
Be patient..... we are fetching your source code.
Objective
This blog post teaches you to make simple iPhone app in Swift Programming Language and in what way to use Objective C file with the Swift Project. You can make the Objective C file and also import from any folder of your Swift Project.
To make you think easier follow the step by step process to get started and go through for creating application and successfully run it.
Step 1 Install and Setting Up Your Development Environment
Download and install Xcode. Xcode6.0 Beta is an Integrated Development Environment (IDE) to create all iPhone apps with Swift Programming Language but it requires OS X 10.9.3 or later to be installed. It contains a suite for software development tools for iOS application. After Installation Xcode; open Xcode.
The following screen will appear:
To create a new Xcode project select option Create a new Xcode project. It appears a Choose a template for your project dialog box as shown in given figure.
From the dialog box select Single View Application option and press next button.
It appears following screen:
The above screen contains number of fields:
- Product Name
- Organization Name
- Company Identifier
- Bundle Identifier
- Class Prefix
User has to provide basic information into specific field, which is very helpful to create a new project. Here, make sure that your Language must be Swift for this application project. Also it includes list of devices for which you wa nt to develop application iPhone/iPad. If you want to develop an application for both then simply select option Universal. After giving appropriate application name press next button.
It appears directory dialog box as following screen:
Select the directory for your new project from directory dialog box where exactly you want to save your application and select Create Button.
Then following screen will appear:
Above screen allow you to select the supported orientations, build and release settings. One of the fields is very important say Deployment Target. It means that what is the earliest OS that you want to run this application? If you set deployment target to iOS 6, you must have iOS 6 to install and run the application.
Step 2 How to run your project
Now select Simulator (Top Left corner) from the drop down, which is near Run (denoted in following screenshot) button and select run.
Following figure will gives you brief idea:
Step 3 List of main files of your project
The main files are given below screen that is generated when you have created your application. By implementing code in following files you can perform various operations to the application.
They are listed as given below:
Step 4 Create Objective-C (.h & .m file) file
Now, create one Objective-C file that you want to use in Swift Project. For these given below Screenshots are shown how to Create Objective-C file:
1) Right click on Project and Click New File:
2) Select Objective-C file and Click Next:
3) Give that File name ObjectiveCFile and create it:
4) After Clicking Create button XCode ask for configure an Objective-C bridging header. You must say YES. The given dialogue box shown only once when first Objective-C file creates in Swift Project:
5) Now, your project Navigation looks like below:
6) Here, ObjectiveCFile.m is implementation file for Objective-C file execution but ObjectiveCFile.h header file must exists for both .m & .h file execute in Objective-C. So, now make header file:
7) Choose Header file and click next:
8) Give file name; make sure that your filename must be same as a .m file name which is previously created:
9) Now, Finally create both you .m & .h file for Objective-C code execution, your project navigation looks like:
Step 5 Code for .m & .h file which is blank
Now, Write a Code below the comment section in ObjectiveCFile.h header file:
#import <UIKit/UIKit.h>
@interface ObjectiveCFile : UIViewController
- (void)displayMessageFromCreatedObjectiveCFile;
@end
Here, create one method displayMessageFromCreateObjectiveCFile which have return type void.
Now, these header file import in implementation file and make some stuffs for method which is implemented in ObjectiveCFile.m and write code as below:
#import <Foundation/Foundation.h>
#import "ObjectiveCFile.h"
@interface ObjectiveCFile ()
@end
@implementation ObjectiveCFile
- (void)viewDidLoad {
[super viewDidLoad];
}
- (void) displayMessageFromCreatedObjectiveCFile {
NSLog(@"Hello. . . ");
NSLog(@"This Message From Created Objective-C File with Swift Project");
}
@end
Here, in method displayMessageFromCreateOBjectiveCFile we can simply print the message for checking file is working or not.
Step 6 Connect this Objective-C file with Swift
For use this .m & .h files, we can connect the Objective-C files to the Swift with the help of Bridging-Header file. In this project, Bridging-Header file is ObjectiveCWithSwiftDemo-Bridging-Header and for any project its name like projectName-Bridging-Header In Bridging-Header file import header file of Objective-C which is ObjectiveCFile.h which looks like:
//
// Use this file to import your target's public headers that you would like to expose to Swift.
//
#import "ObjectiveCFile.h"
Step 7 Create object of Objective-C Class in Swift & call the method
Now, in. swift file, we can create a direct object of the Objective-C class for use their methods. For that in our project, open ViewController.swift file and write a code in viewDidLoad() method:
import UIKit
class ViewController: UIViewController {
override funcviewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
let objObjectiveCFile = ObjectiveCFile()
objObjectiveCFile.displayMessageFromCreatedObjectiveCFile()
}
override funcdidReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
Now, with the help of Step 2 of 7 build & run the project or for shortcut press cmd+b for build & cmd+r for run the project.
Wow, its build successfully. Now see the Log bar for output:
Successfully print Objective-C method in the Swift Project.
You can also, import the existing Objective-C file in the Project. For those file you can only import the header file in Bridging-Header file. Which is shown in below steps:
1) Right click & Add Files to...
