tag, its attributes, any content and closing tag. In short XML element is everything from its start tag to its end tag. |
XML Attributes | An XML element can have attributes that provide additional information about element. Attribute values must always be quoted. Attributes cannot contain multiple values and tree structure. |
XML Node | A node is a part of the hierarchical structure that makes up an XML document. Node is a generic term that applies to any type of XML document object, including elements, attributes, comments and plain text. |
XML Parsing | XML Parsing means reading the XML file/string and getting its content according to the structure to use them in a program. And a program or software that reads XML files and makes information is called XML Parser. In unity, for xml parsing in C#, System.Xml namespace is used. There are many ways for XML Parsing; here it has done using XmlDocument and XmlElement classes. |
XmlDocument Class | The XmlDocument class provides support for the Document Object Model (DOM). This class represents the entire XML document as an in-memory node tree, and it permits both navigation and editing of the document. There are various ways to use the XmlDocument class. By using its different properties and methods, we can travel to tree and make changes. |
XmlElement Class | XmlElement class is based on a class named XmlLinkedNode that itself is based on XmlNode. To access an XML element, we can declare a variable of type XmlElement but the main purpose of this class is to get an element from a DOM object. For this reason, the XmlElement class doesn't have a constructor you can use. |
Step 4 Example
The following simple c# code demonstrate the XMLParsing with the help of XmlDocument and XmlElement classes.
Note
- Create empty game object and add following script on it.
- Place the .xml file in Assets >> Resources folder.
Screenshots:
1) When XML file is initially read, following data will be displayed.
2) By tapping Create Element button, element will be added as shown below.
3) By tapping Modify Element button, elements will be modified as shown below.
4.1 XMLParsing Script
using UnityEngine;
using System.Collections;
using System.Xml;
using System.Collections.Generic;
public class XMLParsing : MonoBehaviour
{
public TextMesh fileDataTextbox;
private string path;
private string fileInfo;
private XmlDocument xmlDoc;
private WWW www;
private TextAsset textXml;
private List<Player> Players;
private string fileName;
// Structure for mainitaing the player information
struct Player
{
public int Id;
public string name;
public int score;
};
void Awake()
{
fileName = "DemoXmlFile";
Players = new List<Player>(); // initalize player list
fileDataTextbox.text = "";
}
void Start ()
{
loadXMLFromAssest();
readXml();
}
// Following method load xml file from resouces folder under Assets
private void loadXMLFromAssest()
{
xmlDoc = new XmlDocument();
if(System.IO.File.Exists(getPath()))
{
xmlDoc.LoadXml(System.IO.File.ReadAllText(getPath()));
}
else
{
textXml = (TextAsset)Resources.Load(fileName, typeof(TextAsset));
xmlDoc.LoadXml(textXml.text);
}
}
void Update()
{
// Following code just check whether any button is touched on mouse down
if(Input.GetMouseButtonDown(0))
