
Be patient..... we are fetching your source code.
Objective
The main objective of this blog post is to explain how to give lighting effect on 2D sprite in unity.
The intention of this blog is to introduce beginners of unity to Light game object and explain how to use lights on 2D sprite. Different types of light available in unity and they have various properties.
Step 1 Light
Lights are illuminate scenes and sometimes to catch the user attention to certain objects. There are four different light components are available in unity.
1.1 Directional Light
Used to create a light source that is extremely far away but illuminate everything in the scene
e.g. The Sun
1.2 Point Light
Used to create a light source that shines equally from all direction
e.g. A Candle or A Light Bulb
1.3 Spot Light
Used to create a light source that shines in one direction and lights objects within a cone
e.g. Stage Spotlights or The Headlights of car
1.4 Area Light
It’s only available for lightmap baking and shine in all directions to one side of a rectangular section of a plane. The Light component has the following properties:
- Type: Determines the type of the Light component.
- Spot: A Spotlight, which simulates a cone shaped light pointing in the local Z-axis.
- Directional: A Directional light, which illuminates all objects in the scene from a single direction. Position is irrelevant.
- Point: A Point light shines light in every direction equally. The light affects only objects within Range distance.
- Area: A light that shines in all directions to one side of a rectangular area of a plane. The rectangle is defined by the width and height properties. Area lights are only available during lightmap baking and have no effect on objects at runtime.
- Range: This property is only available if the Type is either Spot or Point. This value determines the distance (in world units) at which the light will affect an object. The light will not illuminate objects beyond this distance from the light.
- Spot Angle: This property is only available if the Type is set to Spot. This value determines the cone angle (measured in degrees) of the spotlight cone.
- Color: The color of the light emitted.
- Intensity: The brightness of the light.
- Cookie: The alpha channel of the Cookie texture is used to determine the brightness of the light. If the Light is of Type Spot or Directional, then this must be a 2D Texture. If the Light is of Type Point, then this must be a Cubemap.
- Cookie Size: This property is only available if the Type is set to Directional. This property allows you to scale the cookie texture that is applied to the Directional light.
- Shadow Type (Pro only): Shadows are only available with the Pro license. Shadow Type has three values:
- No Shadows: This light does not produce shadows.
- Hard Shadows: The light produces hard shadows. This is the preferred method for generating shadows because it is cheaper to compute.
- Soft Shadows: This method produces shadows with blurred edges. This method is slower than Hard Shadows, but it produces a better result.
- Strength: The darkness of the shadow will be multiplied by this value to reduce the intensity of shadows produced by this light.
- Bias: If the light produces an effect called Shadow Acne then you can adjust the Bias parameter to resolve the artifacts. However, setting the Bias parameter too high may cause Peter Panning.
- Resolution: This property determines the size of the shadow map texture used by this light. Very High Resolution will produce better shadows at the expensive of speed and memory.
- Draw Halo: If selected a spherical halo of light will appear around the object. This will have a radius distance equal to the range property.
- Flare: This is an optional property referring to the Flare that will be rendered at the lights location.
- Render Mode: This is used to select whether the light is rendered as a vertex light, pixel light or automatically determined.
- Culling Mask: This is used to include or exclude layers of objects to be affected by the light.
- Light Mapping: This property determines how this light contributes to the Lightmapping process. It has three values RealtimeOnly, Auto and BakedOnly.
Step 2 Create Light
- To create lighting effect on 2DSprite, first create new material named LightMaterial and set its shader property to Sprites/ Diffuse Shader.
- Now assign this material to all or some sprites of scene on which you want to apply lighting effect.
- Add one of Light object in scene ( GameObject >> CreateOther >> choose appropriate light object ).
- Set the properties of light component as per requirement.
Step 3 Example
The following simple c# code will move the light wherever mouse dragged.
Note
- Add this script on light object.
- Z index of Light object must be higher than sprite on which lighting effect is applied.
- By default when you create a new scene, you will not have any lights in your scene. Without lights, all sprites in the scene, which have LightMaterial, will have a dark-gray color. This dark-gray color is the default color of the Ambient Light property defined in the Render Settings dialog (select Edit -> Render Settings from the main menu). Set it to black to get the lighting effect in dark black background.
3.1 Video: Lighting Script
using UnityEngine;
using System.Collections;
public class Lighting : MonoBehaviour
{
private bool isMousePressed; // Used to determine whether mouse button is pressed or not
void Start ()
{
isMousePressed = false;
}
void Update ()
{
if(Input.GetMouseButtonDown(0))
isMousePressed = true;
else if(Input.GetMouseButtonUp(0))
isMousePressed = false;
// Following lines move the light at mouse position when mouse is pressed
if(isMousePressed)
{
Vector3 lightPos = Camera.main.ScreenToWorldPoint(Input.mousePosition);
lightPos.z = -1;
transform.position = lightPos;
}
}
}
If you have got any query related to Lighting Effect on 2D sprite in unity please comment them below we will try to solve them out.
Got an Idea of Game Development? What are you still waiting for? Contact us now and see the Idea live soon. Our company has been named as one of the best Game Development Company in India.
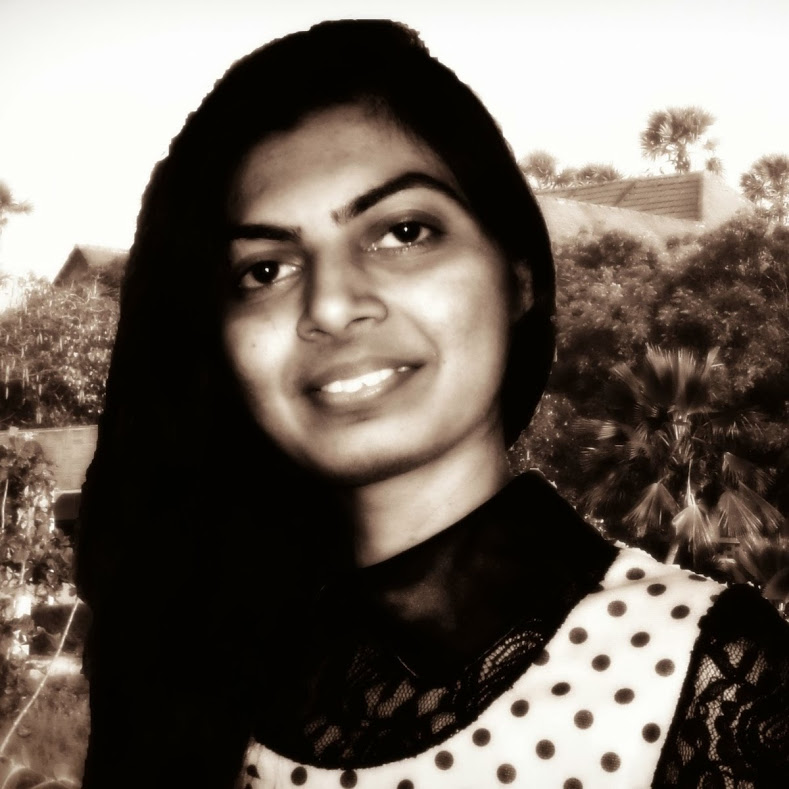
I am professional Game Developer, developing games in cocos2d(for iOS) and unity(for all platforms). Games are my passion and I aim to create addictive, high quality games. I have been working in this field since 1 year.
iOS - Image Processing using GPUImage Framework
iOS - Create Gradient View