
Be patient..... we are fetching your source code.
Objective
The main objective of this blog post is to explain how to implement Google Sign in SDK in your iOS application.
Step 1 Introduction
Google SignIn SDK provides following features:
- Allow a user to log in through Google account.
- Get user’s profile information.
In this blog post, I will explain how a user can log into our application through their Google account.
First of all, you need ClientID for Google Sign in, so you must have a google account.
Step 2 Get Google SignIn Client ID
To use the Google Sign in, we need Google Sign in Client ID.
Google Sign in Client ID is an ID through which we can make calls to Google API from our iOS Application.
Follow these steps to get Client ID for your app:
- Sign into Google Developers Console using your Google Account.
- Create Application if you haven’t created it yet. If an application is already created, choose your application.
Add your application bundle identifier. If it’s already added then choose it.
Choose your country/region and click on Continue button.
- Select Google service you want to use.
Select Google Sign-in and click on ENABLE GOOGLE SIGN-IN button to enable the Google sign in service.
- When you click on Enable button, it will enable Google Sign in for your application.
- When you successfully enabled Google Sign-in for your application, click on Continue button to generate configuration files
- Then you will be redirected to download the configuration file.
Click on Download GoogleService-info.plist button to download the configuration file. The configuration file will be downloaded, we will use it later on at the time of project creation.
Step 3 Project Configuration
Now, create a new Xcode project.
- If you don’t know how to create a new project in Xcode, you can check the link given below to get more details on Sign into Creating Xcode Project.
- To integrate Google Sign-in, I would be using Google Sign-in SDK
- Add GoogleService-Info.plist to the root of your Xcode project
- A new window appears to choose the options for adding files. Check Copy items if needed checkbox and click on Finish button.
- Setup URL Types
You need to setup URL Type to handle the callback from Google.- Locate GoogleService-Info.plist
- Copy REVERSED_CLIENT_ID
- Select your project from the Navigator
- Select your app from Project and Target List
- Go to Info and expand URL Types
- Click on + button to add URL Type
- Paste REVERSED_CLIENT_ID to URL Schemes
- Add another URL Type by clicking on + button
- Paste your project Bundle Identifier
3.1 Add Other Frameworks
Google Sign in SDK needs several other frameworks. Such as
- StoreKit.framework
- AddressBook.framework
- SystemConfiguration.framework
To add these frameworks, select your project in Project Navigator and click on Build Phases.
Expand Link Binary With Libraries and click on + button.
Choose and add the above-listed frameworks.
3.2 Add Bridging Header
As GoogleSignIn.framework is available in Objective-C, we need to add a Bridging header to our Swift project. By using Bridging Header, we can use Objective-C code in our Swift application.?
Add following line of code to Bridging Header file:
#import <GoogleSignIn/GoogleSignIn.h>
Step 4 Sign in Using Google
- Open the AppDelegate.swift file and use following lines of code in the application(_didFinishLaunchingWithOptions:) method.
func application(application: UIApplication, didFinishLaunchingWithOptions launchOptions: [NSObject: AnyObject]?) -> Bool { // Initialize sign-in GIDSignIn.sharedInstance().clientID = "<YOUR_CLIENT_ID_HERE>" GIDSignIn.sharedInstance().delegate = self return true }
In this method, I would be using my API key to Integrate Google Sign-in SDK in my iOS application.
You have to set your application Client ID in.
<YOUR_CLIENT_ID_HERE>
- Add your AppDelegate.swift class to the subclass of GIDSignInDelegate.
class AppDelegate: UIResponder, UIApplicationDelegate, GIDSignInDelegate
- Open AppDelegate.swift and add following lines of code in the application(_openURL:, _options:) method.
func application(application: UIApplication, openURL url: NSURL, options: [String: AnyObject]) -> Bool { return GIDSignIn.sharedInstance().handleURL(url, sourceApplication: options[UIApplicationOpenURLOptionsSourceApplicationKey] as! String, annotation: options[UIApplicationOpenURLOptionsAnnotationKey]) }
This method handles the URL that your application receives at the end of the application process.