{
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
RaycastHit hit;
if(Physics.Raycast(ray, out hit) && hit.collider != null)
{
if(hit.collider.name.Equals("ModifyXmlButton"))
modifyXml();
else if(hit.collider.name.Equals("CreateElementButton"))
createElement();
}
}
}
// Following method reads the xml file and display its content
private void readXml()
{
foreach(XmlElement node in xmlDoc.SelectNodes("Players/Player"))
{
Player tempPlayer = new Player();
tempPlayer.Id = int.Parse(node.GetAttribute("id"));
tempPlayer.name = node.SelectSingleNode("name").InnerText;
tempPlayer.score = int.Parse(node.SelectSingleNode("score").InnerText);
Players.Add(tempPlayer);
displayPlayeData(tempPlayer);
}
}
private void displayPlayeData(Player tempPlayer)
{
fileDataTextbox.text += tempPlayer.Id + "\t\t" + tempPlayer.name + "\t\t" + tempPlayer.score + "\n";
}
// Following method will be called by tapping ModifyXml button
// It just multiply player's score by 10
private void modifyXml()
{
fileDataTextbox.text = "";
foreach(XmlElement node in xmlDoc.SelectNodes("Players/Player"))
{
int nodeId = int.Parse(node.GetAttribute("id"));
Player tempPlayer = Players[nodeId-1];
tempPlayer.score *= 10;
Players[nodeId-1] = tempPlayer;
node.SelectSingleNode("score").InnerText = tempPlayer.score + "";
displayPlayeData(tempPlayer);
}
xmlDoc.Save(getPath()+".xml");
}
// Following method create new element of player
private void createElement()
{
Player tempPlayer = new Player();
tempPlayer.Id = Players.Count+1;
tempPlayer.name = "Player"+tempPlayer.Id;
tempPlayer.score = tempPlayer.Id*10;
Players.Add(tempPlayer);
displayPlayeData(tempPlayer);
XmlNode parentNode = xmlDoc.SelectSingleNode("Players");
XmlElement element = xmlDoc.CreateElement("Player");
element.SetAttribute("id",tempPlayer.Id.ToString());
element.AppendChild(createNodeByName("name",tempPlayer.name));
element.AppendChild(createNodeByName("score",tempPlayer.score.ToString()));
parentNode.AppendChild(element);
xmlDoc.Save(getPath()+".xml");
}
private XmlNode createNodeByName(string name, string innerText)
{
XmlNode node = xmlDoc.CreateElement(name);
node.InnerText = innerText;
return node;
}
// Following method is used to retrive the relative path as device platform
private string getPath(){
#if UNITY_EDITOR
return Application.dataPath +"/Resources/"+fileName;
#elif UNITY_ANDROID
return Application.persistentDataPath+fileName;
#elif UNITY_IPHONE
return GetiPhoneDocumentsPath()+"/"+fileName;
#else
return Application.dataPath +"/"+ fileName;
#endif
}
private string GetiPhoneDocumentsPath()
{
// Strip "/Data" from path
string path = Application.dataPath.Substring(0, Application.dataPath.Length - 5);
// Strip application name
path = path.Substring(0, path.LastIndexOf('/'));
return path + "/Documents";
}
}
For any query or suggestions please comment below. Click here to Download demo from github.
Got an Idea of Game Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Game Development Company in India.
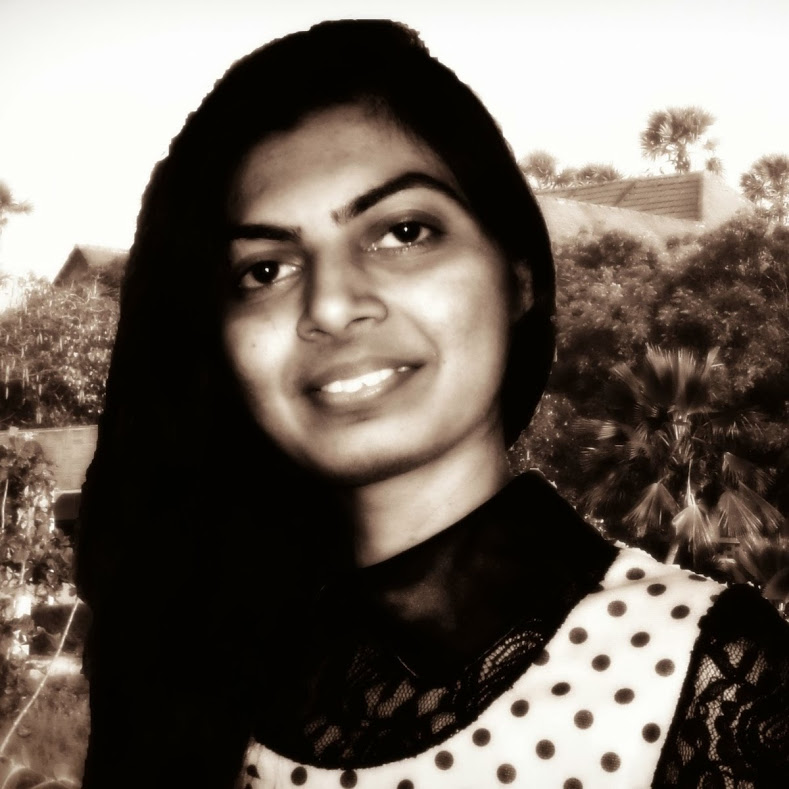
I am professional Game Developer, developing games in cocos2d(for iOS) and unity(for all platforms). Games are my passion and I aim to create addictive, high quality games. I have been working in this field since 1 year.