- Introduction
- Reasons for emphasis on following Coding Standards
- Reflective Examples
- Benefits
- The golden rules
- Rule 1 – Better Naming
- Rule 2 – Things to avoid while naming
- Don’t use names that can have multiple meanings
- Don't use similar names
- Avoid using single-letter variable names
- Don’t name a variable arbitrarily
- Don’t use the names which are confusing
- Don’t use Number-series
- Avoid noise words
- Avoid type encoding
- Avoid mental mapping
- Avoid using the same word for two purposes
- Avoid Clever naming
- Rule 3 – What things to include while naming
- Takeaway
Objective
The main objective of this blog post is to give you an idea about Coding Standards (Naming Conventions)
Step 1 Introduction
It’s now, in the final semester of my 4-year Computer Engineering degree course, that I realise that all along my teachers, from day one, tried to instil in us the habit to write codes independently. They wanted us to become self-taught programmers.
While continuous and fast learning is a crucial skill that companies seek in job candidates, I feel if my classmates and I had been introduced to CODING STANDARDS before we started programming, there would have been a lot lesser gap between the skills companies expected from us as freshers and the skills we actually have.
In this blog series, I will try to bridge the gap between these two skill sets. I’ll also share with you the secrets of a becoming a great coder, keeping some very rudimentary yet highly important principles in mind while you write your next awesome code!
1.1 An insight of your current style of coding
Ask yourself these Questions:
- If you are programming all by yourself, look at the names you give to variables, methods, classes, objects, properties etc. Are the names relevant to the context of the definition of the program?
- If, on the other hand, you are working in a team, does your coding partner or a team member understand the code you wrote easily, without your help?
If the answer to either of the two questions, is an "NO", you’ll be shocked to know that your coding style is flawed, if you go by the industry standard.
Step 2 Reasons for emphasis on following Coding Standards
"I'm just a beginner, I should care more about technical stuff, why should I care about naming conventions?"
While it may sound OK to assign all sorts of weird names to identifiers when you are working alone, it is NOT OK when you start working in a team.
Here are the reasons for you to become more stricter with your coding style.
2.1 Working alone vs working in team
When you start working for a company, you will be working as a part of a team. The project will be much larger than anything you’ve done in your college. You will be a part of a team consisting of designers, testers, other developers etc. There will be many situations where you will be refactoring, debugging or seeking help from your teammates and showing your code to them.
When your teammates look at the code you wrote, they should need practically no explanation as why you named a certain identifier the way you did. (You won’t have time to explain every single name in Corporates, deadlines are taken very seriously.) So every name you use must reflect the purpose and the person reviewing the code must be able to understand it instantly.
2.2 More Lines Of Code.
While you are working on a project you will be dealing with a lot more LOC(Lines of Code) than you’ve ever handled during your college. You will write and have to deal with hundreds and thousands of lines of code. And as uncle Ben would quote "With more power comes great responsibility", I would like to say, "With more LOC come a great number of errors and bugs".
This is where debugger tools will come to your rescue. But only if while writing the code you were careful enough to keep identifiers of the program identifiable. The debugger will only display error and let you execute selective lines of code but will not display the intent of the particular code(or a part of it).
The names should help you locate the particular LOC or at least, the region of code which is causing the problem.
Step 3 Reflective Examples
Here’s an example to start with. It is from one of the first most books will give to begin teaching the syntax. For example,
You are reading about variables( in a JAVA book ) and you understood the syntax of any programming language concept:
Class class_name {
public static void main(String[] args) {
data_type variable_name ; //value not assigned
data_type variable_name = value ;
}
}
And the example is something as follows:
Does it really tell you anything about the object type the above class resembles? No, it doesn’t tell you anything. Or does the names ch or x represent anything? No, Hence it is best suited to a book but has practically no use in the real world out there.
Here's an example of a method name:
int func1(int x , int y) {
//Statements ...
}
Does the name of the function func1 tell you what it is about to perform? No. It is syntactically correct but its purpose is not evident.
The only thing you can assume(by seeing the return type of function and data types of the arguments) is that some operations would be performed on x and y and something would be returned. But which specific operation will it perform? Addition, subtraction, division, multiplication or something else? You can’t figure it out.
Actually, the code was for a function that adds up two numbers.
Check out the naming style below:
int add (int number1, int number2) {
//Logic for adding 2 numbers
}
You see how quickly you could understand what the function is supposed to do? Here is what I came across when I googled:
And it is downright hilarious !!!