2) Choose your folder & select both .h & .m file make sure that checkbox of Copy Items if needed is checked and click Add.
3) After importing file, your project navigation looks like:
4) Now, in Bridging-Header file, one header ObjectiveCFile.h already imported so, we can import another file with name AnotherObjectiveCFile.h which we want to use with Swift Project:
//
// Use this file to import your target's public headers that you would like to expose to Swift.
//
#import "ObjectiveCFile.h"
#import "AnotherObjectiveCFile.h"
5) Now in ViewController.swift file, write code in viewDidLoad method for accessing AnotherObjectiveCFile’smethod, which is shown in below:
//
// ViewController.swift
// ObjectiveCWithSwiftDemo
//
// Created by TheAppGuruz-iOS-103 on 01/08/14.
// Copyright (c) 2014 myOrganizationName. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
override funcviewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
//let objObjectiveCFile = ObjectiveCFile()
//objObjectiveCFile.displayMessageFromCreatedObjectiveCFile()
let objAnotherObjectiveCFile = AnotherObjectiveCFile()
objAnotherObjectiveCFile.displayMessageFromImportedObjectiveCFile()
}
override funcdidReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
6) AnotherObhectiveCFile.h& AnotherObjectiveCFile.m is looks like: AnotherObjectiveCFile.h file is:
#import <UIKit/UIKit.h>
@interface AnotherObjectiveCFile : UIViewController
- (void)displayMessageFromImportedObjectiveCFile;
@end
and AnotrherObjectiveCFile.m file is:
#import <Foundation/Foundation.h>
#import "AnotherObjectiveCFile.h"
@interface AnotherObjectiveCFile ()
@end
@implementation AnotherObjectiveCFile
- (void)viewDidLoad {
[super viewDidLoad];
}
- (void) displayMessageFromImportedObjectiveCFile {
NSLog(@"Hello. . . ");
NSLog(@"This Message From Imported Objective-C File with Swift Project");
}
@end
7) Now, Build & Run the project, it gives output like:
So, finally we can use the Objective-C file with the help of “Bridging-Header” file in Swift Project. We can Create or Import Objective-C file in Swift.
I hope you found this blog helpful while Integrating Objective C file in Swift. Let me know if you have any questions or concerns regarding iOS 8, please put a comment here and we will get back to you ASAP.
Got an Idea of iPhone App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best iPhone Application Development Company in India.
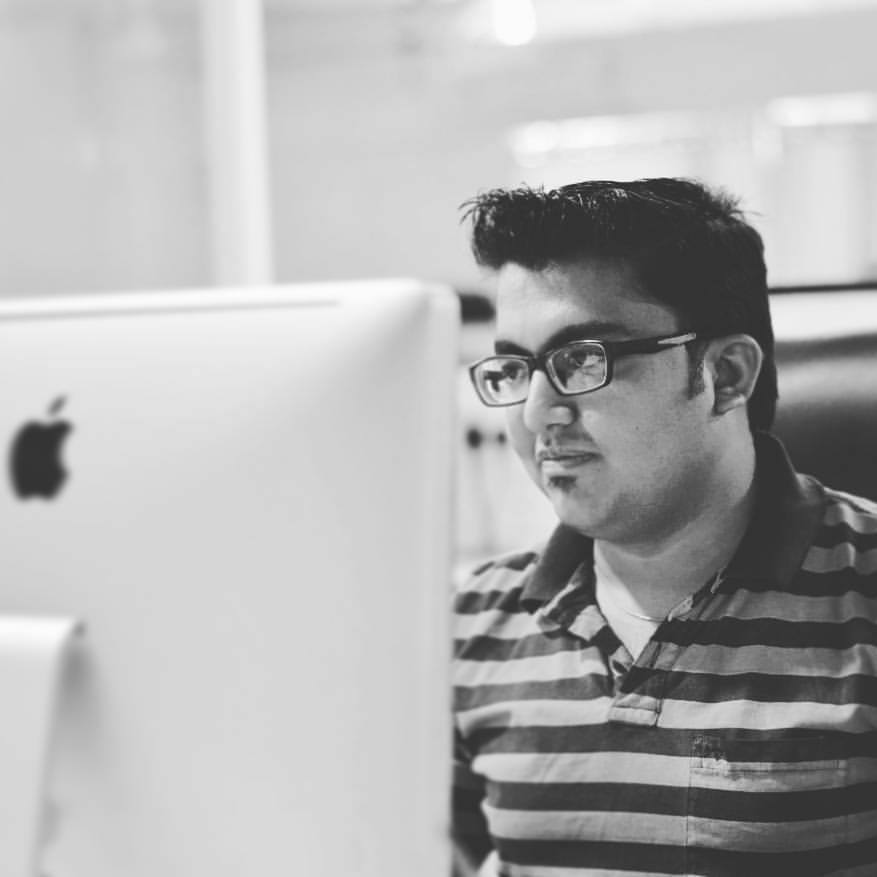
I am iOS Application Developer & I personally believed that When a programming language is created that allows programmers to program in simple English, it will be discovered that programmers cannot speak English.
Create Custom Keyboard for iOS8
Table Tennis 3D Live Ping Pong