
Be patient..... we are fetching your source code.
Objectives
Main objective of this blog post is to explain how to rotate character according to surface/base. This blog post is very useful to the developer that does not know anything about character controller.
This problem is divided into two major segments.
- Character Movement
- Character Rotation According to Surface/Base.
Step 1 Character Movement
Import Character Model into Unity3D.
Add Character Controller Component to that model as shown in figure.
1.1 Code Sample:
public float speed = 6.0F;//Character Movement Speed.
public float jumpSpeed = 8.0F;//Character jump Speed.
public float gravity = 20.0F;//Gravity(We have to apply gravity manualy in character controller component).
private Vector3 moveDirection = Vector3.zero;//Character Movement Direction.
void Update () {
MoveCharacter ();
}
//Method For Character Movement
void MoveCharacter()
{
CharacterController controller = GetComponent();// Get Character Controller Component
if (controller.isGrounded)//Check Whether The Character is In Air or not
{
moveDirection = new Vector3(Input.GetAxis("Horizontal"), 0, Input.GetAxis("Vertical")); // Get Input From User
moveDirection = transform.TransformDirection(moveDirection);//Specify The Character Movement Direction in world space.
moveDirection *= speed;//Add Movement Speed To Character.
if (Input.GetButton("Jump"))//Check For JUMP Button.
moveDirection.y = jumpSpeed;//Make Character JUMP.
}
moveDirection.y -= gravity * Time.deltaTime; //Move Character Down Because Of Gravity That We have assign before.
controller.Move(moveDirection * Time.deltaTime);//Move The Character in specific Direction.
}
1.2 Apply Script
Add this script to character. This is how MoveCharacter method works.
- First get the character controller component to the controller object.
- Check whether character is grounded or not.
- If character is grounded then it will get input from user for movement and assign it to moveDirection object.
- Converts moveDirection into world space using TransformDirection.
- Add character movement speed to moveDirection.
- Move the character in specific direction using controller.Move()
Step 2 Character Rotation
Character Rotation According To Surface / Base Code Sample
2.1 Code Sample
RaycastHit hit;//For Detect Sureface/Base.
Vector3 surfaceNormal;//The normal of the surface the ray hit.
Vector3 forwardRelativeToSurfaceNormal;//For Look Rotation
// Update is called once per frame
void Update () {
CharacterFaceRelativeToSurface ();
}
//Method For Correct Character Rotation According to Surface.
private void CharacterFaceRelativeToSurface()
{
//For Detect The Base/Surface.
if (Physics.Raycast(transform.position, -Vector3.up, out hit, 10))
{
surfaceNormal = hit.normal; // Assign the normal of the surface to surfaceNormal
forwardRelativeToSurfaceNormal = Vector3.Cross(transform.right, surfaceNormal);
Quaternion targetRotation = Quaternion.LookRotation(forwardRelativeToSurfaceNormal, surfaceNormal); //check For target Rotation.
transform.rotation = Quaternion.Lerp(transform.rotation, targetRotation, Time.deltaTime * 2); //Rotate Character accordingly.
}
}
2.2 Apply Script
Add this script to character. This is how CharacterFaceRelativeToSurface method works.
- First it detects the surface/base using RayCast.
- Assign the normal of the surface to surfaceNormal.
- Set player’s targetRotation using Quaternion.LookRotation()
- Rotate player from current rotation to the target rotation using Quaternion.Lerp()
I hope you found this blog helpful while working Character Rotation and Movement According to Surface In UNITY Let me know if you have any questions or concerns regarding character rotation, character movement and surface in unity, please put a comment here and we will get back to you ASAP.
Got an Idea of Game Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Game Development Company in India.
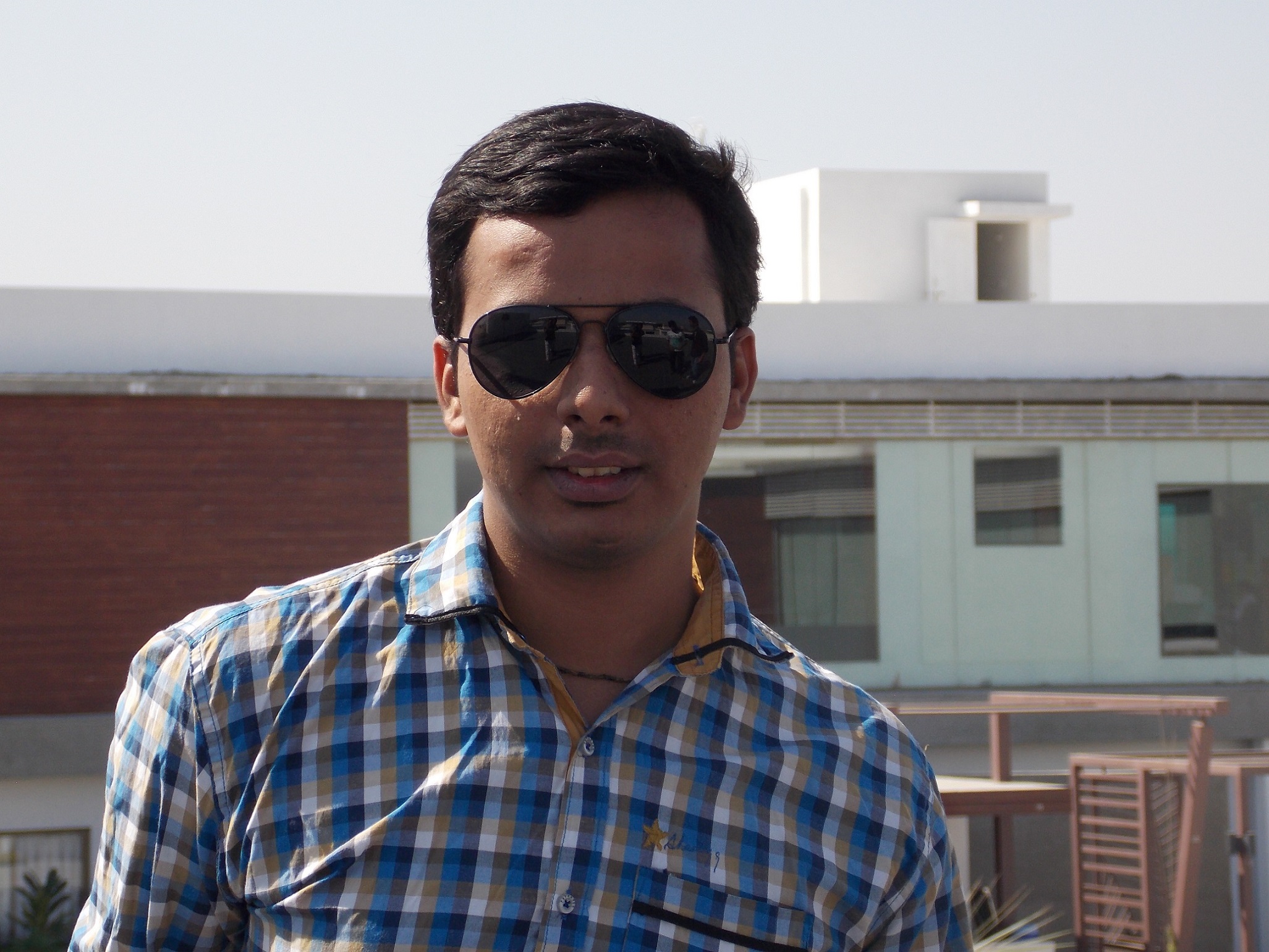
I am professional Android App & Game developer. I love to develop unique and creative Games & Apps. I am very passionate about my work.