Step 4 Benefits
The following are the benefits of following STANDARD naming conventions:
- Reduces the effort needed to read and understand the source code.
- Provides additional information (i.e., metadata) about the use an identifier is put to.
- Helps formalise expectations and promote consistency within a development team.
- Enhances clarity in cases of potential ambiguity.
- Improves the aesthetic and professional appearance of work product (For example, by disallowing overly long names, comical or cute names, or abbreviations)
- Helps avoid naming collisions that might occur when the work product of different organisations is combined.
- Provides meaningful data to be used in project handovers which require submission of program source code and all relevant documentation.
- Aids better understanding in case of code reuse, especially after a long interval of time.
- Makes the process of integration of new requirements easier.
- Ensures a better understanding of the developers and analysts as to what the overall system is trying to do.
Step 5 The golden rules
If you want to be a standout at naming things in your code and get an edge over other job seekers in the market try to follow these rules as closely as possible. Remember to implement their rules while you are coding. You'll become better only if you practice these rules regularly.
5.1 Rule 1 Better Naming
The names of variables, functions or classes should give you a clear idea about their respective functions and their usage in the program.
Example 1:
Suppose we want to name a variable that indicates radius of the circle then the correct names would be:
double radius;
//OR
double radiusofCircle;
While the wrong names may look like:
double r;
double R;
double rad; //Incomplete names are not allowed.
double Radius; //Variable names should begin with lowercase.
Example 2:
Can you guess what this code is doing? Lets, see the below Picture.
Take time to study the function carefully and once done, move forward. Now, just rename the method and the variables and you can experience the magic. Here we are coding for the minesweeper game and we need to write a function for getting the list of all the flagged cells (cell is an array of integer. The array of cells forms the entire board). Then does the method defined below reveal its behaviour?
Here by only renaming theList as gameBoard, list1 as flaggedCells and x as cell makes the method a lot easier to understand. The name of the method getFlaggedCells gives the idea about the method's function. STATUS_VALUE and FLAGGED variables specify the significance of zeroth index of the cell[] and the value for the flagged cell respectively.
5.2 Rule 2 - Things to avoid while naming
5.2.1 Don’t use names that can have multiple meanings
For Example:
int hp;
This variable name hp can mean a hypotenuse variable or height of a picture variable. So, it's better we use hypotenuse or pictureHeight instead of hp.
5.2.2 Don't use names that are similar (which vary in very small ways.)
For Example:
Consider two variables employee and employees, which are the same, except for the last letter. This kind of differences is very hard to spot and often lead to subtle bugs, which are even harder to find during code reviews. So avoid using such names.
5.2.3 Avoid using single-letter variable names
For Example:
int l,r,o,p; // CANNOT BE USED TO REPRESENT INTENT
[single-letter names can ONLY be used as local variables inside short methods ONLY IF NECESSARY or can be used as loop counters]
5.2.4 Don’t name a variable arbitrarily
Just because you can’t use the same name to refer to two different things in the same scope.
Consider, for example, the practice of creating a variable named kool just because the name cool was used for something else.
5.2.5 Don’t use the names which are confusing
You can’t understand clearly what it does later on:
- getActiveAccount()
- getActiveAccounts()
- getActiveAccountInfo()
Check the above-mentioned methods. How can we decide which method is to be called and when it is to be called?
5.2.6 Don’t use Number-series (a1, a2)
This function reads much better when source and destination are used in the place of a1 and a2 respectively for the argument names.
5.2.7 Avoid noise words
(a, an, the). public static void copyChars(char a1[], char a2[])
Noise words are another meaningless distinction, which don’t serve any purpose. Imagine that you have a Product class. If you have another class called ProductInfo or ProductData, you have made the names different without making them mean anything different. Info and Data are indistinct noise words like a, an, and the.
Note that there is nothing wrong with using prefixes conventions like a and the as long as they make a meaningful distinction. For example, you might use a for all local variables and the for all function arguments. The problem comes in when you decide to call a variable zork because you already have another variable named zork.
5.2.8 Avoid type encoding
String phoneNumberString;
Here the problem occurs when the type of the variable is changed and the variable name is not changed. E.g. PhoneNumber becomes long double instead of String.
5.2.9 Avoid mental mapping
For us clarity is king.
