
Be patient..... we are fetching your source code.
Objective
The main goal of this tutorial is to get current user location using GPS device when you move.
Introduction
The given example just shows you the basics concepts of how to add GPS capability to find user’s current location in your iPhone and update the user’s current location.
The CoreLocation framework provides necessary objective-c interfaces to get user’s current location. You can see different classes used for finding the current location information below:
- CLLocationManager: An object of this class provides you the location data. It tracks large or small changes in user’s current location with given degree of accuracy. Below are some of its most usefulmethods :
- stopUpdatingLocation : It stops the generation of location updates.
- StartUpdatingLocation: This method is used to start the generation of updates that report the user’s current location.
Some important properties are given below:
-
- desiredAccuracy : This property defines the accuracy of the location data.
- distanceFilter : It defines the minimum distance the device must move horizontally before update is generated.
- CLGeocoder: This class provides the functionality to convert the coordinates into user-friendly representation. A user-friendly representation of coordinates consists of the name of the city, state, country etc.. One of its most useful methods is described below:
- reverseGeocodeLocation:completionHandler: : This method is used to submit the location data to the geocode server asynchronously.This method take a latitude and longitude value and return the result in user-readable format. This result is returned through the CLPlacemark object.
- reverseGeocodeLocation:completionHandler: : This method is used to submit the location data to the geocode server asynchronously.This method take a latitude and longitude value and return the result in user-readable format. This result is returned through the CLPlacemark object.
- CLPlacemark: An object of this class stores the placemark data for a given latitude and longitude. Placemark data includes user-readable format such as country, state, city and street address that belongs to the specified coordinates. Some of its useful properties are given below:
- name: The name of the placemark.
- ocean: Ocean name associated with the placemark.
- postalCode: The postal code associated with placemark.
- thoroughfare: The street address associated with the placemark.
- locality: The city associated with the placemark.
- ISOcountryCode: The abbreviated country name.
- country: The country associated with the placemark.
All of the above properties are read-only. Now let’s see how to perform this task in your application.
Step 1 Create Xcode Project
First of all create an XCode project named LocationFinderDemo and save it. This project will contain one ViewController.
Step 2 Add Framework
Add CoreLocation framework to your project. You can follow the below steps to add this framework to your project.
- Highlight the application target and click on build phases tab.
- Expand the Link with Binary Libraries section and click the + button.
- Finally choose the CoreLocation framework from the given list of frameworks.
Step 3 Import Framework
Go to "ViewController.h" file and modify it as shown below:
#import<UIKit/UIKit.h>
#import<CoreLocation/CoreLocation.h>
@interfaceViewController : UIViewController
CLLocationManager *locationManager;
@end
Step 4 Create Object of Framework
Now go to "ViewController.m" file. Create objects of CLPlacemark and CLGeocoder.
@interfaceViewController ()
{
CLGeocoder *geocoder;
CLPlacemark *placemark;
}
@end
Step 5 Initialized CLLocationManager
Now initialize and setup the instance of CLLocationManager and all other objects in ViewController implementation. Call startUpdatingLocation on your location manager.
- (void)viewDidLoad
{
[superviewDidLoad];
geocoder = [[CLGeocoderalloc] init];
if (locationManager == nil)
{
locationManager = [[CLLocationManageralloc] init];
locationManager.desiredAccuracy = kCLLocationAccuracyNearestTenMeters;
locationManager.delegate = self;
}
[locationManagerstartUpdatingLocation];
}
Step 6 CLLocationManager Delegate Method
Now implement the delegate methods of the CLLocationManager.
-(void)locationManager:(CLLocationManager *)manager didUpdateLocations:(NSArray *)locations {
CLLocation *newLocation = [locations lastObject];
[geocoderreverseGeocodeLocation:newLocationcompletionHandler:^(NSArray *placemarks, NSError *error) {
if (error == nil&& [placemarkscount] >0) {
placemark = [placemarkslastObject];
NSString *latitude, *longitude, *state, *country;
latitude = [NSStringstringWithFormat:@"%f",newLocation.coordinate.latitude];
longitude = [NSStringstringWithFormat:@"%f",newLocation.coordinate.longitude];
state = placemark.administrativeArea;
country = placemark.country;
} else {
NSLog(@"%@", error.debugDescription);
}
}];
// Turn off the location manager to save power.
[managerstopUpdatingLocation];
}
- (void)locationManager:(CLLocationManager *)manager didFailWithError:(NSError *)error
{
NSLog(@"Cannot find the location.");
}
Screen Shots:
If you have got any query related to using GPS device get Current Location in iOS in comment them below.
Got an Idea of iPhone App Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best iPhone App Development Company in India.
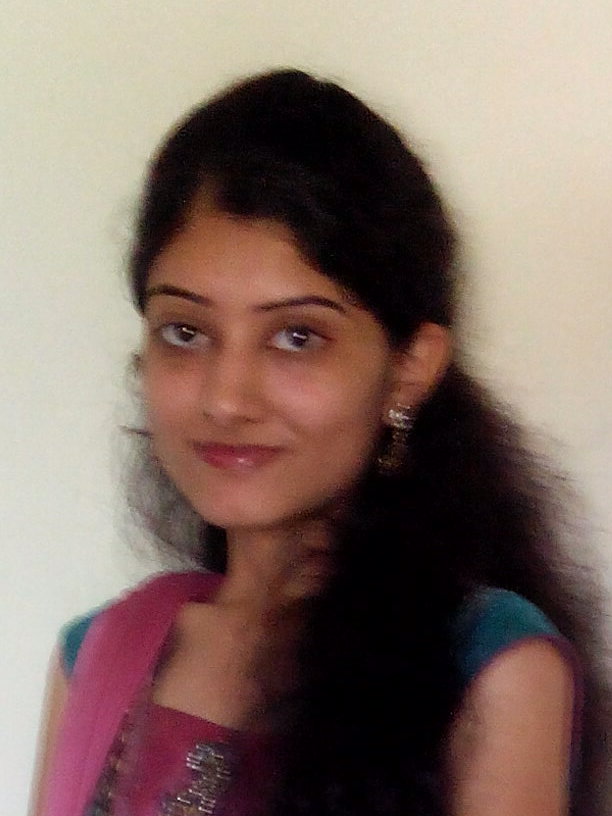
I am iOS Developer. I like to learn new technologies. I believe that any fool can write code that a computer can understand but good programmers write code that humans can understand.
Ultimate iOS File Management Tips
iOS - Lazy Loading Images