If your application will run on iOS 8 or older version, add the application(_sourceApplication:, _annotation) deprecated method.
func application(application: UIApplication, openURL url: NSURL, sourceApplication: String?, annotation: AnyObject?) -> Bool { return GIDSignIn.sharedInstance().handleURL(url,sourceApplication: sourceApplication,annotation: annotation) }
- Add following GIDSignInDelegate() methods in AppDelegate.swift
func signIn(signIn: GIDSignIn!, didSignInForUser user: GIDGoogleUser!, withError error: NSError!) { if (error == nil) { // Perform any operations on signed in user here. let userId = user.userID // For client-side use only! let idToken = user.authentication.idToken //Safe to send to the server let name = user.profile.name let email = user.profile.email let userImageURL = user.profile.imageURLWithDimension(200) // ... } else { print("\(error.localizedDescription)") } }
This method will be called when a user successfully signs into his/her account. You can get the user’s basic profile information in this method.
func signIn(signIn: GIDSignIn!, didDisconnectWithUser user:GIDGoogleUser!, withError error: NSError!) { // Perform any operations when the user disconnects from app here. }
This method will be called when a user disconnects from Google account.
- Add Google Sign-in Button so that user can do the sign-in process.
- Make your ViewController to the subclass of GIDSignInUIDelegate.
class ViewController: UIViewController, GIDSignInUIDelegate
- Add a following line of code to the viewDidLoad() method of your ViewController.
GIDSignIn.sharedInstance().uiDelegate = self
- Add UIView to your storyboard
- Select the view that you have added and add GIDSignInButton as a custom class
- In your ViewController, declare an IBOutlet
@IBOutlet weak var signInButton: GIDSignInButton!
- Connect IBOutlet with UIView that you have created in storyboard
- Make your ViewController to the subclass of GIDSignInUIDelegate.
You cannot see anything in the UIView in the storyboard even if you have added GIDSignInButton as a custom class. But when you will run the application Google Sign-in button will appear in the view at run time.
Google Sign-in SDK automatically handles the sign-in process. When you tap on that button it will ask the user to enter the credential for his/her Google account.
Step 5 Sign out from Google account
Call the following method to sign out a user from their Google account.
GIDSignIn.sharedInstance().signOut()
I hope you will find this blog post helpful while working with Google SignIn SDK in iOS. Let me know in a comment if you have any questions regarding Google Sign in iOS. Please put a comment here and we will get back to you ASAP.
Got an Idea of iPhone App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best iPhone App Development Company in India.
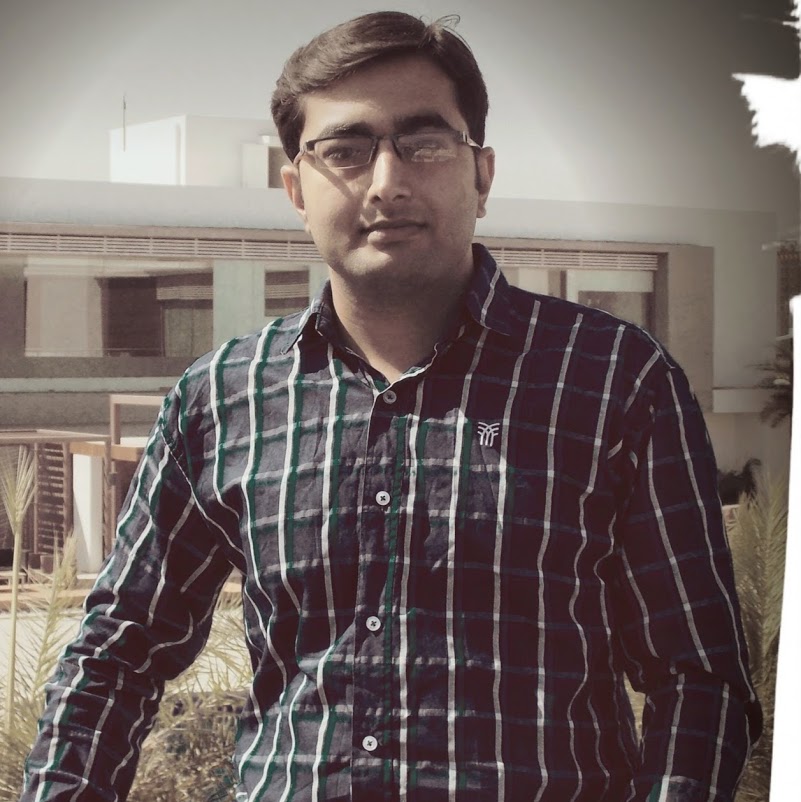
I am 2D Game developer and iOS Developer with an aspiration of learning new technology and creating a bright future in Information Technology.
The Programming Nomenclature