Consider the following declaration:
int r; // lowercase version of the url with host & //scheme removed
Here, every time the programmer comes across r in the code further, he has to remember that r is the lowercased version of the URL with the host and scheme removed.
5.2.10 Avoid using the same word for two purposes
If you follow the “one word per concept” rule, you could end up with many classes that have, for example, an add method. Now suppose we have many classes where add will create a new value by adding or concatenating two existing values.
Suppose now we are writing a new class that has a method that puts its single parameter into a collection, should we call this method add? It might seem consistent because we have so many other add methods, but in this case, the semantics are different, so we should use a name like insert or append instead.
5.2.11 Avoid Clever naming, avoid using Synonyms.
If names are too clever, they will be memorable only to people who share the author’s sense of humour, and only as long as these people remember the joke. e.g. don’t use the name like augment() or join() to mean add().
5.3 Rule 3 - What things to include while naming
5.3.1 Always use pronounceable names.
Use:
private Date modificationTimestamp;
Instead of:
private Date modyts; //WRONG
5.3.2 Use searchable names
One might easily be able to find MAX_CLASSES_PER_STUDENT, but finding the number 7 for the same could be more troublesome. Searches may turn up the digit as part of file names, other constant definitions, and in various expressions where the value is used with a different intent.
Besides, when someone else is reviewing your source code then it will be very easy for him to grasp the intent of your code if you have used searchable names.
COMPARE:
TO:
Note that sum, above, is not a particularly useful name but, at least, is searchable, but consider how much easier it will be to find WORK_DAYS_PER_WEEK than to find all the places where 5 was used and filter the list down to just the instances with the intended meaning.
5.3.3 Classes and objects should have a noun or noun phrase.
While names like MyClass, UserClass, ClassA, Class1 are already bad names for classes, avoid using names like Manager, Processor, Data, or Info. Do not use partial names and abbreviations. The class name is the representation of the similar type of objects and thus, should be named accordingly. Good examples of class name are Customer, WikiPage, Account, AddressParser and Animal. The first letter of the class should be capital.
Although an Object is an instance of a class, avoid using instance1, instance2 or object1, object2. If possible give unique names to objects. For example you can use Panda, Zebra, Lion etc. For the Animal class instead of animal1, animal2.
Avoid using a verb for a class name.
5.3.4 Methods should have a verb or verb phrase
Method's name should reveal the intent of the method in context of the program(that is, which procedure the method will carry out.) Good examples are postPayment, deletePage, or savePage instead of more generalised post, delete and save respectively.
- If the method is returning something it is good and acceptable to prefix the method name with the get.
- If the method is changing and assigning the value of a variable, property etc. Then it is prefixed by set.
- Make good use of common verb e.g. is, has, can or do.
Examples:
String name = employee.getName();
customer.setName("mike");
if (paycheck.isPosted()) {
//Statements;
};
if(isRaining){
bringUmbrella();
}
5.3.5 Try to add meaningful context
Imagine that you have variables named firstName, lastName, street, houseNumber, city, state, and zip-code. Taken together it’s pretty clear that they form an address. But what if you just saw the state variable being used alone in a method? Would you automatically infer that it was part of an address?
You can add context by using prefixes: addrFirstName, addrLastName, addrState, and so on. At least, readers will understand that these variables are part of a larger structure. A better solution is to create a class named Address.
Consider the following example for better understanding of this case:
Variables with unclear context:
Variables with clear context:
Step 6 Takeaway
Though naming conventions are one of the fundamental aspects of programming, it's often overlooked. Don’t be satisfied at the first go, always try to read your code and strive for more meaningful names, which are clear and reveal the intent.
I hope this blog post is helpful to you while coding in any programming language. Let me know in comments below if you have any questions regarding The Programming Nomenclature. I will try my best to reply.
Got an Idea of The Programming Nomenclature? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Game Development Company in India
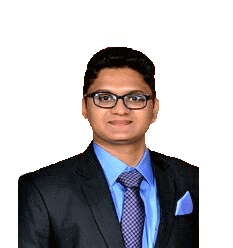
I am a passionate and adroit Android Programmer. I like to learn new technologies and contribute new ideas and updates to an existing project. I enjoy Blogging too :). Solving problems logically always fascinates me. Always happy to help and always ready to answer your questions, stay tuned for my new blog posts.
Integrate Pushwoosh SDK In iOS