
Be patient..... we are fetching your source code.
Objective
Main objective of this blog post is to give you an idea about how to Create Audio Player in iOS.
To play audio file in iOS application you have to add audio player in application. For that you have to use AVAudioPlayer class. To use AVAudioPlayer class you have to add AVFoundation framework in your application.
Lets create a demo application, which use AVAudioPlayer.
Step 1 Create XCode Project
Open Xcode and create new project with single view application template as shown in below figure.
In the next step enter AudioPlayerDemo as a product name and also select Objective-C as a language as shown in below figure.
Step 2 Design UI
Add a button with title "Play" and other button with title “Stop”. Define action method for both buttons. Also add slider that allows to set volume of audio player. Also defile action method for volume slider.
Step 3 Add Framework and Audio file
Add audio file, which you want to play from your application in application bundle. In this example, sound.mp3 audio file added in application bundle.
Add AVFoundation framework to your application. Go to build phase -> Link binary with library -> click add button -> select AVFoundation.framework from list and add.
Step 4 Initialize AVAudioPlayer object
Import AVFoundation framework in your viewcontroller.h file. Declare AVAudioPlayer object as shown below.
#import <UIKit/UIKit.h>
#import <AVFoundation/AVFoundation.h>
@interface ViewController : UIViewController
{
AVAudioPlayer *audioPlayer;
}
@end
In viewDidLoad method initialize audioplayer object with sound.mp3 file url. Also declare NSError object to handle error.
NSError *error;
audioPlayer = [[AVAudioPlayer alloc] initWithContentsOfURL:[NSURL URLWithString:[[NSBundle mainBundle] pathForResource:@"sound" ofType:@"mp3"]] error:&error];
if (error) {
NSLog(@"Error : %@", [error localizedDescription]);
} else {
[audioPlayer prepareToPlay];
}
Step 5 Play and Stop Audio
In action method of play button call play method of AVAudioPlayer object, to play sound.mp3 file.
- (IBAction)btnPlayClicked:(id)sender
{
[audioPlayer play];
}
In action method of stop button call stop method of AVAudioPlayer object, to stop audio. Before calling stop method check if AVAudioPlayer object is initialized or not.
- (IBAction)btnStopClicked:(id)sender
{
if (audioPlayer) {
[audioPlayer stop];
}
}
Step 6 Adjust Volume of Audio Player
In action method of volume slider set audio player volume. You can set audio player volume between 0 to 1. When slider value changes set its value as audio player volume. Before set volume of audio player check AVAudioPlayer object is initialized.
- (IBAction)sliderVolumeChanged:(id)sender
{
if (audioPlayer) {
audioPlayer.volume = [(UISlider *)sender value];
}
}
I hope you will find this blog post very useful. While working with Audio Player in iOS. Let me know in comment if you have any questions regarding in IOS. I will reply you ASAP
Got an Idea of iPhone App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best iPhone App Development Company in India.
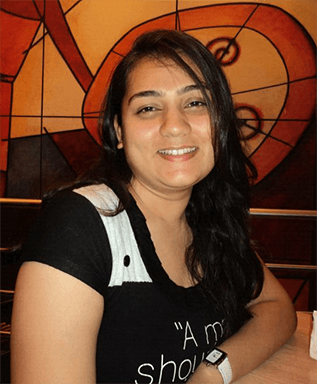
I am iOS developer with an aspiration of learning new technology and creating a bright future in Information Technology.
MapKit for User Location in iOS
Basecamp API using Laravel in Web